Unlocking the Power of Terraform: Mastering Expressions and Dynamic Blocks for Efficient Infrastructure Management
Terraform has revolutionized infrastructure as code (IaC) by allowing developers to define and provision data center infrastructure using a high-level configuration language. But to unlock its full potential, it's crucial to master its advanced features, particularly expressions and dynamic blocks. In this blog post, we'll explore how these features can make your infrastructure management more efficient and maintainable.
Understanding Terraform Expressions
Expressions are a fundamental part of Terraform configurations. They allow you to compute values and specify conditions that define the infrastructure. Understanding how to use expressions effectively can significantly optimize your Terraform code.
The Basics of Terraform Expressions
Terraform supports several types of expressions, including literals, references, and complex data types like lists and maps. For example:
variable "region" {
default = "us-west-1"
}
resource "aws_instance" "example" {
ami = "ami-123456"
instance_type = "t2.micro"
count = var.region == "us-west-1" ? 3 : 1
}
In the above code, the count
parameter uses a conditional expression to determine the number of instances to create based on the region.
Using Functions and Operators
Terraform provides a wide array of built-in functions and operators that you can leverage within expressions. Functions like element
, lookup
, and join
help manipulate data, while arithmetic and logical operators allow for complex conditionals.
variable "instance_type" {
type = string
default = "t2.micro"
}
resource "aws_instance" "web" {
ami = data.aws_ami.ubuntu.id
instance_type = var.instance_type
tags = {
Name = join("-", ["web", var.instance_type])
}
}
In this example, the join
function is used to create a tag name that combines the string "web" with the instance type.
Diving into Dynamic Blocks
Dynamic blocks in Terraform allow for the creation of repeatable nested blocks based on expressions. This is especially useful for configurations that need to adapt to varying conditions or datasets.
When to Use Dynamic Blocks
Consider using dynamic blocks when you need to generate multiple similar nested blocks, such as security group rules or instance configurations, based on variable input. This enhances code reusability and reduces redundancy.
Creating Dynamic Blocks
Dynamic blocks are defined using the dynamic
keyword and a block iterator. Here's an example that dynamically generates security group rules based on a list of ports:
variable "ingress_ports" {
type = list(number)
default = [80, 443]
}
resource "aws_security_group" "example" {
name = "example_sg"
vpc_id = var.vpc_id
dynamic "ingress" {
for_each = var.ingress_ports
content {
from_port = ingress.value
to_port = ingress.value
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
}
}
In this code, the dynamic
block generates an ingress
rule for each port specified in the ingress_ports
variable.
Practical Tips for Efficient Infrastructure Management
Here are some tips to help you manage your Terraform configurations more efficiently:
- Use variable files and workspaces to manage different environments.
- Leverage Terraform modules to encapsulate and reuse code across projects.
- Take advantage of version control to track changes and collaborate with team members.
- Regularly validate and format your configuration with
terraform validate
andterraform fmt
.
Conclusion
Mastering Terraform expressions and dynamic blocks can significantly enhance your infrastructure management skills. By using these powerful features, you can create more flexible, maintainable, and scalable infrastructure configurations. Start experimenting with expressions and dynamic blocks in your Terraform projects today, and unlock the full potential of infrastructure as code.
Recent Posts
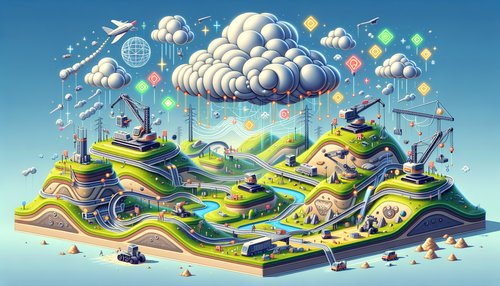
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
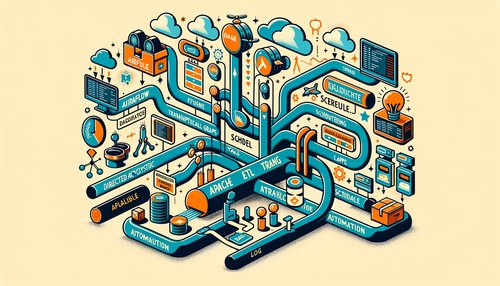
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow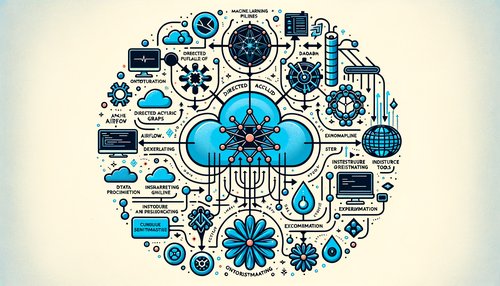
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow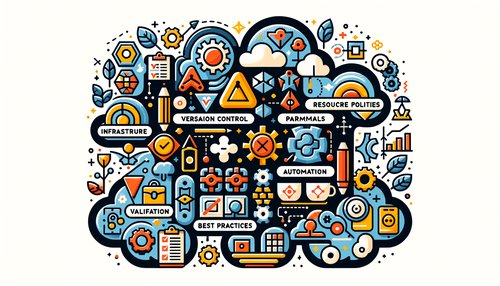
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
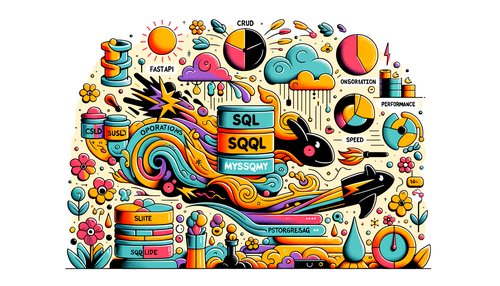