Unlocking the Secrets of Data: An Introductory Guide to Pandas' Powerful Structures
Welcome to the fascinating world of data analysis with Python! In this blog post, we're going to dive deep into the heart of data manipulation and analysis using one of the most powerful tools available to data scientists and analysts alike: Pandas. Whether you're a beginner just starting out in the field of data science or a seasoned professional looking to brush up on your skills, this guide is designed to unlock the secrets of data through the powerful structures provided by Pandas. Get ready to transform raw data into insightful information that can drive decision-making processes and fuel innovation.
Introduction to Pandas
Pandas is an open-source data analysis and manipulation tool built on top of the Python programming language. It offers data structures and operations for manipulating numerical tables and time series, making it a quintessential tool for data munging/wrangling. At the core of Pandas' functionality are its two primary data structures: Series and DataFrame. These structures are designed to handle a vast array of data types and are optimized for performance, making Pandas an indispensable part of the data scientist's toolkit.
Understanding Pandas Series
A Series is a one-dimensional array-like object that can hold many data types, including integers, strings, and floating-point numbers, among others. Each element in a Series is associated with an index, the default of which is a sequence of integers starting from 0. However, indices in Pandas are highly flexible, allowing for labeling that can make data handling more intuitive.
import pandas as pd
s = pd.Series([1, 3, 5, np.nan, 6, 8])
print(s)
This simple example demonstrates how to create a Series in Pandas, including the handling of missing data represented by 'np.nan'.
Mastering the DataFrame
The DataFrame is perhaps the most critical data structure in Pandas. It represents a two-dimensional, size-mutable, and potentially heterogeneous tabular data structure with labeled axes (rows and columns). DataFrames can be thought of as collections of Series objects that share the same index. This structure is incredibly versatile, allowing for a wide range of operations including data manipulation, aggregation, and visualization.
data = {'Name': ['John', 'Anna', 'Peter', 'Linda'],
'Age': [28, 34, 29, 32],
'City': ['New York', 'Paris', 'Berlin', 'London']}
df = pd.DataFrame(data)
print(df)
This example illustrates how to create a DataFrame from a dictionary of lists, showcasing the simplicity with which structured data can be organized and manipulated with Pandas.
Data Manipulation and Analysis
One of the strengths of Pandas is its extensive set of features for data manipulation and analysis. From sorting and filtering to grouping and aggregating data, Pandas provides powerful and efficient methods to explore and analyze datasets. The library also includes functions for handling missing data, merging datasets, and performing time-series analysis, making it a comprehensive tool for all stages of data analysis.
Practical Tips and Tricks
- Data Cleaning: Use the
.dropna()
method to remove missing values and the.fillna()
method to replace them with a specific value. - Data Filtering: Use boolean indexing to filter data. For example,
df[df['Age'] > 30]
retrieves all rows where the age is greater than 30. - Data Aggregation: The
.groupby()
method is incredibly powerful for aggregating data based on categories.
Conclusion
In this blog post, we've only scratched the surface of what's possible with Pandas. By understanding the basics of Series and DataFrame structures, along with some essential data manipulation techniques, you're well on your way to unlocking the vast potential of data analysis with Pandas. As you continue to explore, remember that the real power of data analysis comes from the insights that you can generate by asking the right questions and using the tools at your disposal to find answers.
Whether you're analyzing financial records, customer data, or scientific research, Pandas provides the foundation you need to uncover the stories hidden within your data. So, dive in, start experimenting, and unlock the secrets of your data today!
Recent Posts
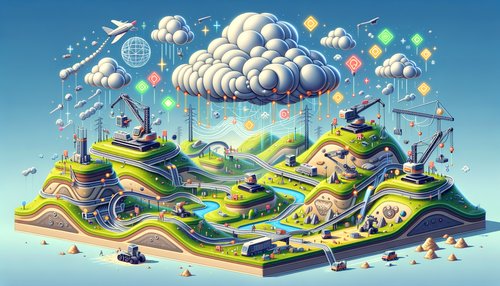
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
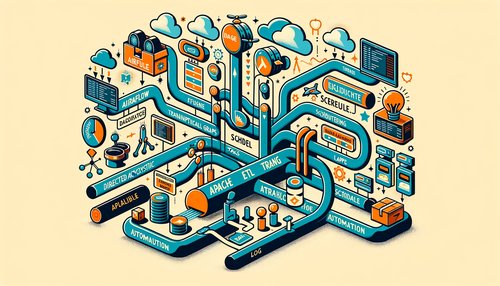
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow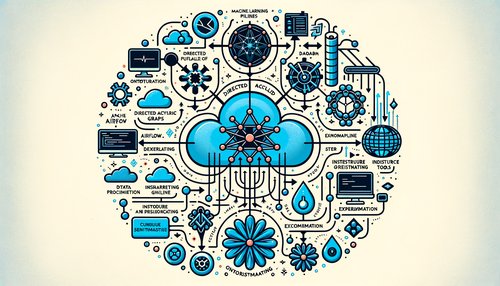
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow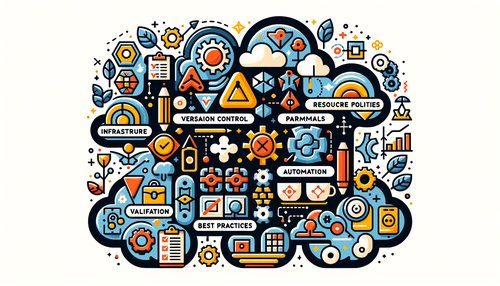
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
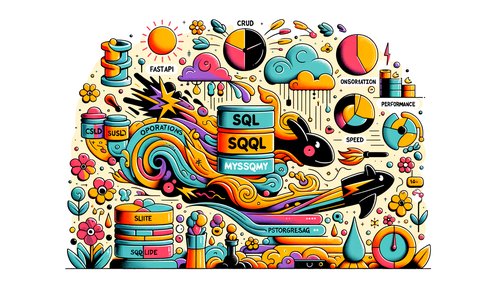