Unlocking the Power of Words: A Deep Dive into the Pandas User Guide for Text Data Mastery
Welcome to a journey into the heart of text data analysis with Python's Pandas library. In the age of data, understanding and manipulating text data is a crucial skill for data scientists and analysts. Whether you're mining social media sentiment, analyzing customer feedback, or working with any form of textual data, Pandas offers a powerful toolkit to unlock insights from words. This blog post will guide you through mastering text data with Pandas, from basic operations to advanced text processing techniques. So, let's dive in and explore how to harness the power of words with precision and ease.
Getting Started with Text Data in Pandas
Before diving into the advanced functionalities, it's essential to understand the basics of handling text data in Pandas. Text data in Pandas is usually represented as a Series or DataFrame column consisting of strings. To get started, you'll need to familiarize yourself with the str
accessor, which provides a way to vectorize string operations and apply them to each element in a Series. Here's a quick example:
import pandas as pd
# Sample series of text data
data = pd.Series(['Pandas is powerful', 'Python is versatile', 'Data Science is fascinating'])
# Convert all text to uppercase
data_upper = data.str.upper()
print(data_upper)
This simple example demonstrates how to apply a string method to an entire Series to convert all text to uppercase. The str
accessor is your gateway to a wide range of string operations in Pandas.
Text Data Cleaning and Preprocessing
Cleaning and preprocessing are crucial steps in text data analysis. This involves removing unnecessary characters, correcting typos, standardizing text format, and more. Pandas provides various methods to accomplish these tasks efficiently. Here are a few practical tips:
- Trimming whitespace: Use
data.str.strip()
to remove leading and trailing spaces. - Removing punctuation: Apply a regex replacement using
data.str.replace('[^\w\s]', '')
to eliminate punctuation. - Handling missing values: Use
data.fillna('')
to replace NaN values with empty strings, ensuring your text operations run smoothly.
Remember, the goal of preprocessing is to standardize your text data, making it easier to analyze and derive insights.
Advanced Text Processing
Once your data is clean, you can move on to more advanced text processing tasks. Pandas, combined with libraries like NLTK or spaCy, can be used for tokenization, stopword removal, and even sentiment analysis. Here's an example of how to tokenize text data in a Pandas Series:
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
# Sample series of text data
data = pd.Series(['Pandas makes data manipulation easy.', 'NLTK is great for natural language processing.'])
# Tokenize text
data_tokenized = data.apply(lambda x: word_tokenize(x))
print(data_tokenized)
This example demonstrates how to tokenize each string in the Series, breaking down sentences into individual words. Tokenization is a foundational step for many NLP tasks, such as frequency analysis, sentiment analysis, and more.
Text Data Analysis
With your text data cleaned and processed, you're now ready to perform analysis. Pandas offers powerful tools for this purpose, like the value_counts()
method, which can be used to count word frequencies or the groupby()
method for aggregating data. Analyzing text data often involves exploring word frequencies, identifying common phrases, or calculating sentiment scores. By combining Pandas with NLP libraries, you can unlock a wide range of analytical possibilities.
Summary
In this post, we've explored the essentials of handling text data with Pandas, from basic operations to advanced text processing techniques. We've covered how to clean and preprocess text data, apply tokenization, and perform text analysis. By mastering these skills, you can unlock valuable insights from textual data, enhancing your data science projects.
As a final thought, remember that the power of words is immense. With the tools and techniques discussed, you're well-equipped to harness this power, turning raw text into actionable insights. So, go ahead, dive into your text data, and unlock the stories they hold. Happy analyzing!
Recent Posts
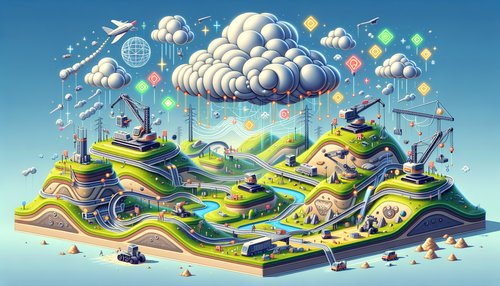
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
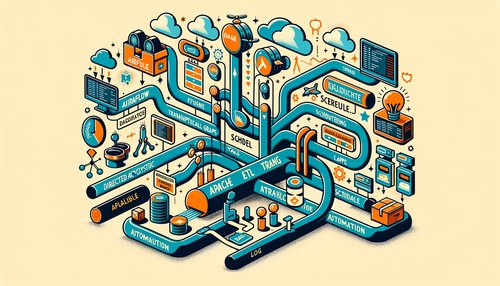
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow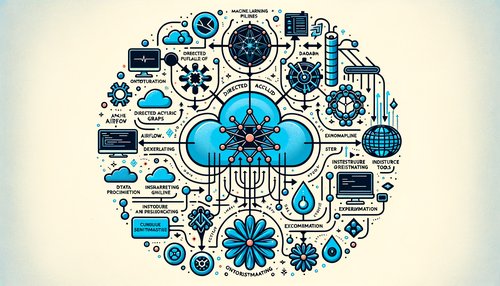
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow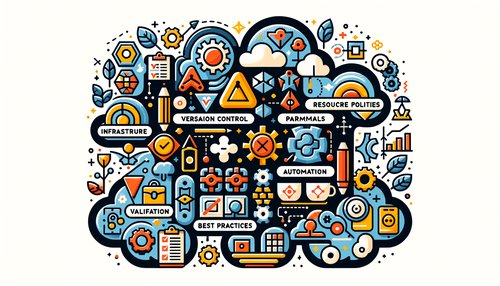
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
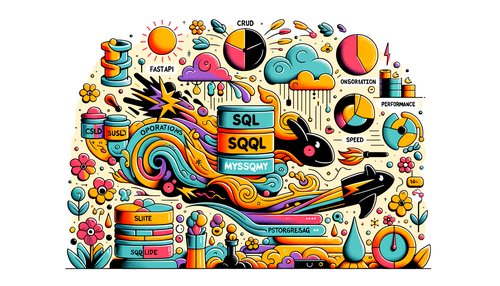