Unlocking the Power of FastAPI: Mastering JSON Compatibility with the Ultimate User Guide Encoder!
Welcome to the exciting world of FastAPI, a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. If you're looking to elevate your backend development with efficiency and ease, you've come to the right place. This comprehensive guide will dive deep into unlocking the potential of FastAPI, focusing on mastering JSON compatibility through the ultimate user guide encoder. Whether you're a seasoned developer or just starting, understanding how to efficiently handle JSON data can significantly improve your API's performance and your productivity.
Understanding JSON Compatibility in FastAPI
JSON (JavaScript Object Notation) is a lightweight data-interchange format that's easy for humans to read and write and easy for machines to parse and generate. FastAPI leverages JSON as its primary data exchange format, making understanding its compatibility crucial. FastAPI's automatic data conversion and validation mechanisms, powered by Pydantic models, ensure seamless JSON compatibility, but there's more to unlock for advanced usage and customization.
Pydantic Models: The Heart of JSON in FastAPI
At the core of FastAPI's JSON handling are Pydantic models. These models define the structure of your data, including types and validation rules, allowing for automatic parsing of request bodies and response data. Defining a Pydantic model is straightforward:
from pydantic import BaseModel
class Item(BaseModel):
name: str
description: str = None
price: float
tax: float = None
This model can automatically handle JSON data in requests and responses, validating and serializing without manual effort.
Advanced JSON Encoding with Custom Encoders
Sometimes, you'll encounter data types not directly serializable to JSON, such as datetime objects or Decimal. FastAPI provides a powerful way to handle these cases: custom JSON encoders. By defining how these types should be converted to JSON-compatible types, you ensure your API remains flexible and powerful.
Implementing a custom encoder involves extending FastAPI's JSONResponse class:
from fastapi.responses import JSONResponse
from datetime import datetime
import json
class CustomJSONResponse(JSONResponse):
def render(self, content: any) -> bytes:
return json.dumps(
content,
default=str, # Convert non-serializable types to strings
ensure_ascii=False,
allow_nan=False,
indent=None,
separators=(",", ":"),
).encode("utf-8")
This custom encoder converts datetime objects to string representation, ensuring they can be JSON-serialized.
Optimizing JSON Performance
While FastAPI and Pydantic offer excellent performance out of the box, large applications with complex data structures or high traffic might require optimization. Techniques such as lazy loading data, using Pydantic's 'orm_mode' for database models, and leveraging asynchronous database calls can significantly enhance your API's responsiveness and throughput.
Practical Tips and Tricks
To master JSON compatibility in FastAPI, consider these practical tips:
- Use Pydantic's advanced validation features to ensure data integrity, such as regex patterns, min/max values, and custom validators.
- Leverage FastAPI's dependency injection system to reuse database connections and other resources across requests efficiently.
- Profile your API's performance to identify bottlenecks in JSON serialization/deserialization and optimize accordingly.
Conclusion
Mastering JSON compatibility in FastAPI through understanding Pydantic models, implementing custom JSON encoders, and optimizing performance can transform your API development process. By leveraging these techniques, you can build more efficient, robust, and scalable web APIs. Remember, the key to mastering FastAPI is continuous learning and experimentation. So, dive in, experiment with the tips shared in this guide, and unlock the full potential of FastAPI in your projects. Happy coding!
As you continue to develop your skills, consider contributing to the FastAPI community or sharing your insights with others. The journey of mastering FastAPI and its JSON capabilities is both challenging and rewarding, and your experiences can help guide others on their path to becoming FastAPI experts.
Recent Posts
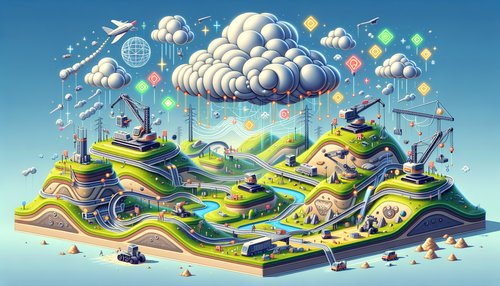
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
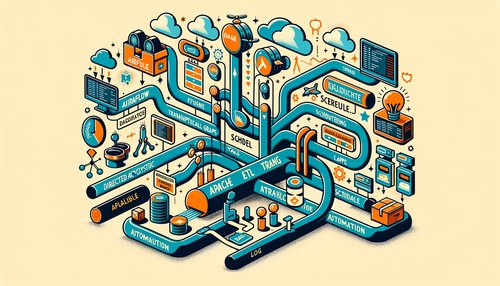
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow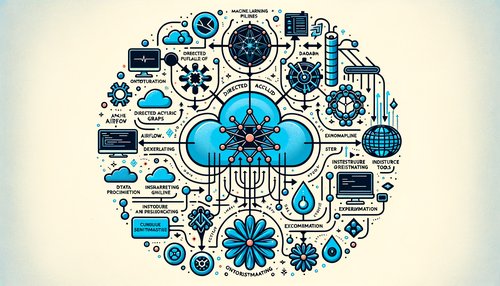
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow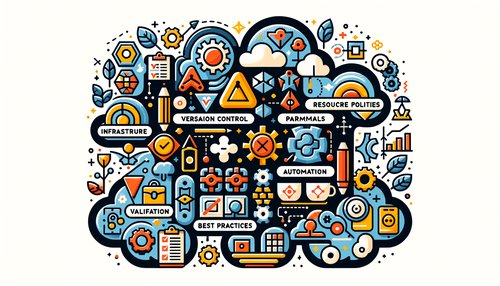
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
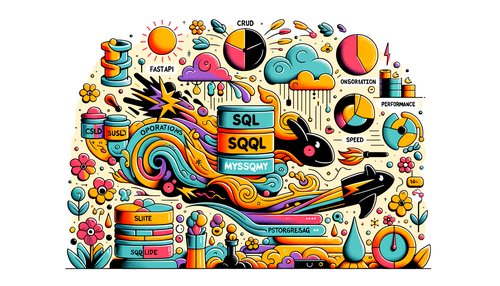