Unlocking the Power of FastAPI: A Deep Dive into Crafting Seamless User Experiences with Custom Response Models
Welcome to a journey into the world of FastAPI, a modern, fast (high-performance), web framework for building APIs with Python 3.7+ that's based on standard Python type hints. The core of FastAPI offers a lot of functionalities that help developers create APIs quickly and with minimal code. However, the true power of FastAPI shines when you start leveraging its capabilities to enhance user experience through custom response models. This blog post aims to explore the depths of FastAPI's features and how you can use them to craft seamless user experiences.
Why Focus on User Experience?
Before diving into the technicalities, it's crucial to understand why user experience (UX) holds such significance in API development. In a digital world where users have countless options at their fingertips, delivering an exceptional UX becomes paramount. A well-designed API not only ensures efficient data retrieval and manipulation but also enhances the overall interaction your users have with your application or service. By focusing on UX, you're prioritizing your end-users' needs and setting up your API for wider adoption and success.
FastAPI: A Quick Overview
FastAPI is an asynchronous framework that's built on Starlette for the web parts and Pydantic for the data parts. It's designed to be easy to use, while also enabling new, high-performance possibilities. One of FastAPI's standout features is its automatic API documentation, but what truly sets it apart is its support for asynchronous request handling and its dependency injection system, which allows for cleaner, more modular code, and its robust security features.
Custom Response Models: Enhancing UX
One of the keys to improving UX with FastAPI is through the use of custom response models. Response models allow you to shape the data you send to your clients. By carefully designing your response models, you can ensure that your API provides data in the most useful and intuitive format possible. This includes omitting unnecessary information, renaming fields for clarity, and structuring the response to match the needs of your client applications.
Defining Custom Response Models
To define a custom response model in FastAPI, you typically use Pydantic models. These models allow you to describe the types and structure of the data your API will return. You can then use these models in your route decorators to tell FastAPI to transform the output data according to the model. Here's a simple example:
from fastapi import FastAPI from pydantic import BaseModel class UserResponse(BaseModel): id: int username: str email: str app = FastAPI() @app.get("/user/{user_id}", response_model=UserResponse) async def get_user(user_id: int): # Fetch user from the database user = get_user_from_db(user_id) return user
This approach not only ensures that your clients receive the data in the expected format but also helps with data validation and documentation.
Advanced Techniques with Response Models
FastAPI provides several advanced features for working with response models. For instance, you can use response_model_exclude_unset to exclude default values from the response, or use response_model_include and response_model_exclude to fine-tune the fields that are returned. These features can significantly reduce the amount of data sent over the network and tailor the response to the specific needs of each client.
Practical Tips for Crafting Seamless UX
- Keep It Intuitive: Design your API endpoints and response models with the end-user in mind. The structure should be intuitive and easy to understand.
- Be Consistent: Ensure consistency in how data is returned across different endpoints. This reduces the learning curve for developers using your API.
- Document Thoroughly: Make full use of FastAPI's automatic documentation features. Well-documented APIs are easier to use and integrate with.
- Embrace Async: Take advantage of FastAPI's asynchronous capabilities to improve performance, especially for I/O-bound operations.
Conclusion
FastAPI provides a powerful toolkit for building APIs that not only perform well but also offer a superior user experience. By focusing on custom response models and adhering to best practices in API design, you can ensure that your users enjoy a seamless, intuitive interaction with your service. Remember, the goal is not just to expose data, but to do so in a way that is most beneficial to your users. As you continue to explore FastAPI's capabilities, keep pushing the boundaries of what you can achieve and always prioritize the needs of your end-users.
In the world of API development, the user experience is king. By harnessing the power of FastAPI and its features for crafting custom response models, you're well on your way to creating APIs that not only meet but exceed user expectations. So, take these insights, apply them to your projects, and watch as your APIs transform into gateways of seamless, efficient, and enjoyable interactions.
Recent Posts
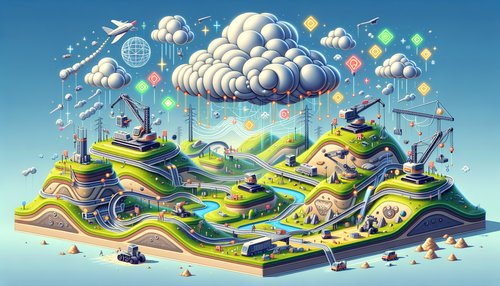
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
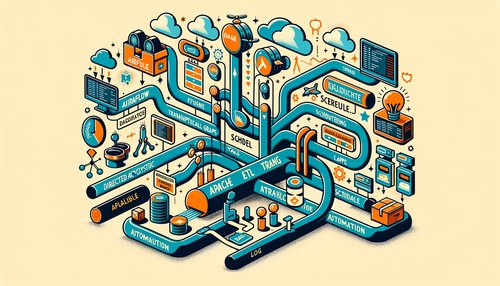
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow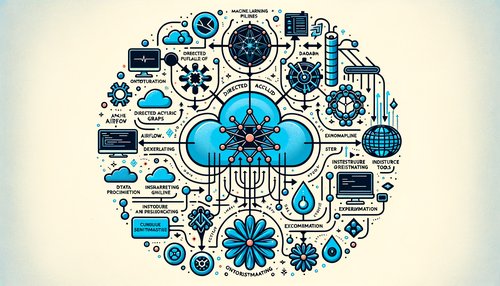
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow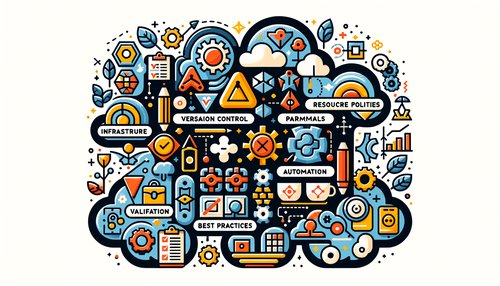
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
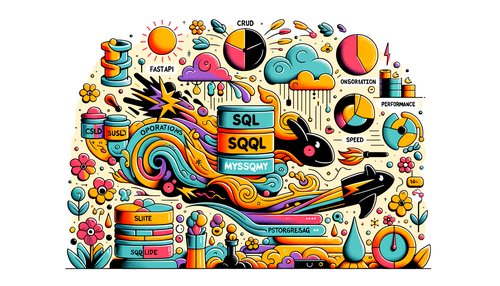