Unlocking the Power of FastAPI: A Comprehensive Guide to User Metadata and Documentation URLs
FastAPI has revolutionized the way developers approach building web applications. With its ability to handle API requests at lightning speed and its intuitive design, it has become a favorite among many in the software development community. This comprehensive guide will delve into two powerful features of FastAPI: User Metadata and Documentation URLs.
In this guide, we'll explore:
- An introduction to FastAPI and its benefits
- Using Metadata to enhance your API documentation
- Customizing Documentation URLs for better user experience
- Practical tips and examples to get you started
Introduction to FastAPI and Its Benefits
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.6+ based on standard Python type hints. It touts some incredible features:
- Fast to code: Increase the speed to develop features by about 200% to 300%.
- Fewer bugs: Reduce about 40% of human (developer) induced errors.
- Intuitive: Great editor support. Completion everywhere. Less time debugging.
- Easy to use: Designed to be easy to use and learn. Less time reading docs.
- Production-ready: Robust, mature, and versatile, production-ready framework.
Incorporating FastAPI into your workflow can transform the way you build and maintain APIs. Let's dig deeper into two of its most effective features: User Metadata and Documentation URLs.
Using Metadata to Enhance Your API Documentation
Metadata in FastAPI is powerful for improving your API's documentation, making it easier for users to understand and interact with your endpoints.
Adding Metadata to Your Routes
FastAPI allows you to add metadata to your endpoints with the use of docstrings, which are automatically integrated into the interactive API documentation (Swagger UI). Here is a simple example:
from fastapi import FastAPI
app = FastAPI()
@app.get("/items/{item_id}", tags=["items"], summary="Get an item by ID", description="Retrieve an item from the database by its unique ID.")
def read_item(item_id: int):
return {"item_id": item_id}
This metadata enhances the user experience by clearly defining what each endpoint does, its tags, and how it should be used.
Customizing Documentation URLs for Better User Experience
One of the impressive features of FastAPI is its automatic API documentation. By default, FastAPI provides automatic interactive API documentation using Swagger UI and ReDoc at specific URLs. However, you can customize these URLs to better fit your application.
Changing Documentation URLs
You can change the default documentation URLs easily:
from fastapi import FastAPI
app = FastAPI(docs_url="/api/docs", redoc_url="/api/redoc", openapi_url="/api/openapi.json")
In this example, the Swagger UI documentation will be available at /api/docs
, ReDoc documentation at /api/redoc
, and the OpenAPI schema at /api/openapi.json
. Customizing these URLs can help you integrate the documentation seamlessly into your existing application structure.
Practical Tips and Examples
Here are some practical tips and examples to make full use of FastAPI's metadata and documentation features:
Grouping Endpoints with Tags
Using tags can help group related endpoints together. This makes your API documentation more organized:
@app.get("/users/", tags=["users"])
def get_users():
return [{"username": "John Doe"}]
@app.get("/items/", tags=["items"])
def get_items():
return [{"item_name": "Item 1"}]
In the interactive documentation, endpoints with the same tags will be grouped, making it easier for users to navigate.
Adding Detailed Descriptions
Including detailed descriptions and summaries in your endpoint definitions enhances clarity:
@app.post("/users/", tags=["users"], summary="Create a new user", description="This endpoint creates a new user and stores it in the database.")
def create_user(user: User):
return {"username": user.username}
The detailed descriptions will show up in the interactive documentation, providing users with all the information they need to use your API effectively.
Conclusion
FastAPI's robust features for user metadata and customizable documentation URLs significantly enhance the development and user experience. By leveraging these capabilities, you can create APIs that are both powerful and user-friendly.
Start experimenting with FastAPI's metadata options and documentation customization today, and see how they can transform your API development process. Happy coding!
Recent Posts
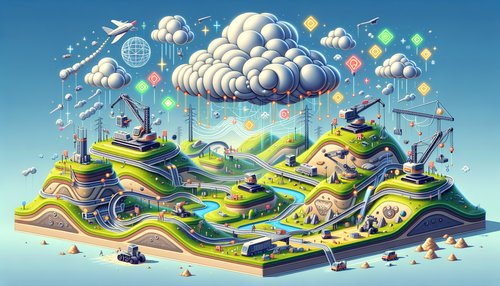
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
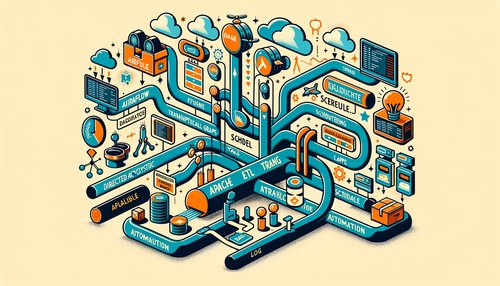
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow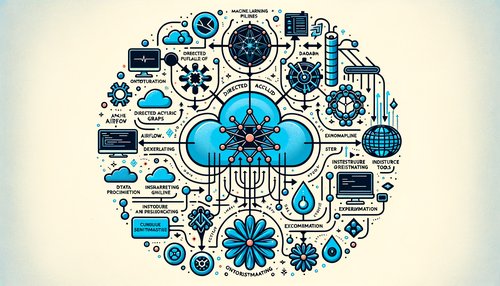
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow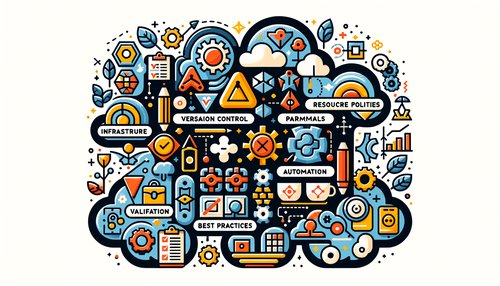
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
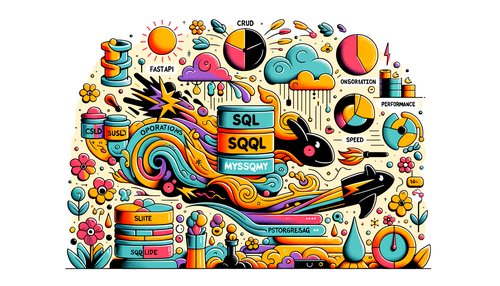