Unlocking the Secrets of Missing Data: A Comprehensive Guide to Mastering Pandas
Welcome to the journey of mastering one of the most critical aspects of data analysis with Pandas: handling missing data. Whether you are a data science enthusiast, a budding analyst, or a seasoned professional, missing data is a challenge you've likely encountered. This comprehensive guide is designed to equip you with the knowledge and skills to effectively manage and manipulate missing data, ensuring your datasets are accurate and your analyses are robust. Let's dive into the world of Pandas and unlock the secrets of missing data together!
Understanding Missing Data
Before we tackle the how-to, it's crucial to understand the what and why. Missing data occurs for various reasons: from errors in data collection to intentional omission where data is not applicable. Recognizing the type of missing data you're dealing with is the first step towards effective management. In Pandas, missing data is usually represented by NaN
(Not a Number) or None
.
Identifying Missing Data in Pandas
Identifying missing data is a preliminary step in the data cleaning process. Pandas provides several functions to make this task easier, such as isnull()
and notnull()
. These functions can be applied to a DataFrame or Series to detect missing values, allowing you to get a clear picture of the extent and distribution of missing data in your dataset.
import pandas as pd
# Sample DataFrame
df = pd.DataFrame({'A': [1, 2, None, 4],
'B': [None, 2, 3, 4],
'C': [1, None, 3, 4]})
# Identifying missing values
print(df.isnull())
Handling Missing Data
Once you've identified the missing data, the next step is deciding how to handle it. The two main strategies are deletion and imputation. Deletion involves removing records with missing values, while imputation involves replacing missing values with substitute values. The choice between these strategies depends on the nature of your data and your analysis goals.
Deletion
Deletion is straightforward with Pandas using the dropna()
method. You can choose to drop rows or columns that contain missing values depending on your needs. However, deletion is only advisable when the missing data is not significant to your analysis, as it can lead to the loss of valuable information.
# Dropping rows with any missing values
df.dropna()
Imputation
Imputation is a more sophisticated approach to handling missing data. Pandas offers the fillna()
method, which allows you to replace missing values with a specific value, the mean, median, or mode of the column. Imputation helps preserve your data's integrity, especially when the missing data cannot be ignored.
# Replacing missing values with the mean of the column
df.fillna(df.mean())
Advanced Techniques
Beyond basic deletion and imputation, several advanced techniques can be more effective in certain scenarios. For example, interpolation methods (linear, quadratic, etc.) provided by Pandas can be particularly useful for time series data. Additionally, machine learning models can predict missing values based on the rest of the data, although this approach requires a higher level of sophistication.
Summary
Handling missing data is an essential skill in data analysis, and Pandas provides a powerful set of tools to deal with this challenge effectively. By understanding the nature of your missing data and applying appropriate strategies—whether it's deletion, imputation, or more advanced techniques—you can ensure the accuracy and integrity of your analyses. Remember, the goal is not just to deal with missing data but to do so in a way that enhances your overall analysis. Happy data cleaning!
Now that you've unlocked the secrets of missing data in Pandas, it's time to put these skills into practice. Dive into your datasets, explore the missing data, and choose the best strategy to handle it. Your journey towards mastering Pandas and becoming a proficient data analyst is well underway!
Recent Posts
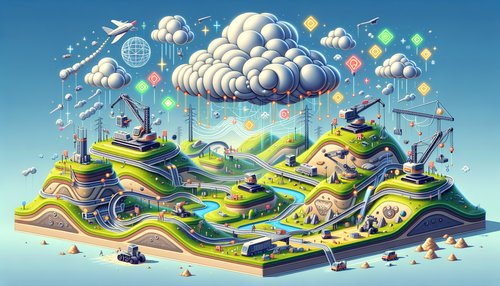
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
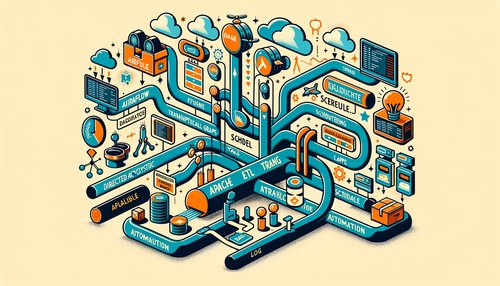
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow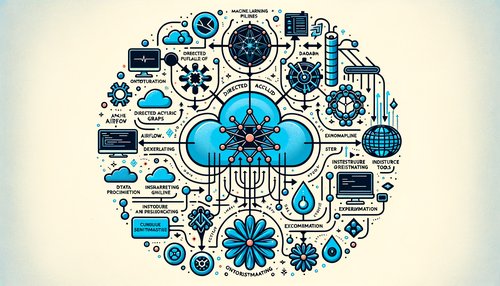
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow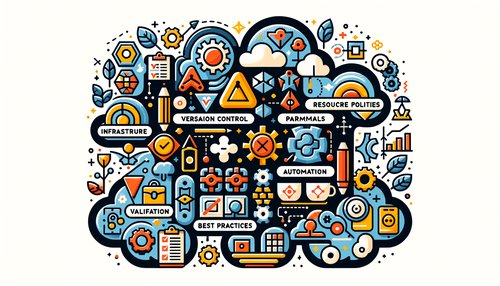
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
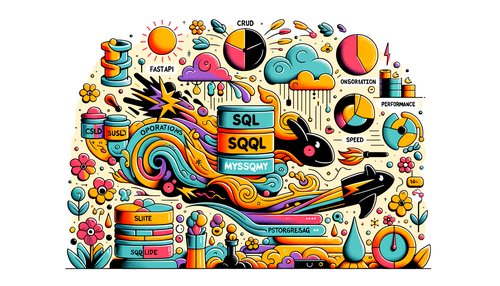