Unlocking the Magic of Data Transformation: Exploring the Power of Angular Pipes
Welcome to a journey through the enchanting world of data transformation in Angular applications. In this blog post, we will dive deep into the concept of Angular pipes, a powerful feature that enables developers to write cleaner, more readable code while performing complex data transformations right within the template. Whether you are a seasoned Angular developer or just starting, understanding how to leverage pipes will significantly enhance your application's functionality and user experience. Let's explore the magic that Angular pipes bring to the table, from the basics to more advanced techniques.
What are Angular Pipes?
At their core, Angular pipes are simple functions used in template expressions to accept an input value and return a transformed value. Think of them as filters or processors that can be applied to data right within the Angular template, without the need for additional JavaScript or TypeScript code in your component files. Angular comes with a robust set of built-in pipes for common tasks such as formatting dates, numbers, and strings, as well as more complex operations like filtering and sorting lists.
Using Built-in Angular Pipes
Angular includes several out-of-the-box pipes that you can use immediately in your projects. Let's explore some of the most commonly used built-in pipes:
- DatePipe: Transforms a date value into a string format according to locale rules.
- UpperCasePipe and LowerCasePipe: Converts a string to uppercase or lowercase.
- DecimalPipe: Transforms a number into a string with a decimal point, formatted according to locale rules.
- CurrencyPipe: Transforms a number into a currency string, formatted according to locale rules.
Using these pipes in your templates can drastically reduce the complexity of your components, making your code cleaner and more maintainable.
Creating Custom Angular Pipes
While Angular's built-in pipes cover a wide range of common use cases, you might encounter situations where you need to perform custom data transformations. Fortunately, Angular makes it easy to create your own custom pipes. Here's a basic example of how to create a custom pipe that reverses the characters of a string:
@Pipe({name: 'reverseStr'})
export class ReverseStrPipe implements PipeTransform {
transform(value: string): string {
return value.split('').reverse().join('');
}
}
You can then use this custom pipe in your templates just like any built-in pipe:
{{ 'hello' | reverseStr }}
Practical Tips for Using Angular Pipes
While Angular pipes are incredibly powerful, here are some practical tips to keep in mind to make the most out of them:
- Avoid complex operations in pipes: Pipes are executed on every change detection cycle, so heavy computations can lead to performance issues.
- Use pure pipes when possible: Pure pipes only execute when they detect a pure change to the input value (like primitives or object reference changes), which can improve performance.
- Combine pipes: You can chain multiple pipes together to perform complex transformations in a clean and readable way.
Summary
In this blog post, we've explored the magical world of Angular pipes, from their basic usage to the creation of custom pipes and practical tips for optimizing performance. Angular pipes are a powerful feature that can greatly simplify your code and enhance your application's functionality. Whether you're using built-in pipes for common data transformations or creating your own custom pipes for unique requirements, Angular pipes offer a flexible and efficient way to transform data right within your templates.
As you continue to build and enhance your Angular applications, I encourage you to experiment with pipes and discover the many ways they can improve your development workflow and user experience. Remember, the true magic of Angular pipes lies in their simplicity and power—unlock it, and you'll open up a world of possibilities for your Angular projects.
Happy coding!
Recent Posts
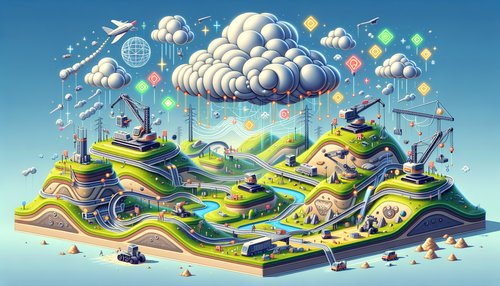
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
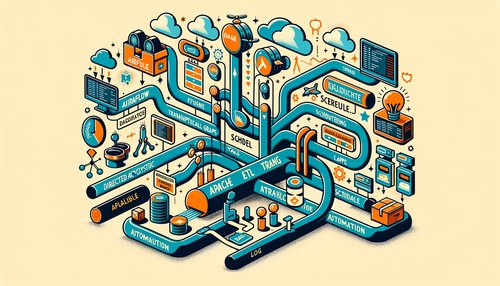
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow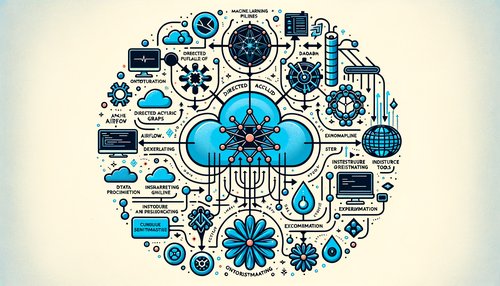
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow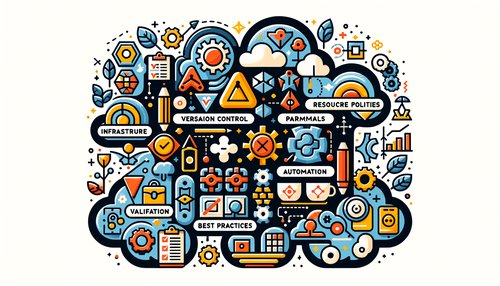
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
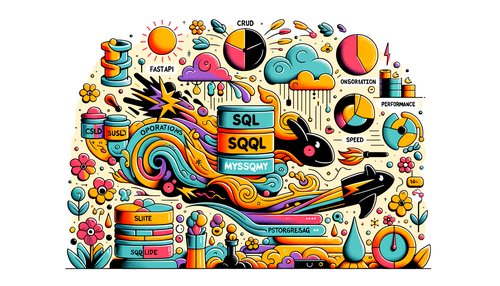