Unlocking the Power of Pandas: Master MultiIndex and Advanced Indexing Techniques
Welcome to an illuminating journey into the world of Pandas, the powerhouse Python library that has revolutionized data manipulation and analysis. If you’ve ever found yourself wrestling with complex datasets, yearning to unlock their secrets with ease and precision, this post is your key. Today, we dive deep into the art of MultiIndex and advanced indexing techniques, tools that, once mastered, will elevate your data analysis skills from competent to extraordinary. Whether you're a data science enthusiast, a seasoned analyst, or somewhere in between, mastering these techniques will open up a new realm of possibilities for your data exploration and manipulation tasks.
Understanding MultiIndex
At the heart of advanced Pandas operations lies the MultiIndex, or hierarchical indexing, which allows you to incorporate multiple index levels on an axis. This seemingly simple concept is a game-changer, enabling you to conduct sophisticated analyses and manipulations on complex datasets with relative ease.
Why Use MultiIndex? MultiIndexing allows for more natural data organization and more efficient data slicing, dicing, and summarizing. Imagine dealing with time series data for multiple categories across different geographical locations. With MultiIndex, you can structure this data in a way that reflects its hierarchical nature, making it easier to analyze patterns at different levels of granularity.
Creating a MultiIndex DataFrame
Creating a MultiIndex DataFrame is straightforward. You can either set multiple indexes upon creation using the pd.DataFrame()
constructor or by setting a MultiIndex after the fact with the set_index()
method on an existing DataFrame.
import pandas as pd
# Example: Creating a MultiIndex DataFrame
data = {
'Category': ['Cat1', 'Cat1', 'Cat2', 'Cat2'],
'Subcategory': ['Sub1', 'Sub2', 'Sub1', 'Sub2'],
'Value': [1, 2, 3, 4]
}
df = pd.DataFrame(data).set_index(['Category', 'Subcategory'])
print(df)
Advanced Indexing Techniques
With your MultiIndex DataFrame in place, the next step is to master advanced indexing techniques that will allow you to slice and dice your data in powerful ways.
loc and iloc
The loc
and iloc
methods are your bread and butter for data selection. loc
is label-based, meaning you use it with the names of your rows or columns, while iloc
is position-based, for when you want to select by integer position.
When working with MultiIndex DataFrames, these methods become even more potent, allowing for precise selection across multiple levels.
# Example: Using loc with a MultiIndex DataFrame
print(df.loc[('Cat1', 'Sub1')])
# Example: Selecting a slice
print(df.loc['Cat1'])
xs Method
The xs
method provides a more convenient way to select data across one level of a MultiIndex. It’s particularly useful when you want to select data across all levels but one.
# Example: Using xs to select data
print(df.xs('Sub1', level='Subcategory'))
Advanced Slicing
Advanced slicing techniques allow you to combine multiple conditions and slicing methods to refine your data selection even further.
# Example: Advanced slicing with loc
print(df.loc[('Cat1', slice(None)), :])
Practical Tips and Insights
As you become more comfortable with MultiIndex and advanced indexing, here are a few tips and insights to keep in mind:
- Keep Performance in Mind: While MultiIndexing is powerful, it can impact performance on very large datasets. Consider whether a MultiIndex is the best choice for your specific scenario.
- Use Sorting: Ensure your MultiIndex is sorted with
sort_index()
for optimal performance, especially when slicing. - Reindexing: Sometimes, you might need to reset your index or change its structure. Familiarize yourself with methods like
reset_index()
andreindex()
to modify your DataFrame's indexing effectively.
Conclusion
Mastering MultiIndex and advanced indexing techniques in Pandas opens up a world of data manipulation possibilities, allowing you to handle complex datasets with ease. By understanding how to efficiently organize, select, and slice your data, you can uncover insights that would be difficult to achieve otherwise. Remember, the key to becoming proficient in these techniques is practice. So, dive into your data, experiment with these methods, and watch your data analysis skills soar to new heights.
As we conclude this journey into the power of Pandas, take a moment to reflect on the techniques covered and consider how they can be applied to your next data project. With these tools in your arsenal, you’re well on your way to becoming a Pandas power user. Happy data wrangling!
Recent Posts
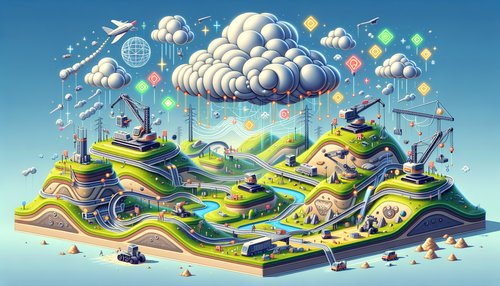
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
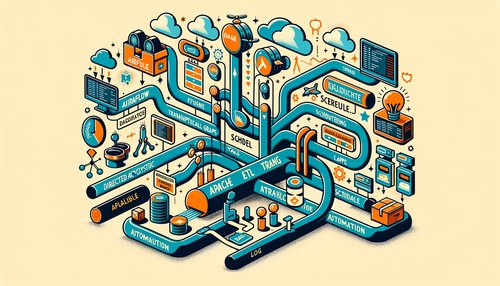
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow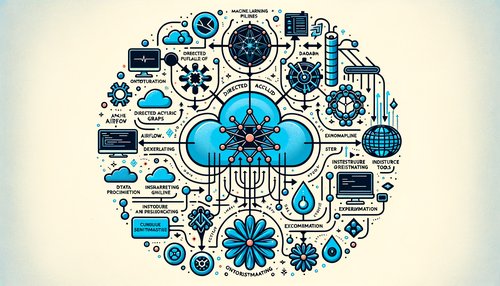
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow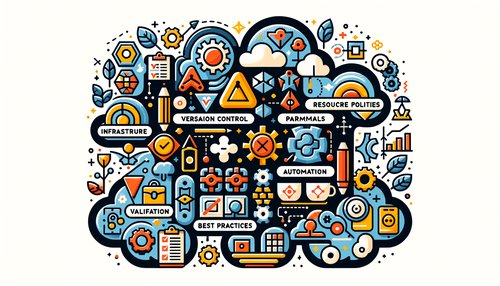
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
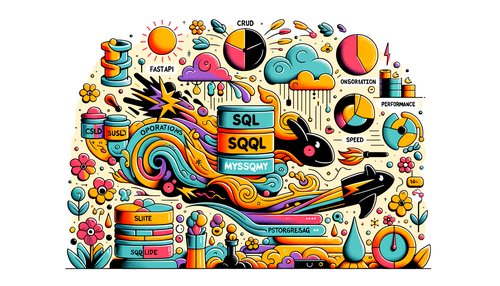