Unlocking the Power of Forms in React: A Deep Dive into Formik Mastery
Handling forms in React applications can often feel like an uphill battle, fraught with complexity and repetitive boilerplate code. Enter Formik, a powerful library that simplifies form handling in React apps, making form validation, submission, and overall management a breeze. In this blog post, we'll take a deep dive into the world of Formik, exploring its core concepts, practical applications, and advanced features to truly unlock its potential. Whether you're new to Formik or looking to elevate your form game, this guide is your ticket to mastering this essential tool in the React ecosystem.
Why Choose Formik?
Before diving into the nitty-gritty of Formik, let's first understand why it stands out among other form handling libraries. Formik is designed to handle complex form logic, validation, and UI updates efficiently, reducing the amount of code you need to write and maintain. It integrates seamlessly with React's component model, making it easy to create reusable form components. Additionally, Formik comes with out-of-the-box support for Yup, a schema builder for value parsing and validation, further streamlining the form handling process.
Getting Started with Formik
To kickstart your journey with Formik, begin by installing the library in your React project:
npm install formik --save
Next, create a simple form component. Formik's <Formik />
component encapsulates form logic, providing props for handling form submission, values, and errors. Here's a basic example:
import React from 'react';
import { Formik, Form, Field } from 'formik';
const MyForm = () => (
<Formik
initialValues={{ name: '', email: '' }}
onSubmit={(values, actions) => {
console.log(values);
actions.setSubmitting(false);
}}
>
{() => (
<Form>
<Field type="text" name="name" placeholder="Name" />
<Field type="email" name="email" placeholder="Email" />
<button type="submit">Submit</button>
</Form>
)}
</Formik>
);
export default MyForm;
This example illustrates the simplicity of creating a form with Formik, including fields for a user's name and email and a submit button.
Form Validation with Yup
Form validation is crucial to ensuring that users submit the correct data. Formik pairs beautifully with Yup for schema-based validation. Here's how you can add validation to the previous form example:
import * as Yup from 'yup';
const validationSchema = Yup.object().shape({
name: Yup.string()
.min(2, 'Too Short!')
.max(50, 'Too Long!')
.required('Required'),
email: Yup.string()
.email('Invalid email')
.required('Required'),
});
Integrate this schema with Formik by adding the validationSchema
prop to the <Formik />
component. Formik will automatically validate the form fields on submission and display the errors.
Advanced Features and Techniques
As you become more comfortable with Formik, you'll discover its flexibility and power. Here are some advanced features and techniques to further enhance your forms:
- Custom Input Components: Create reusable input components that integrate seamlessly with Formik's
<Field />
component. - Formik Context: Use Formik's context to access form state and methods anywhere in your component tree, enabling complex form layouts and interactions.
- Optimization: Leverage React's
memo
and Formik'sFastField
to optimize form performance, especially in forms with a large number of fields.
Putting It All Together
Formik is a robust solution for managing forms in React applications, offering a balance of simplicity and flexibility. By understanding its core concepts and exploring its advanced features, you can significantly reduce the complexity of form handling, validation, and state management in your projects.
Conclusion
We've covered the essentials of Formik, from basic setup and validation with Yup to advanced techniques for optimizing form performance. Formik not only simplifies form handling in React apps but also encourages best practices and clean code. As you continue to build and manage forms, remember that Formik is a tool designed to work for you, making your development process more efficient and enjoyable. Embrace the power of Formik in your next React project, and watch your form handling woes disappear.
Ready to master Formik? Dive into the documentation, experiment with its features, and join the community of developers who've discovered the joy of streamlined form management. Happy coding!
Recent Posts
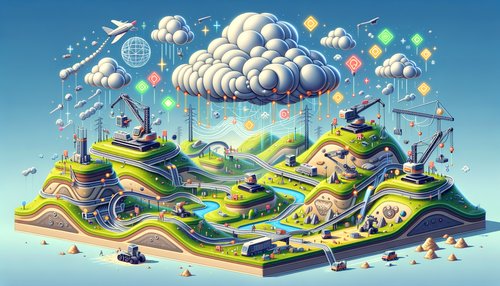
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
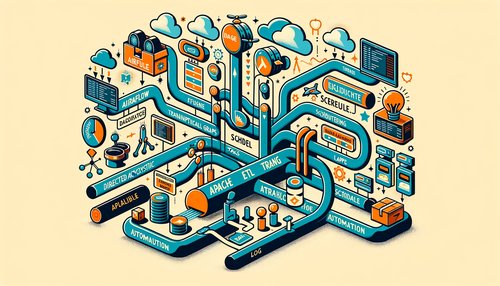
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow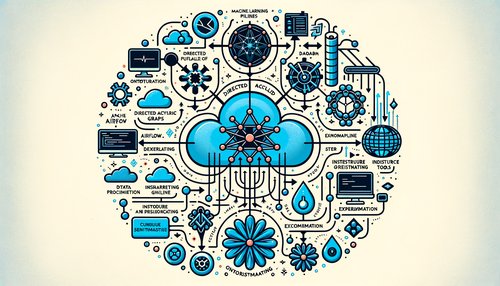
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow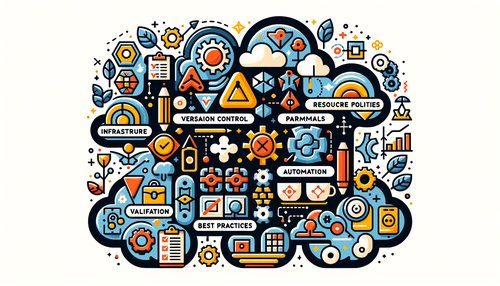
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
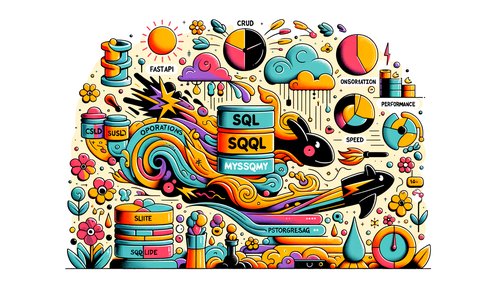