Unlocking the Power of FastAPI: A Comprehensive User Guide to Mastering Response Status Codes
FastAPI has rapidly emerged as a game-changer in the world of web development, thanks to its high performance and ease of use for creating APIs. At the heart of every API interaction lie the response status codes, a critical aspect that can make or break the user experience. Understanding and mastering these codes is pivotal for developers looking to fine-tune their applications and provide seamless services. This guide aims to unlock the power of FastAPI by diving deep into the world of response status codes, offering insights, practical tips, and examples to help you become a FastAPI maestro.
Understanding Response Status Codes
Before delving into the specifics of FastAPI, it's crucial to grasp what response status codes are and why they matter. In essence, these codes are standard HTTP responses sent from the server to the client, indicating the status of the request. They range from successful operations (200 series), client errors (400 series), to server errors (500 series), each providing a snapshot of what happened with the request. Knowing these codes is the first step towards debugging issues and enhancing your API's performance.
FastAPI and Response Status Codes
FastAPI provides a robust framework for handling response status codes effectively. It allows developers to specify the status code for each endpoint, ensuring that clients receive accurate feedback regarding their requests. This level of control is invaluable for creating intuitive and reliable APIs.
Example: Setting a status code in FastAPI is straightforward:
from fastapi import FastAPI, status
app = FastAPI()
@app.post("/items/", status_code=status.HTTP_201_CREATED)
async def create_item(name: str):
return {"name": name}
This snippet demonstrates how to specify that a successful POST request to the "/items/" endpoint should return a 201 (Created) status code, signaling to the client that the operation was successful.
Best Practices for Using Response Status Codes in FastAPI
While FastAPI makes it easy to work with response status codes, adhering to best practices can significantly enhance your API's usability and reliability. Here are some tips:
- Be Consistent: Use standard HTTP status codes consistently across your API. This helps clients understand and predict your API's behavior, reducing confusion and potential errors.
- Use Appropriate Codes: Always return the most appropriate status code for the situation. For example, use 404 (Not Found) when a resource doesn't exist, not just a generic 400 (Bad Request).
- Document Your Codes: Clearly document the possible status codes for each endpoint in your API documentation. This transparency is crucial for clients integrating with your API.
Advanced Techniques
Once you're comfortable with the basics, you can explore advanced techniques for further optimizing your use of response status codes in FastAPI:
- Custom Response Classes: FastAPI allows you to create custom response classes, enabling you to add headers, cookies, or modify the response body before sending it to the client.
- Error Handling: Utilize FastAPI's exception handlers to catch exceptions and return appropriate status codes, along with error messages that can help clients debug issues.
Conclusion
Mastering response status codes in FastAPI is essential for developing robust, user-friendly APIs. By understanding the basics, adhering to best practices, and leveraging advanced techniques, you can significantly enhance the quality and reliability of your API services. Remember, the goal is not just to handle requests and responses but to do so in a way that is intuitive, informative, and helpful to the client. Embrace the power of FastAPI and unlock the full potential of your web applications with mastery over response status codes.
As you continue to develop and refine your FastAPI applications, keep these insights and tips in mind to ensure that your APIs are not just functional, but truly exceptional. Happy coding!
Recent Posts
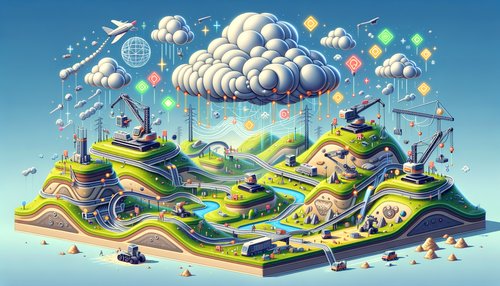
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
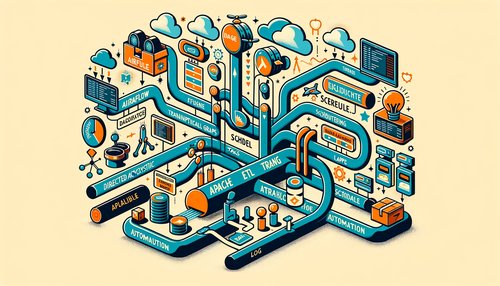
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow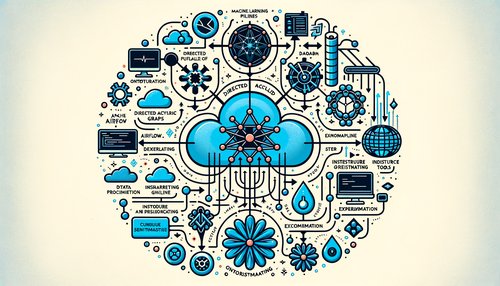
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow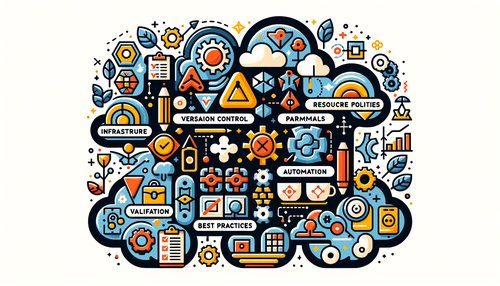
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
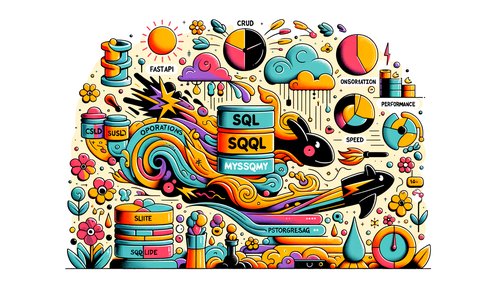