Unlocking the Secrets of FastAPI: Mastering Cookie Parameters for Enhanced User Guides
Welcome to a deep dive into one of the most powerful yet underutilized features of FastAPI: cookie parameters. In this comprehensive guide, we're going to unlock the secrets behind effectively using cookies to improve user experiences and guide developers through mastering this crucial aspect of web development. Whether you're a seasoned developer or new to FastAPI, understanding how to leverage cookies can significantly enhance the functionality and user-friendliness of your web applications. Let's embark on this journey together and explore how cookie parameters can be your ally in creating intuitive, efficient, and secure web applications.
Understanding Cookies in FastAPI
Before we delve into the intricacies of cookie parameters, let's first understand what cookies are and how they function within FastAPI. Cookies are small pieces of data stored on the client's computer by the web browser while browsing a website. They play a crucial role in enhancing user experience by remembering users' actions and preferences. FastAPI, a modern, fast (high-performance) web framework for building APIs with Python 3.7+ based on standard Python type hints, provides comprehensive support for handling cookies, making it easier for developers to implement this functionality in their applications.
Setting Up Cookies
Setting cookies in FastAPI is straightforward. When you define an endpoint, you can use FastAPI's Response
object to set cookies. Here's a simple example:
from fastapi import FastAPI, Response app = FastAPI() @app.get("/set-cookie") def set_cookie(response: Response): response.set_cookie(key="user_id", value="123456") return {"message": "Cookie is set"}
This snippet demonstrates how to set a cookie named user_id
with a value of 123456
. Whenever this endpoint is accessed, FastAPI sets the specified cookie in the user's browser.
Reading Cookies
Reading cookies is just as intuitive in FastAPI. You can access the cookies sent by the client in your endpoint by including a Cookie
parameter. Here's how:
from fastapi import FastAPI, Cookie app = FastAPI() @app.get("/get-cookie") def get_cookie(user_id: str = Cookie(None)): return {"user_id": user_id}
This code retrieves the value of the user_id
cookie. If the cookie is not present, it defaults to None
.
Best Practices for Using Cookies
While cookies are incredibly useful, they should be used responsibly to ensure the security and privacy of your users. Here are some best practices to keep in mind:
- Use secure cookies: Always set cookies with the
secure
flag, especially if they contain sensitive information. This ensures cookies are sent only over HTTPS. - HttpOnly cookies: To prevent cross-site scripting (XSS) attacks, mark cookies as
HttpOnly
. This makes them inaccessible to JavaScript running in the browser. - Set cookie domains and paths: Be specific about which domains and paths can access your cookies. This limits the scope of the cookie and enhances security.
Advanced Cookie Management
For more advanced scenarios, such as creating session cookies or implementing cookie-based authentication, FastAPI provides flexible tools to manage these requirements efficiently. Utilizing dependency injection and middleware, you can create reusable components for cookie management that can be applied across your application.
Session Cookies
Session cookies are temporary and are deleted when the user closes their browser. They are ideal for maintaining user sessions without requiring login credentials for every request. Here's an example of how you might implement session cookies in FastAPI:
# This is a simplified example. In production, you should use secure and HttpOnly flags. from fastapi import FastAPI, Response, Depends app = FastAPI() def fake_login(username: str, response: Response): response.set_cookie(key="session_token", value="fake_session_token") @app.get("/login") def login(response: Response): fake_login("user123", response) return {"message": "User logged in"}
Conclusion
Mastering cookie parameters in FastAPI can significantly enhance the functionality and security of your web applications. By understanding how to set, retrieve, and manage cookies, you can create more personalized and user-friendly experiences. Remember to follow best practices for cookie security and privacy to protect your users. As you become more comfortable with FastAPI's cookie management features, you'll unlock new possibilities for your web applications that can set you apart from the competition. Start experimenting with cookies in your FastAPI projects today, and see the difference they can make!
Happy coding!
Recent Posts
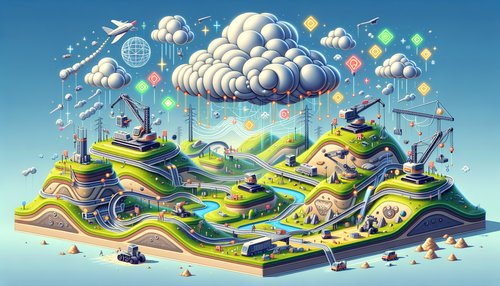
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
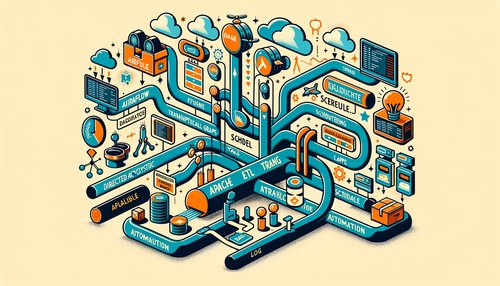
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow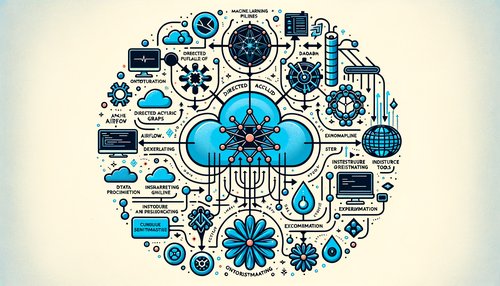
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow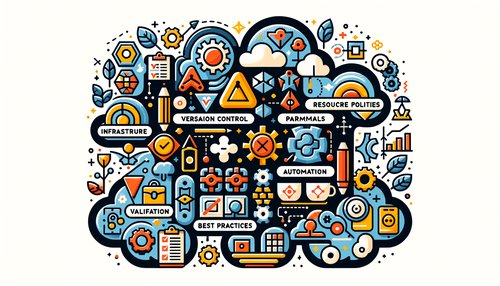
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
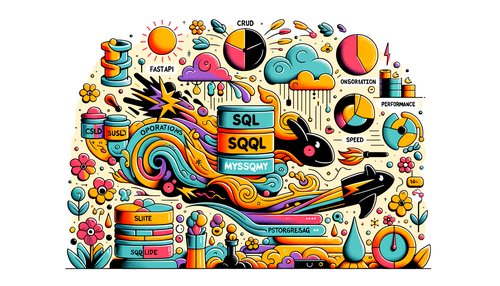