Unlocking the Power of FastAPI: A Comprehensive Guide to Mastering User Guide Dependencies and Global Dependencies
Welcome to the world of FastAPI, a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. This comprehensive guide is designed to unlock the full potential of FastAPI, focusing on mastering user guide dependencies and global dependencies. Whether you're a beginner aiming to get a solid start or an experienced developer looking to deepen your understanding, this guide will provide valuable insights, practical tips, and examples to enhance your FastAPI projects.
Understanding Dependencies in FastAPI
Before diving into the specifics, it's crucial to grasp what dependencies in FastAPI are and how they function. In the context of FastAPI, a dependency is a standalone function that can be reused across multiple path operations. Dependencies are used for a wide range of purposes, including database connections, authentication, and more. They help in creating cleaner, more modular, and more scalable codebases.
Types of Dependencies
FastAPI supports several types of dependencies, but we will focus on two main categories:
- User Guide Dependencies: These are custom dependencies that you define to solve specific problems in your application, like fetching user information from a database or verifying permissions.
- Global Dependencies: These apply to your entire application or to specific groups of routes. They are ideal for functionality that needs to be applied universally, such as CORS (Cross-Origin Resource Sharing) policies, database session management, or middleware.
Mastering User Guide Dependencies
User guide dependencies in FastAPI allow for incredibly flexible and powerful configurations. Here are some tips and insights to master them:
Creating and Using Custom Dependencies
To create a custom dependency, you simply define a function and use it in your route operations. Here’s a basic example:
from fastapi import Depends, FastAPI
def common_parameters(q: str = None, skip: int = 0, limit: int = 100):
return {"q": q, "skip": skip, "limit": limit}
app = FastAPI()
@app.get("/items/")
async def read_items(commons: dict = Depends(common_parameters)):
return commons
This example shows a custom dependency that extracts common query parameters. It's then used in a route to get and return these parameters.
Advanced Dependency Techniques
FastAPI dependencies can also utilize advanced Python features, such as classes, async functions, and more. For instance, using classes as dependencies allows for the initialization of shared resources or services that your application can reuse. This can significantly enhance efficiency and performance.
Utilizing Global Dependencies
Global dependencies provide a way to apply certain functionalities across your FastAPI application. Implementing global dependencies effectively can greatly improve your app's architecture and maintainability.
Applying Global Dependencies
To apply a global dependency in FastAPI, you use the dependencies
argument of the FastAPI
class. This is particularly useful for middlewares, error handlers, and other app-wide functionalities. Here’s an example of how to apply a simple global dependency that ensures all responses are in JSON format:
from fastapi import FastAPI, Depends
def ensure_json_response():
# Your implementation here
pass
app = FastAPI(dependencies=[Depends(ensure_json_response)])
Best Practices for Global Dependencies
While global dependencies are powerful, they should be used judiciously. Always consider the impact on the entire application and whether a dependency truly needs to be global. In many cases, applying dependencies at the router level might be more appropriate and flexible.
Conclusion
FastAPI's dependency system offers a robust and flexible way to build and manage your web applications. By mastering user guide dependencies and global dependencies, you can create more modular, scalable, and maintainable codebases. Remember, the key to effectively using dependencies is understanding their scope and impact. Use them to abstract common functionality, manage resources, and apply consistent policies across your application. With practice and experimentation, you'll unlock the full potential of FastAPI's dependency system.
As a final thought, consider contributing to the FastAPI community. Whether it's by sharing your custom dependencies, writing tutorials, or providing feedback on the framework, your contributions can help others and drive the framework forward. Happy coding!
Recent Posts
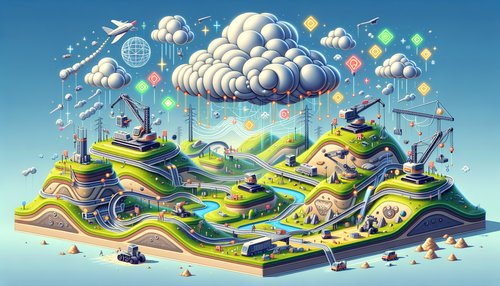
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
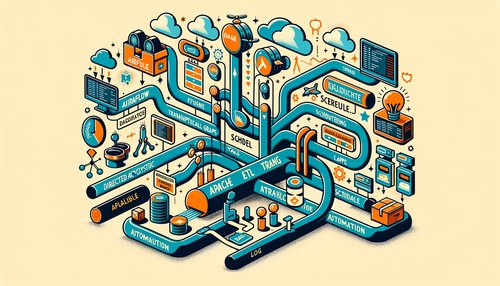
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow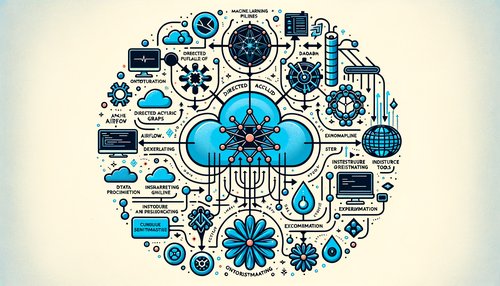
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow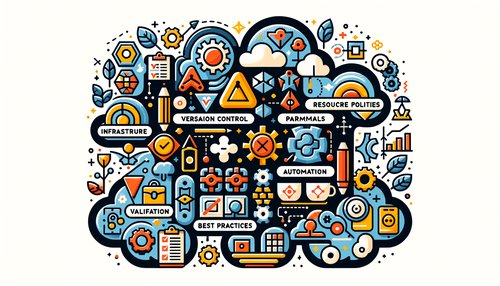
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
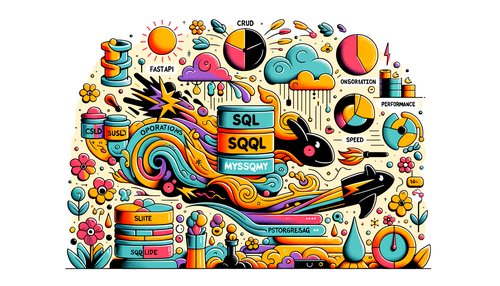