Unlocking the Power of FastAPI: How Custom Middleware Can Elevate Your Web Applications
When it comes to building modern, high-performance web applications, the choice of framework can significantly affect both development speed and the final product's efficiency. FastAPI, a relatively new entrant in the world of Python frameworks, has quickly gained popularity for its speed, ease of use, and robust feature set. One of FastAPI's most powerful, yet underutilized, features is its support for custom middleware. In this blog post, we'll dive into how leveraging custom middleware can transform your FastAPI applications, making them more efficient, secure, and scalable.
Understanding Middleware in FastAPI
Before we explore the depths of custom middleware, let's first understand what middleware is. In the context of FastAPI, middleware is a function that runs before and after each request. It's a layer that sits between the client and the server, allowing you to process requests and responses globally. This could include tasks like processing requests before they reach your endpoint logic, or modifying responses before they are sent to the client.
Why Custom Middleware?
Custom middleware can serve a multitude of purposes in your FastAPI applications. From security enhancements like CORS headers management and rate limiting to response transformation and request logging, the possibilities are vast. Implementing custom middleware can help you keep your endpoint logic clean and focused on business requirements, while cross-cutting concerns are handled separately, leading to more maintainable and scalable codebases.
Creating Custom Middleware in FastAPI
Implementing custom middleware in FastAPI is straightforward. You define a function that accepts a request and a call next parameter, which allows you to pass the request through to the next middleware or endpoint. Within this function, you can manipulate the request before it reaches the endpoint, and then alter the response before it's sent back to the client. Here's a simple example:
from fastapi import FastAPI, Request from starlette.responses import Response app = FastAPI() @app.middleware("http") async def add_custom_header(request: Request, call_next): response = await call_next(request) response.headers['X-Custom-Header'] = 'Value' return response
This middleware adds a custom header to all responses from your application. The beauty of FastAPI's middleware system is its simplicity and flexibility, allowing you to easily add such cross-cutting functionality.
Practical Uses of Custom Middleware
Now that we've covered how to create custom middleware, let's look at some practical examples of how it can be used to enhance your applications:
- Authentication: Implement token-based authentication across your application by validating tokens in a middleware, thus securing your endpoints.
- Logging: Log details about each request and response, such as endpoints accessed, response times, and status codes, for monitoring and debugging purposes.
- Rate Limiting: Protect your application from abuse by limiting the number of requests a user can make within a certain timeframe.
- Data Transformation: Modify request or response data globally, for example, to ensure all responses are formatted in a certain way.
Best Practices for Using Middleware
While custom middleware offers powerful capabilities, it's essential to use it judiciously to avoid negatively impacting your application's performance and maintainability. Here are some best practices to keep in mind:
- Keep middleware functions focused and avoid making them too large or complex.
- Be mindful of the order in which middleware is applied, as it can affect the outcome.
- Test middleware thoroughly, as issues in middleware can affect the entire application.
- Use middleware for cross-cutting concerns, and keep business logic within your endpoints.
Conclusion
Custom middleware in FastAPI opens up a world of possibilities for enhancing your web applications, from improving security and performance to ensuring consistent request and response handling. By understanding and leveraging this powerful feature, you can build more robust, efficient, and scalable web applications. Remember to follow best practices and keep your middleware focused and tested to get the most out of this functionality. With FastAPI and custom middleware, you're well-equipped to take your web applications to the next level.
As you continue to explore FastAPI and its capabilities, consider how custom middleware can be integrated into your projects to solve common problems and streamline your application architecture. Happy coding!
Recent Posts
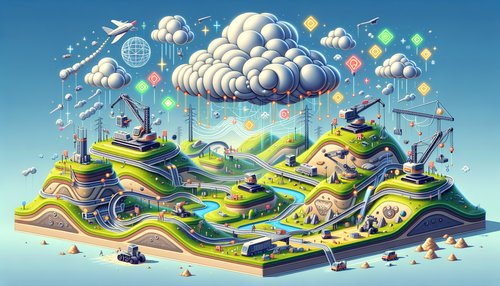
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
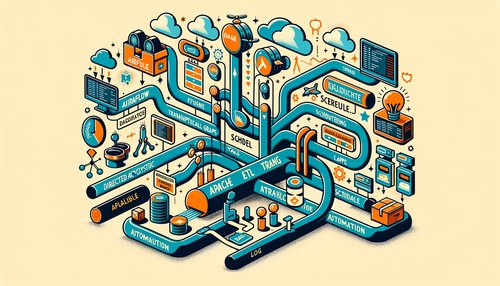
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow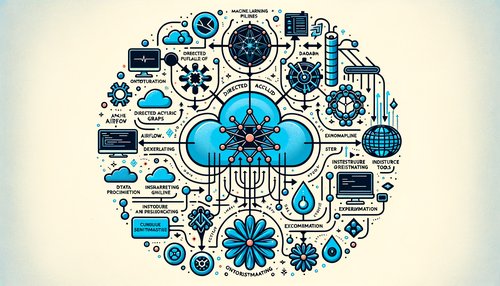
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow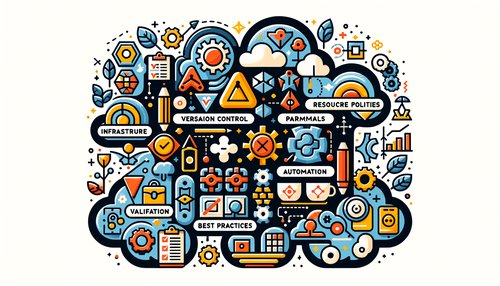
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
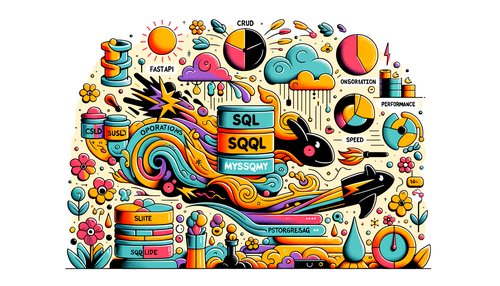