Unlocking the Power of FastAPI: A Comprehensive Guide to Handling User File Requests Like a Pro!
Welcome to a deep dive into the capabilities of FastAPI, specifically tailored for developers looking to master handling user file requests. Whether you're building a web application that requires users to upload documents, images, or any other file type, FastAPI offers a streamlined, efficient way to handle these operations. This guide will take you through everything from the basics of file uploading to advanced techniques that will make your API more robust and user-friendly. Get ready to unlock the full potential of FastAPI and elevate your web development skills!
Understanding File Uploads in FastAPI
Before we jump into the technicalities, it's crucial to understand how FastAPI handles file uploads. FastAPI uses form data to receive files uploaded by the user. This method is both efficient and straightforward, allowing for the easy integration of file uploads into your API endpoints. We'll explore how to set up your FastAPI application to accept file uploads, manage file storage, and ensure your application remains secure and scalable.
Setting Up Your Environment
First things first, ensure that your development environment is ready. You'll need Python 3.6+ installed, along with FastAPI and Uvicorn, an ASGI server that serves your application. Install FastAPI and Uvicorn using pip:
pip install fastapi uvicorn python-multipart
Note the inclusion of python-multipart
. It's essential for FastAPI to handle file uploads.
Creating a File Upload Endpoint
With your environment set up, the next step is to create an endpoint in your FastAPI application that can accept file uploads. FastAPI simplifies this process, allowing you to define file parameters in your function arguments. Here's a simple example:
from fastapi import FastAPI, File, UploadFile
from typing import List
app = FastAPI()
@app.post("/uploadfile/")
async def create_upload_file(file: UploadFile = File(...)):
return {"filename": file.filename}
This code snippet creates an endpoint that accepts a single file upload. The UploadFile
object provides several useful attributes and methods, such as filename
, which we return in this example.
Handling Multiple File Uploads
To handle multiple file uploads, you can simply modify the endpoint to accept a list of UploadFile
objects:
@app.post("/uploadfiles/")
async def create_upload_files(files: List[UploadFile] = File(...)):
return {"filenames": [file.filename for file in files]}
This adjustment allows users to upload several files at once, with the endpoint returning a list of the file names.
Advanced File Handling Techniques
Once you've mastered the basics of file uploading in FastAPI, you can explore more advanced techniques to improve your application's functionality and user experience.
File Validation
Validating the files uploaded by users is crucial for security and ensuring that only the correct file types are processed. You can validate files based on their MIME type or extensions, for example, to allow only images:
from fastapi import HTTPException
@app.post("/uploadimage/")
async def create_upload_image(file: UploadFile = File(...)):
if not file.content_type.startswith('image/'):
raise HTTPException(status_code=400, detail="Invalid file type")
return {"filename": file.filename}
This code snippet checks the MIME type of the uploaded file and raises an HTTP exception if the file is not an image.
Storing Files
Handling the storage of uploaded files is another critical aspect. You can save files to a local directory or a cloud storage service. Here's how to save a file to a local directory:
import shutil
@app.post("/savefile/")
async def save_upload_file(file: UploadFile = File(...)):
with open(f"uploads/{file.filename}", "wb") as buffer:
shutil.copyfileobj(file.file, buffer)
return {"filename": file.filename}
This method uses shutil.copyfileobj
to write the uploaded file to a specified directory.
Conclusion
FastAPI provides a powerful, efficient way to handle user file uploads in your web applications. By following this guide, you've learned how to set up your FastAPI environment for file uploads, create endpoints for single and multiple file uploads, and implement advanced techniques like file validation and storage. As you continue to explore FastAPI's features, remember that the key to successful file handling is not just about accepting files but ensuring they are processed securely and efficiently. Happy coding!
Now that you're equipped with the knowledge to handle file uploads like a pro, why not take your FastAPI skills to the next level by exploring more of its features and best practices? Your journey into the world of FastAPI is just beginning, and the possibilities are endless.
Recent Posts
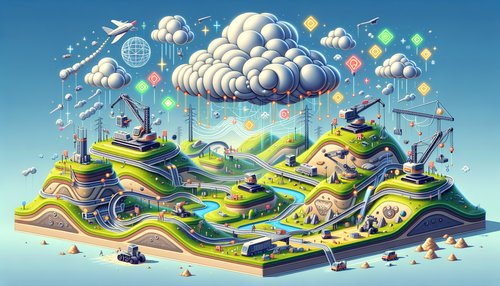
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
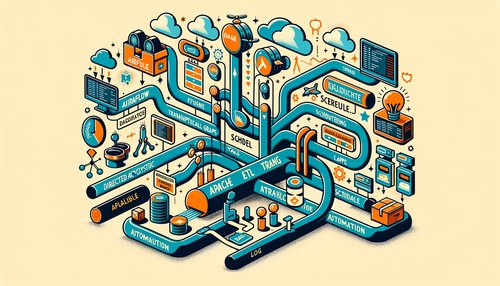
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow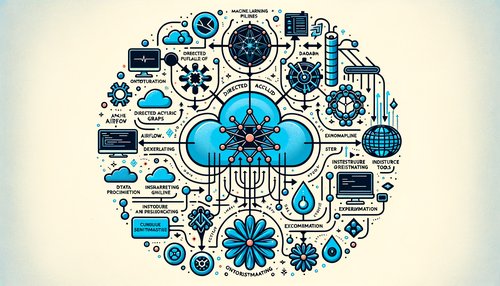
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow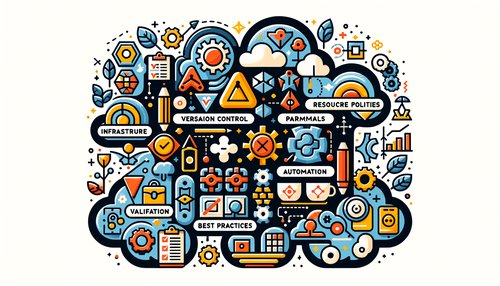
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
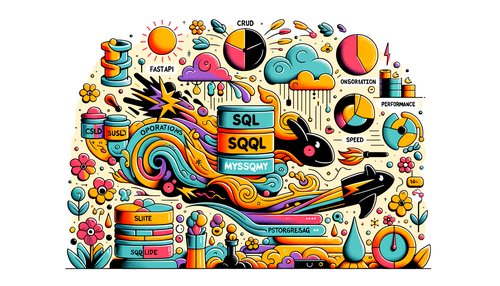