Unlocking the Power of Django: How Receivers Can Transform Your Application's Efficiency
Welcome to a deep dive into one of Django's most powerful, yet underutilized features: receivers. Django, a high-level Python web framework, simplifies the creation of complex, database-driven websites. Among its plethora of features, receivers stand out as a transformative tool for enhancing your application's efficiency. This post will explore the concept of receivers, how they work, and practical ways to implement them in your Django projects. Whether you're a seasoned developer or new to Django, understanding receivers will unlock new levels of efficiency and functionality in your applications.
Understanding Django Signals and Receivers
Before delving into receivers, it's crucial to understand the concept of signals in Django. Signals allow certain senders to notify a set of receivers when certain actions occur. It's a strategy to allow decoupled applications to get notified when certain actions are performed elsewhere in the framework. Receivers are functions connected to signals, and they react to the signal being sent. This mechanism can be incredibly useful for executing tasks like invalidating caches, sending notifications, or updating related objects in response to certain events.
Why Use Receivers?
Receivers can significantly enhance your application's efficiency and cleanliness. By using receivers, you can keep your business logic separated from your models and views, adhering to the Single Responsibility Principle. This separation makes your codebase more maintainable, testable, and scalable.
Setting Up Your First Receiver
Setting up a receiver in Django involves two main steps: defining the receiver function and then registering it with a signal. Here’s a simple example:
from django.db.models.signals import post_save
from django.dispatch import receiver
from myapp.models import MyModel
@receiver(post_save, sender=MyModel)
def my_model_changed(sender, instance, **kwargs):
print(f"{instance} has been saved!")
This receiver listens to the post_save
signal of the MyModel
class. Whenever an instance of MyModel
is saved, the my_model_changed
function gets executed.
Advanced Receiver Patterns
While the above example is straightforward, receivers can be much more powerful. Here are a few advanced patterns:
- Conditional Signals: You can use conditions within your receivers to perform actions based on certain attributes of the instance or the context of the signal.
- Signal Chaining: Receivers can emit signals themselves, allowing for complex workflows where different parts of your application respond to a series of events.
- Using Custom Signals: While Django provides a range of built-in signals, you can also create your own custom signals to represent specific events in your application.
Best Practices for Working with Receivers
While receivers are powerful, they should be used judiciously. Here are some best practices to keep in mind:
- Keep Receivers Lightweight: Receivers are called synchronously, so they can potentially slow down your request/response cycle. Ensure they execute quickly.
- Idempotency: Make sure that your receivers are idempotent. Executing the receiver multiple times should not have unintended side effects.
- Debugging: Debugging receivers can sometimes be challenging since they are decoupled from the request that triggered the signal. Logging and monitoring are essential.
Conclusion
Receivers in Django offer a robust mechanism for enhancing application efficiency, enabling clean separation of concerns, and facilitating complex workflows. By understanding and implementing receivers, you can take your Django projects to new heights of scalability and maintainability. Remember to adhere to best practices to ensure your receivers contribute positively to your application's performance. Happy coding!
As a final thought, consider diving deeper into Django's signals and receivers in your next project. Experiment with different patterns and explore how they can simplify your application's logic and improve efficiency. The power of Django lies in its flexibility and the vast ecosystem it supports. Embracing advanced features like receivers is just the beginning of unlocking its full potential.
Recent Posts
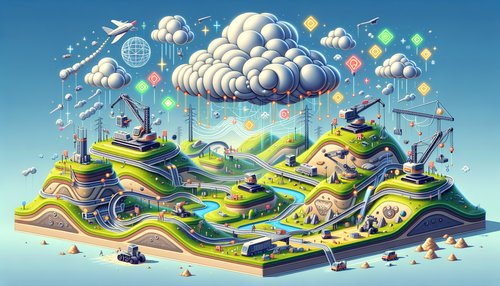
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
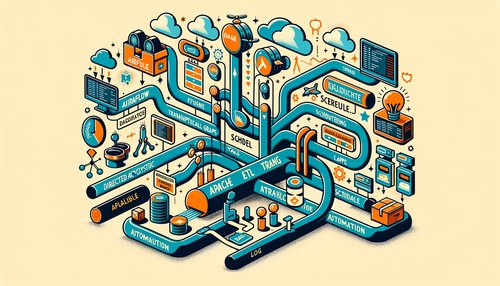
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow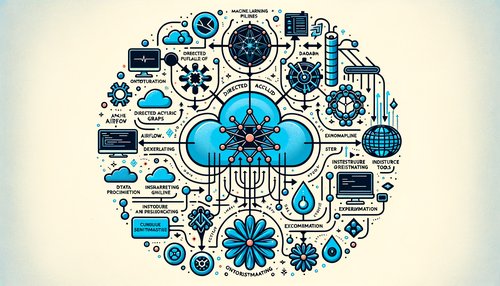
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow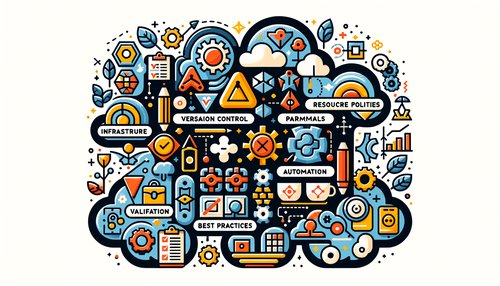
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
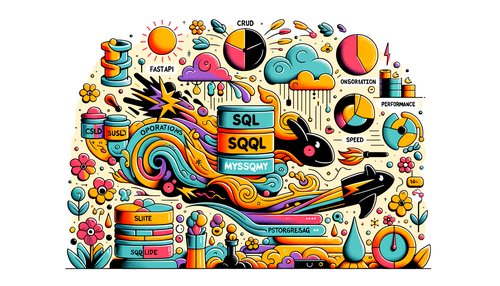