Unlocking the Power of Forms in React: A Deep Dive into Formik's Magic
Welcome to a comprehensive exploration of how Formik elevates the process of building and managing forms in React applications. Forms are the backbone of user interaction, from signing up to feedback submission. However, managing form state, handling submissions, and validating input can quickly become a tangled web of challenges. Enter Formik, a powerful library designed to take the pain out of forms in React. This blog post will delve into the core features of Formik, offering a blend of practical advice, examples, and insights to harness its full potential effectively.
Why Choose Formik?
Before diving into the technicalities, let's first understand why Formik has become a go-to solution for many developers. Handling forms in React can be verbose and cumbersome, especially when dealing with complex structures and validations. Formik provides a neat abstraction, reducing boilerplate and offering a more straightforward approach to form management. It handles form state, submission, and validation out of the box, allowing developers to focus on the unique aspects of their forms.
Getting Started with Formik
Integrating Formik into your React project is straightforward. First, you need to install Formik via npm or yarn. Once installed, you can start creating forms using either the <Formik />
component or the useFormik()
hook, depending on your preference for components or hooks. A simple Formik form includes the form state, an onSubmit function to handle form submission, and validation logic.
Example:
import React from 'react';
import { Formik, Form, Field } from 'formik';
const MyForm = () => (
<Formik
initialValues={{ name: '', email: '' }}
onSubmit={(values) => {
console.log(values);
}}
// Add validation here
>
{() => (
<Form>
<Field name="name" type="text" />
<Field name="email" type="email" />
<button type="submit">Submit</button>
</Form>
)}
</Formik>
);
Validation with Yup
Formik pairs beautifully with Yup, a JavaScript schema builder for value parsing and validation. Using Yup, you can define a schema to validate form fields. Formik integrates seamlessly with Yup, allowing you to validate forms based on your specified schema with minimal effort.
Example:
import * as Yup from 'yup';
const validationSchema = Yup.object().shape({
name: Yup.string().required('Name is required'),
email: Yup.string().email('Invalid email').required('Email is required'),
});
Advanced Features
Beyond the basics, Formik offers a range of advanced features to handle more complex form scenarios. These include handling array fields, nested object fields, and custom input components. Formik's <FieldArray />
component and the useField()
hook are particularly useful for managing dynamic forms and custom input fields, respectively.
Best Practices
To maximize the benefits of Formik, consider the following best practices:
- Keep validation logic separate: Define your validation schema outside the component to keep your form logic clean and maintainable.
- Utilize Formik's context: For deeply nested form fields, use Formik's context to avoid prop drilling.
- Leverage custom input components: Create reusable input components to simplify your form markup and enhance consistency.
Conclusion
Formik stands out as a powerful and efficient tool for managing forms in React applications. By abstracting the boilerplate and intricacies of form handling, Formik allows developers to create more reliable, maintainable, and user-friendly forms. With its integration with Yup for validation and support for advanced features, Formik is well-equipped to handle a wide range of form-related challenges. Whether you're building a simple contact form or a complex dynamic form, Formik's combination of simplicity, flexibility, and power makes it an invaluable asset in any React developer's toolkit.
As we've explored the magic of Formik, it's clear that embracing this library can significantly enhance your form management strategy. By following the discussed best practices and leveraging Formik's features to their fullest, you're well on your way to creating efficient, robust forms that elevate user experience. Dive into Formik, and unlock the true potential of forms in your React applications.
Recent Posts
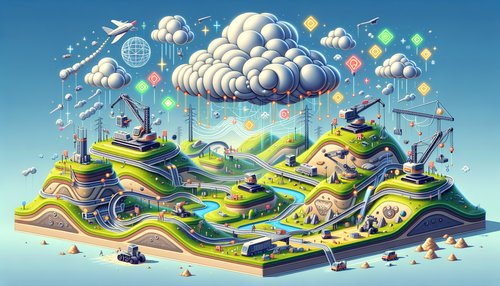
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
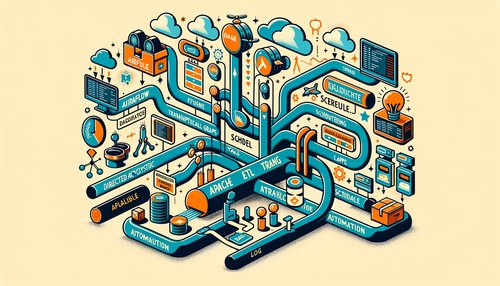
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow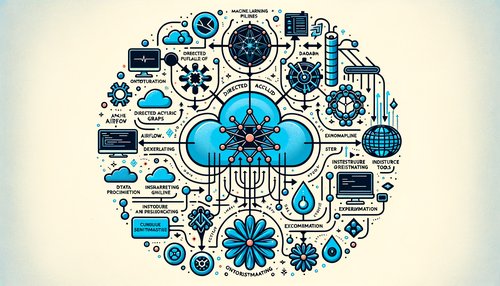
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow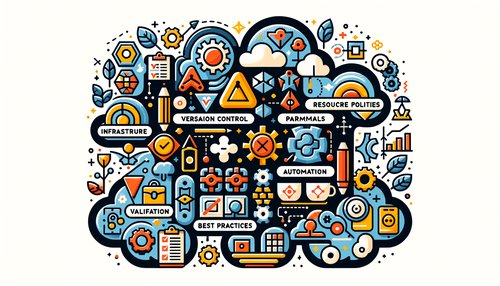
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
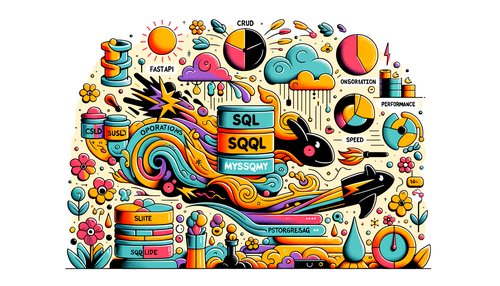