Unlocking the Mysteries of Categorical Data: A Beginner’s Guide to Mastering Pandas
Welcome to the enthralling world of data analysis with Pandas! If you've ever felt overwhelmed by the sheer volume of data available in today’s digital age or puzzled over how to organize, analyze, and derive meaningful insights from categorical data, you're in the right place. This guide is meticulously crafted for beginners eager to navigate the complexities of categorical data using Pandas, a cornerstone library in Python for data manipulation and analysis. Let’s embark on this journey to demystify categorical data, making it not just comprehensible but also manageable and insightful for your projects.
Understanding Categorical Data
Categorical data, often referred to as qualitative data, represents types or categories. Unlike numerical data that expresses quantities, categorical data reflects attributes or qualities that can be sorted into groups or categories. Examples include colors (red, green, blue), sizes (small, medium, large), or ratings (poor, average, good). Understanding how to handle this type of data is crucial for any aspiring data analyst, and Pandas provides the tools you need to get started.
Getting Started with Pandas
Before diving into the nitty-gritty of categorical data, it's essential to set up your environment. If you haven’t already, install Pandas in your Python environment using pip:
pip install pandas
Once installed, you can import Pandas and start utilizing its powerful features. A good starting point is to create a DataFrame, which is essentially a table, or a two-dimensional labeled data structure with columns of potentially different types.
import pandas as pd
data = {'Category': ['A', 'B', 'C', 'A', 'B', 'C'],
'Value': [1, 2, 3, 4, 5, 6]}
df = pd.DataFrame(data)
This simple example illustrates how to create a DataFrame with categorical data. Here, 'Category' is a categorical variable with three categories (A, B, C).
Manipulating Categorical Data
One of Pandas' strengths is its ability to handle and manipulate categorical data efficiently. You can convert a column in your DataFrame to a categorical type using the astype
method:
df['Category'] = df['Category'].astype('category')
This conversion is not just for show; it optimizes memory usage and speeds up operations like sorting and grouping. For large datasets, this can lead to significant performance improvements.
Sorting and Grouping with Categorical Data
Sorting and grouping are fundamental operations when working with data. Pandas makes these tasks intuitive:
# Sorting by category
df.sort_values(by='Category')
# Grouping by category
df.groupby('Category').sum()
These operations allow you to quickly organize your data and perform computations like summing values within each category. Such insights can be invaluable for data analysis projects.
Visualizing Categorical Data
Visualization is a powerful tool for understanding and presenting categorical data. While Pandas itself does not provide direct visualization capabilities, it integrates seamlessly with Matplotlib, a comprehensive library for creating static, animated, and interactive visualizations in Python. Here’s how you can create a simple bar chart:
import matplotlib.pyplot as plt
df.groupby('Category').sum().plot(kind='bar')
plt.show()
This simple example aggregates the values by category and displays them as a bar chart, making it easier to compare the categories visually.
Advanced Tips
As you become more comfortable with Pandas, explore more advanced features such as:
- Using the
CategoricalDtype
to define a specific order for your categories, rather than the default alphabetical order. - Handling missing data within categorical columns, using methods like
fillna
ordropna
. - Utilizing category codes to represent each category with an integer code, which can be useful for certain types of analysis or machine learning models.
Summary
We’ve covered the basics of handling categorical data in Pandas, from understanding what categorical data is to manipulating, sorting, grouping, and visualizing it. Pandas is an incredibly powerful tool that, when mastered, can significantly streamline your data analysis process. Remember, the journey to mastering Pandas doesn’t stop here; there’s always more to learn and explore. So, keep practicing, experimenting with new datasets, and pushing the boundaries of what you can achieve.
Embrace the power of Pandas and unlock the potential of your data. Happy analyzing!
Recent Posts
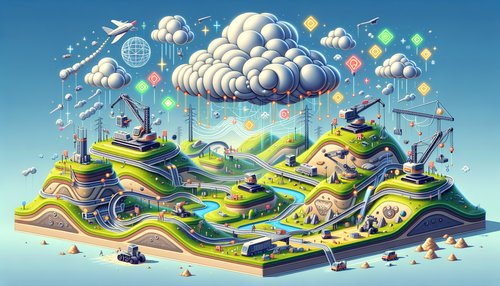
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
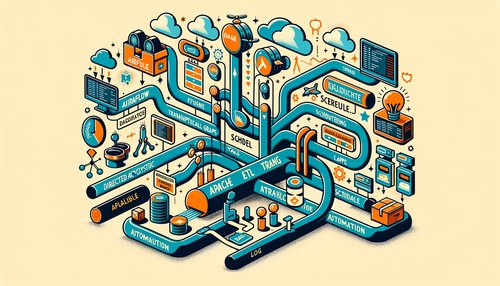
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow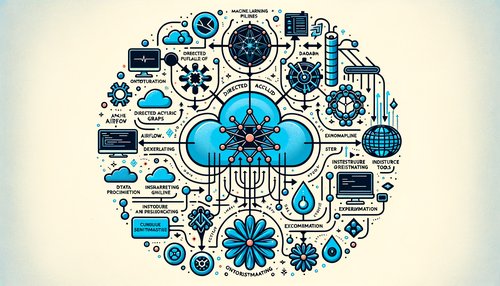
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow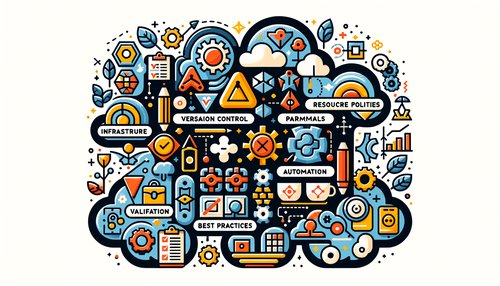
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
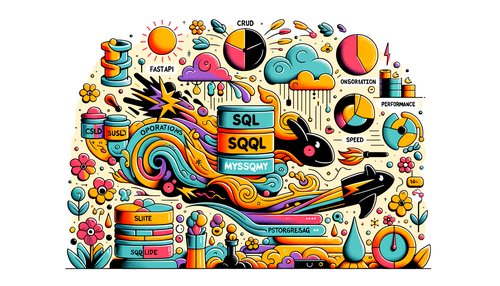