Unlocking the Power of Terraform: Crafting Complex Configurations with Expressions for Expressions
Terraform has revolutionized how we manage and provision cloud infrastructure, enabling us to define infrastructure as code that is easy to understand, share, and automate. One of the most powerful features in Terraform is the use of expressions. In this blog post, we will delve deep into the use of expressions for crafting complex configurations, providing practical tips, examples, and insights along the way.
What are Terraform Expressions?
Expressions in Terraform are used to compute or manipulate values. They can be as simple as a single value like a string or as complex as a combination of operations and functions. Understanding how to leverage expressions will allow you to create more dynamic and flexible configurations.
Using Basic Expressions
At the most basic level, expressions can be constants or variables. For example:
variable "region" {
default = "us-west-1"
}
resource "aws_instance" "example" {
ami = "ami-123456"
instance_type = "t2.micro"
availability_zone = "${var.region}-a"
}
In the above example, the region variable is combined with a string to form an availability zone using a basic expression. This is a simple yet powerful way to craft dynamic configurations.
Combining Expressions for Greater Flexibility
As you grow more comfortable with basic expressions, you can start combining them to create more complex logic. For example, conditional expressions allow you to define different values based on certain conditions:
resource "aws_instance" "example" {
ami = var.environment == "production" ? "ami-prod" : "ami-dev"
instance_type = "t2.micro"
}
In this example, the AMI used by the AWS instance changes based on the value of the environment
variable. Conditional expressions are immensely useful for managing different environments or configurations based on external inputs.
Leveraging Functions within Expressions
Terraform offers a rich set of built-in functions that can be used within expressions to manipulate and transform your data. Here are some frequently used functions:
length
: Returns the length of a string or the number of elements in a list or map.merge
: Combines multiple maps into one.lookup
: Retrieves the value of a specified key from a map.join
: Concatenates elements of a list into a single string.
Using these functions enables more sophisticated data processing within your configuration files. For example:
variable "tags" {
type = map(string)
}
resource "aws_instance" "example" {
ami = "ami-123456"
instance_type = "t2.micro"
tags = merge({
Name = "example-instance"
}, var.tags)
}
Here, the merge
function is used to combine a default set of tags with user-provided tags, making the configuration more flexible and user-friendly.
Creating Reusable Modules with Expressions
One of the best practices in Terraform is creating reusable modules. Expressions can play a key role in making your modules more flexible and powerful. For example:
module "vpc" {
source = "./modules/vpc"
cidr_block = var.environment == "production" ? "10.0.0.0/16" : "10.1.0.0/16"
}
In this module configuration, we use a conditional expression to change the CIDR block of a VPC based on the environment, allowing the same module to be reused in different contexts while adapting its behavior dynamically.
Practical Tips for Using Expressions Effectively
Here are some tips to get the most out of Terraform expressions:
- Keep expressions simple and readable. Complex logic should be broken down into multiple smaller expressions or outputs.
- Use comments to describe non-trivial expressions, making the configuration easier to understand for others.
- Test expressions thoroughly to ensure they produce the expected results, especially when combining multiple expressions or functions.
- Leverage Terraform's
plan
command to preview the impact of your expressions before applying changes.
Conclusion
Terraform expressions are a powerful tool for creating dynamic, flexible, and reusable configurations. From simple calculations and string manipulations to complex conditionals and function calls, expressions can help you unlock the full potential of Terraform. By using the techniques and tips provided in this blog post, you will be able to craft sophisticated infrastructure configurations that are both maintainable and scalable.
Happy Terraforming! If you found this post helpful, be sure to share it with others who might benefit from learning about Terraform expressions.
Recent Posts
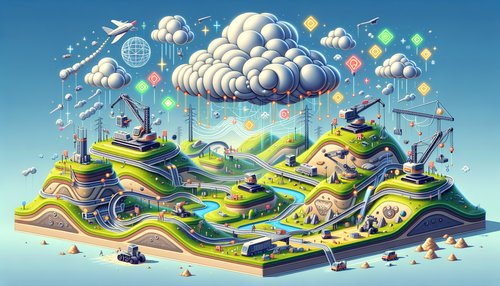
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
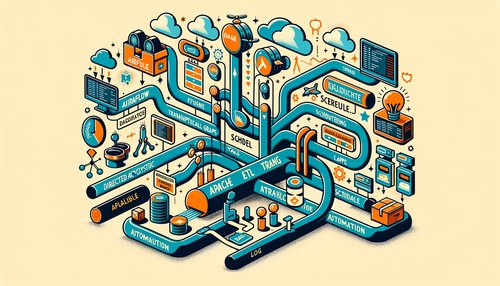
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow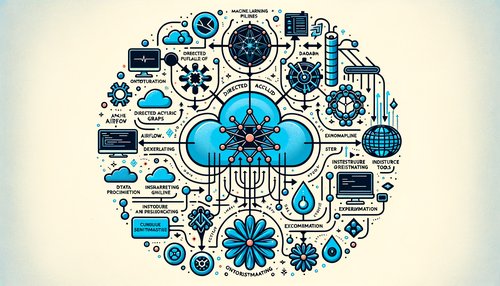
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow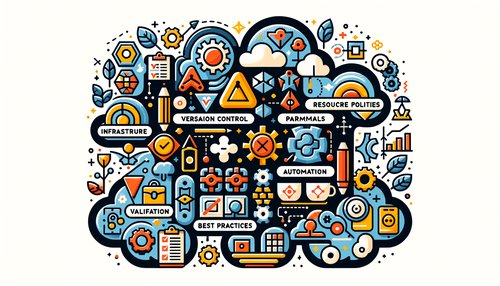
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
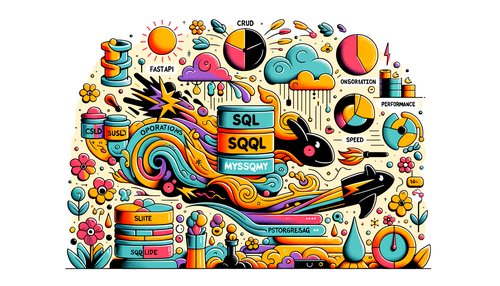