Unlocking the Power of Relational Databases in FastAPI: The Ultimate User Guide to SQL Mastery
Welcome to a comprehensive exploration designed to unlock the full potential of relational databases within the dynamic environment of FastAPI. Whether you're a seasoned developer or just starting out, understanding how to effectively integrate SQL with FastAPI can revolutionize how you build and manage your applications. This guide promises to take you on a detailed journey through the intricacies of leveraging relational databases to their fullest, ensuring you achieve SQL mastery for robust application development.
Why FastAPI with SQL?
Before diving into the technicalities, let's understand why marrying FastAPI with SQL databases is a game-changer for developers. FastAPI, known for its high performance and ease of use, provides an excellent framework for building APIs. When combined with the power and reliability of SQL databases, developers can create efficient, scalable, and secure applications. This synergy allows for handling complex queries, transactions, and relationships with ease, providing a solid foundation for any application.
Setting the Stage: Integrating SQL with FastAPI
Integrating SQL into FastAPI requires a foundational setup that involves choosing the right library, establishing a database connection, and creating models that reflect your database schema. Libraries like SQLAlchemy or databases offer asynchronous support, making them perfect candidates for FastAPI applications. Begin by installing your chosen library, setting up your database connection using a connection string, and then proceed to define your models. This setup paves the way for seamless interaction between your FastAPI application and your SQL database.
Practical Tips:
- Choose a library that supports asynchronous operations to take full advantage of FastAPI's async features.
- Use environment variables for your database connection strings to enhance security.
- Reflect your database schema accurately in your models to ensure data integrity.
CRUD Operations: The Backbone of Your Application
At the heart of integrating SQL with FastAPI are CRUD (Create, Read, Update, Delete) operations. These operations are essential for interacting with your database and manipulating data. Implementing CRUD operations involves defining asynchronous functions that use SQL queries or ORM methods to perform actions on the database. Through FastAPI's path operations, you can then expose these functions as endpoints, allowing for data manipulation through API calls.
Example:
Here's a simple example of a Read operation using SQLAlchemy:
from fastapi import FastAPI
from sqlalchemy import async_session
from models import User
app = FastAPI()
@app.get("/users/{user_id}")
async def read_user(user_id: int):
async with async_session() as session:
user = await session.get(User, user_id)
return user
Advanced Techniques: Join Queries, Transactions, and More
Moving beyond basic CRUD operations, mastering join queries, transactions, and other advanced SQL techniques is crucial for developing complex applications. Join queries allow you to retrieve data from multiple tables based on a related column, enabling the creation of rich, informative responses for your API endpoints. Transactions ensure data integrity by grouping multiple operations into a single, atomic operation, which either completely succeeds or fails, preventing partial data updates.
Insights:
- Use join queries to aggregate data from multiple tables, enhancing the richness of your API responses.
- Leverage transactions to maintain data integrity, especially in operations involving multiple steps or updates.
- Explore SQL's window functions for complex data analytics and reporting within your FastAPI applications.
Security and Performance Optimization
Ensuring the security and performance of your FastAPI application is paramount. When it comes to SQL databases, use prepared statements to safeguard against SQL injection attacks. Additionally, optimize your queries by indexing frequently accessed columns and using pagination for large datasets. These practices not only enhance security but also improve the responsiveness and scalability of your application.
Practical Tips:
- Always use prepared statements or ORM methods to prevent SQL injection.
- Implement indexing on columns that are frequently used in WHERE clauses or as join keys.
- Use pagination for endpoints that return large amounts of data to improve load times and resource usage.
Conclusion
Integrating relational databases with FastAPI opens a realm of possibilities for developing robust, efficient, and secure applications. By understanding and implementing the practices outlined in this guide, you'll be well on your way to achieving SQL mastery within your FastAPI projects. Remember, the journey to mastering SQL with FastAPI is ongoing, and there's always more to learn and explore. So, keep experimenting, refining your skills, and pushing the boundaries of what you can build. Happy coding!
As a final thought, consider contributing to the FastAPI and SQL communities. Sharing your knowledge, experiences, and code can help others on their journey, fostering a vibrant ecosystem of collaborative development.
Recent Posts
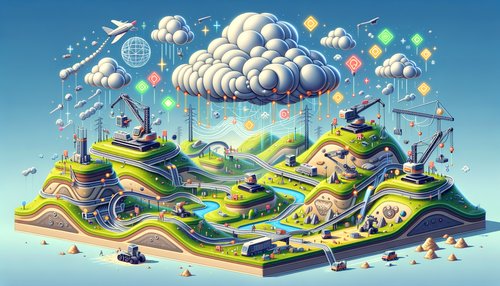
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
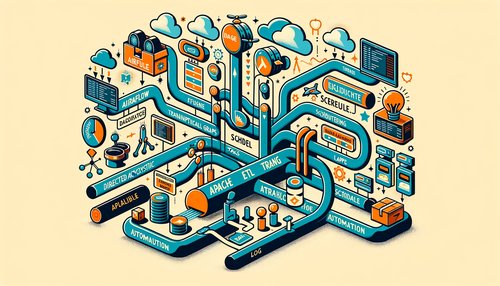
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow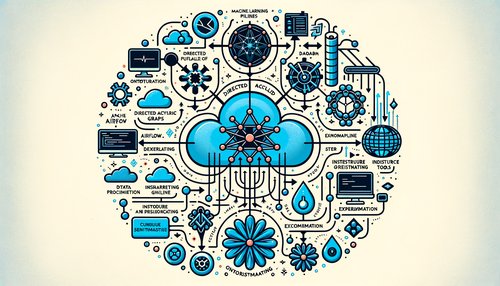
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow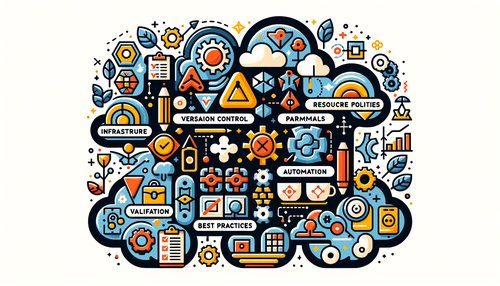
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
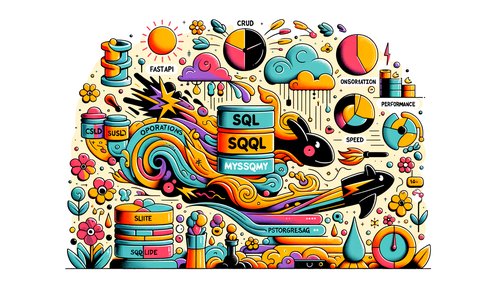