How to Set Up Django Receivers for Maximum Efficiency
Django receivers are a powerful feature that can be used to extend the functionality of your Django application. They allow you to create custom signals that are sent when certain events occur in your application, and can be used to trigger custom actions. In this blog post, we'll take a look at how to set up Django receivers for maximum efficiency.
What are Django Receivers?
Django receivers are a way of extending the functionality of your Django application. They are functions that are triggered when certain events occur in your application, such as when a model instance is saved. These receivers can then be used to perform custom actions, such as sending an email or updating a database table.
Setting Up Django Receivers
Setting up Django receivers is a straightforward process. First, you'll need to define the receiver function. This function should take two arguments: the sender and the instance. The sender is the model class that triggered the receiver, and the instance is the instance of that model class that triggered the receiver. Here's an example of a receiver function:
def my_receiver(sender, instance): # Do something with the sender and instance
Next, you'll need to connect the receiver to a signal. To do this, you'll use the @receiver
decorator. The decorator takes two arguments: the signal and the receiver function. Here's an example of connecting a receiver to the post_save
signal:
@receiver(post_save, sender=MyModel) def my_receiver(sender, instance): # Do something with the sender and instance
Once you have defined and connected your receiver, you'll need to register it with Django. To do this, you'll need to add the following line to your urls.py
file:
url(r'^my_receiver/$', my_receiver, name="my_receiver")
This will register the receiver with Django, and it will be triggered whenever the post_save
signal is sent.
Optimizing Django Receivers
Once your receivers are set up, there are a few things you can do to optimize them for maximum efficiency. First, you should add checks to your receivers to make sure they are only triggered when necessary. For example, you can add a check to make sure that the receiver is only triggered when the instance is saved, not when it is created. This will ensure that the receiver is not triggered unnecessarily.
You should also consider adding caching to your receivers. Caching will help reduce the number of requests made to your receiver, as the data will be stored in memory. This can significantly improve the performance of your application.
Finally, you should consider using a task queue to handle tasks that take a long time to process. This will ensure that your receiver is not blocked while waiting for a long-running task to complete.
Conclusion
Django receivers are a powerful feature that can be used to extend the functionality of your application. Setting up receivers is a straightforward process, and can be optimized for maximum efficiency by adding checks, caching, and using a task queue. With the right setup, receivers can be an invaluable tool for improving the performance and usability of your application.
Recent Posts
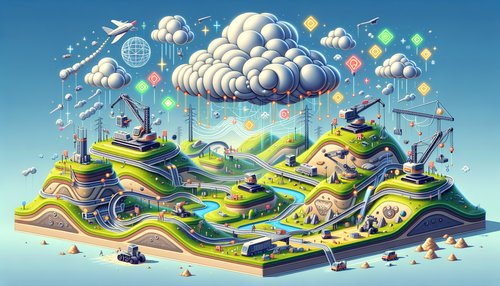
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
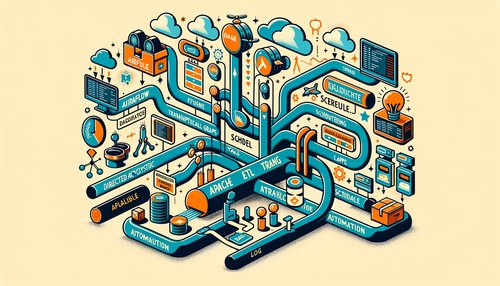
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow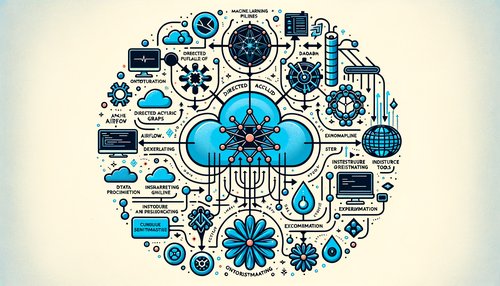
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow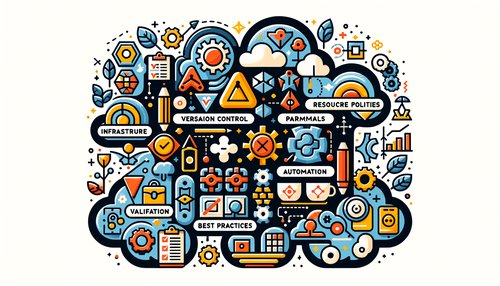
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
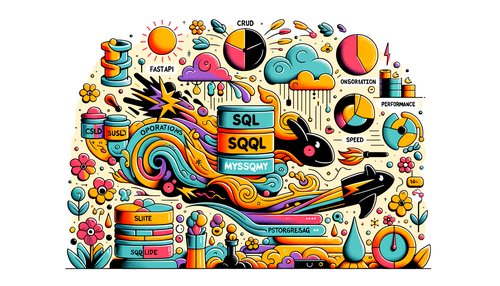