Using Django Class-Based-Views to Streamline Your Web Development Workflow
Are you a web developer looking for a way to streamline your workflow? Django Class-Based-Views (CBVs) can help. CBVs are a powerful tool in Django for quickly and easily creating web applications. They provide an abstraction layer between the model and view layers of an application, allowing you to focus on the functionality of your application without worrying about the underlying details.
Using Django CBVs can help you quickly and easily create powerful web applications. By leveraging the power of the Django framework, you can create applications with minimal effort and time. The Django framework provides a robust set of features to help developers create web applications quickly and efficiently.
Using Django CBVs, you can easily create views that handle HTTP requests, such as GET and POST. These views can be used to display data from the database, handle form submissions, and more. You can also create custom view classes that can be used to extend the functionality of your application.
For example, let’s say you have a simple web application that displays a list of products. Using Django CBVs, you can create a view class that handles the HTTP request and retrieves the data from the database. The view class can then be used to render the data in HTML.
class ProductListView(ListView):
model = Product
template_name = 'products.html'
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
context['products'] = Product.objects.all()
return context
The code above creates a view class that retrieves the list of products from the database and renders it in an HTML template. This view class can then be used to display the list of products in any page of the web application.
Using Django CBVs is an easy and efficient way to create powerful web applications. By leveraging the power of the Django framework, you can quickly and easily create powerful web applications without having to worry about the underlying details. With Django CBVs, you can streamline your web development workflow and create applications quickly and efficiently.
Recent Posts
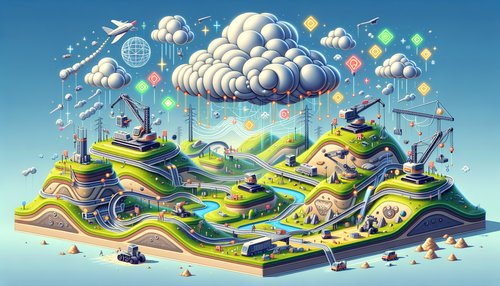
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
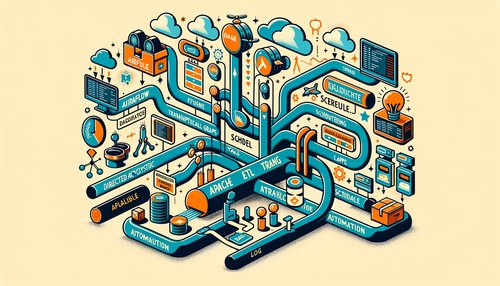
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow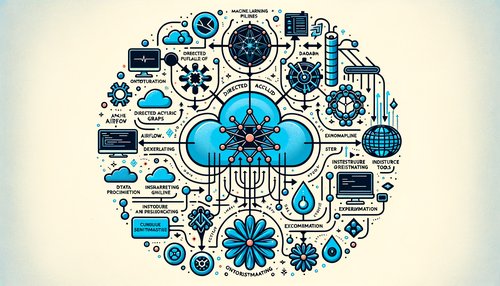
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow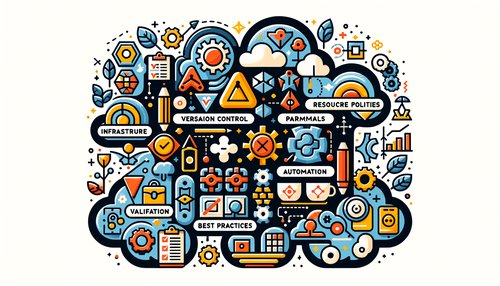
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
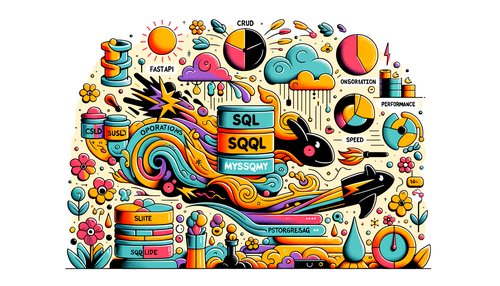