Unveiling the Secrets of Data Manipulation: Mastering Pandas IO Tools for Text, CSV, and HDF5 Files
In the vast ocean of data analysis, the ability to efficiently manipulate and process data files is akin to possessing a magical compass that guides you to the treasure of insights hidden within your data. Among the most powerful tools in a data scientist's arsenal for such tasks is the Pandas library in Python. This blog post embarks on a journey to explore the depths of data manipulation using Pandas, with a specific focus on its IO (Input/Output) capabilities for handling text, CSV, and HDF5 files. Whether you are a seasoned data analyst or a budding data enthusiast, mastering these tools can significantly enhance your data processing skills. So, buckle up as we dive into the secrets of data manipulation and uncover the prowess of Pandas IO tools.
Understanding Pandas IO Tools
Before we delve into the specifics, it's crucial to grasp what Pandas IO tools are. Pandas provides a robust set of IO capabilities designed to read and write a wide range of data formats, including but not limited to text, CSV, and HDF5. These tools are highly optimized for performance and offer a level of abstraction that simplifies complex data manipulation tasks. By leveraging these tools, data practitioners can effortlessly import data from various sources into Pandas DataFrames, perform complex manipulations, and export the processed data to a format of their choice.
Working with Text Files
Text files are one of the simplest and most common data storage formats. Pandas' read_csv
function, despite its name, is incredibly versatile and can be used to read not only CSV files but also delimited text files. Here's a simple example:
import pandas as pd
# Reading a text file
df = pd.read_csv('example.txt', sep='\\t') # Assuming a tab-separated values file
print(df)
This function is highly customizable, with parameters to specify delimiters, column names, data types, and even handling of missing values. For writing data back to a text file, the to_csv
method can be used, which also allows specifying delimiters, among other options.
Mastering CSV Files
CSV (Comma-Separated Values) files are ubiquitous in data science due to their simplicity and ease of use. Pandas shines in handling CSV files, offering both flexibility and efficiency. The read_csv
function is your go-to tool for importing CSV data, providing a plethora of parameters to deal with common issues like header manipulation, date parsing, and chunk loading for large files. Here's how you can use it:
import pandas as pd
# Reading a CSV file
df = pd.read_csv('data.csv')
print(df)
Exporting data to a CSV file is just as straightforward with the to_csv
method, making data exchange between applications seamless.
Exploring HDF5 Files with Pandas
HDF5 stands for Hierarchical Data Format version 5, which is designed to store and organize large amounts of data. It's particularly useful for handling complex data collections and supporting high volumes of data. Pandas provides support for HDF5 through the high-level HDFStore
class, allowing efficient read and write operations. Here's a basic example:
import pandas as pd
# Creating an HDF5 store
store = pd.HDFStore('data.h5')
# Writing data to the store
store['df'] = pd.DataFrame({'A': [1, 2, 3]})
# Reading data from the store
df = store['df']
print(df)
# Closing the store
store.close()
When working with HDF5, it's essential to manage your data's organization and structure carefully, as it can significantly impact performance and scalability.
Summary
In this blog post, we've embarked on a journey through the capabilities of Pandas IO tools for text, CSV, and HDF5 files. We've seen how these tools can simplify data manipulation tasks, making it easier to import, process, and export data in various formats. By mastering these tools, you can significantly enhance your data analysis workflow, making it more efficient and versatile.
As we conclude, remember that the power of Pandas is not just in its functionality but in its ability to transform raw data into meaningful insights. I encourage you to explore these tools further, experiment with different parameters and options, and discover the best practices that suit your data manipulation needs. Happy data wrangling!
Recent Posts
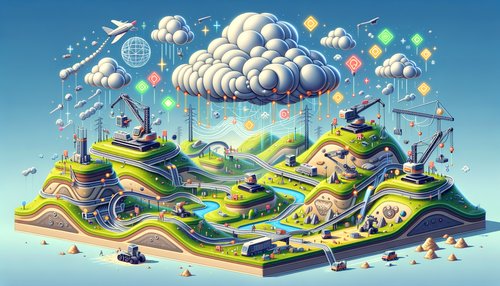
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
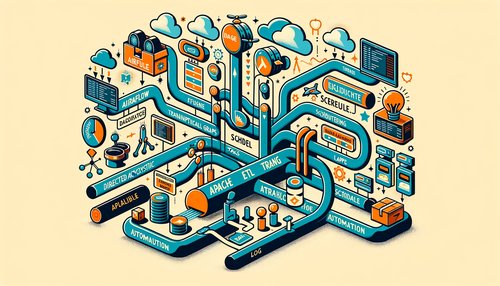
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow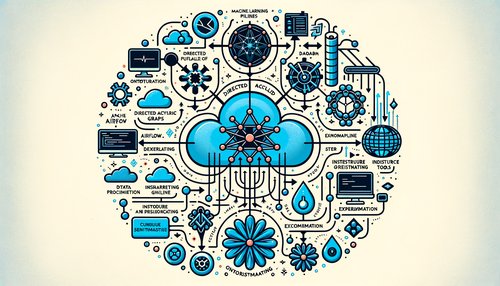
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow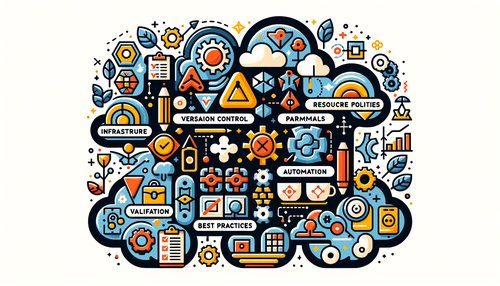
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
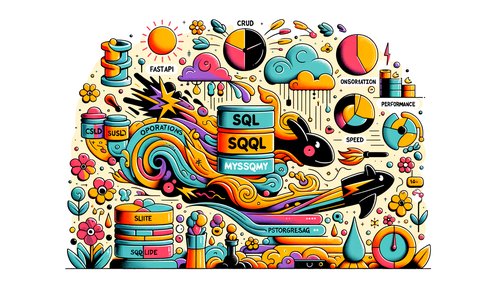