Unlocking the Power of Django Signals: A Guide to Streamlining Your App's Performance
Django Signals are powerful tools that allow developers to automate and streamline their applications. Signals allow developers to register functions to be executed when certain events occur, such as when a model is saved or when a request is received. By using signals, developers can quickly and easily create powerful and efficient applications without having to write a lot of code.
In this guide, we'll cover the basics of Django Signals, how to create and use them, and some of the powerful features they offer. We’ll also look at some real-world examples of how to use Django Signals to improve the performance of your application.
What are Django Signals?
Django Signals are a type of event-driven programming system. Signals allow developers to register functions that will be executed when certain events occur. For example, developers can register a signal to be executed when a model is saved or when a request is received. By using signals, developers can quickly and easily create powerful and efficient applications without having to write a lot of code.
How to Create and Use Django Signals
Creating and using Django Signals is simple and straightforward. To create a signal, you first need to create a function that will be executed when the signal is triggered. This function should take at least one argument, which is the sender of the signal. The sender is the object that triggered the signal, such as a model or request.
Once the function has been created, the signal can be registered. This is done by using the @receiver
decorator, which takes the signal and the function as arguments. Here's an example of a signal being registered:
@receiver(post_save, sender=MyModel)
def my_signal_handler(sender, instance, **kwargs):
# Do something with the instance
Powerful Features of Django Signals
One of the most powerful features of Django Signals is that they can be used to trigger other signals. This allows developers to chain signals together, creating complex and powerful event-driven logic. For example, a signal could be used to trigger another signal when a model is saved. This could be used to send an email notification or update another model.
Signals can also be used to trigger different functions based on the sender. This allows developers to create powerful and efficient applications without having to write a lot of code. For example, a signal could be used to trigger different functions based on the type of model that was saved.
Real-World Examples
Django Signals can be used in a variety of real-world applications. Here are a few examples of how you can use signals to streamline your application’s performance:
- Automatically updating a cache when a model is saved
- Sending an email notification when a model is updated
- Logging changes to a model when it is saved
- Automatically generating thumbnails when an image is uploaded
By using signals, developers can quickly and easily create powerful and efficient applications without having to write a lot of code.
Conclusion
Django Signals are powerful tools that allow developers to automate and streamline their applications. Signals allow developers to register functions to be executed when certain events occur, such as when a model is saved or when a request is received. By using signals, developers can quickly and easily create powerful and efficient applications without having to write a lot of code.
Recent Posts
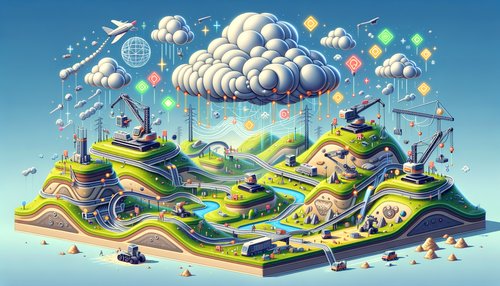
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
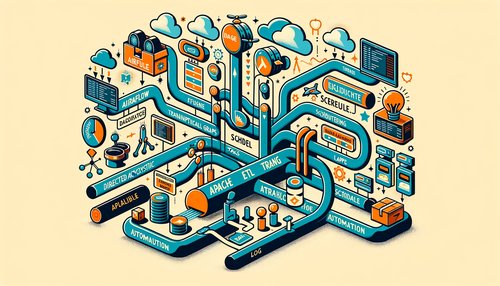
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow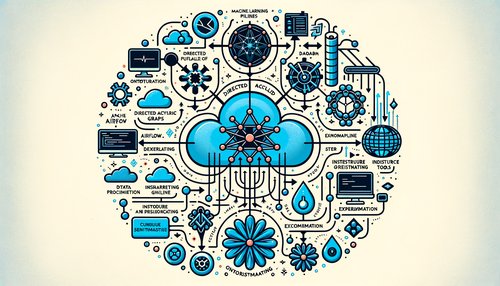
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow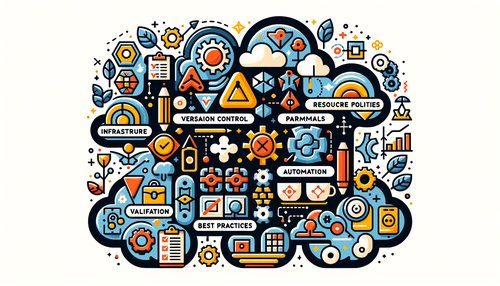
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
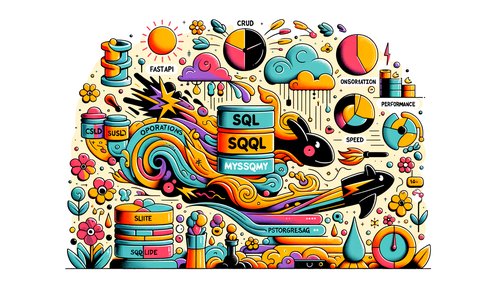