How to Create a Powerful Django REST API in 10 Simple Steps
Creating a powerful Django REST API can be a daunting task, but with the right tools and a few simple steps, it can be achieved with ease. In this blog post, we’ll explore how to build a powerful Django REST API in 10 simple steps.
Step 1: Set Up Your Environment
Before you can start building your API, you’ll need to set up your development environment. This includes installing Python, Django, and the Django REST framework.
Once you have these installed, you’ll need to create a project directory and set up your virtual environment. You can do this with the following commands:
mkdir my_project
cd my_project
python -m venv my_venv
source my_venv/bin/activate
pip install django djangorestframework
Step 2: Create the Django Project
Now that you’ve set up your environment, you can create your Django project. To do this, run the following command:
django-admin startproject my_project
This will create the necessary files and directories for your project.
Step 3: Create the Models
Now that you’ve created your project, you’ll need to create the models for your API. To do this, create a new file called models.py
in the my_project
directory and add the following code:
from django.db import models
class MyModel(models.Model):
name = models.CharField(max_length=255)
description = models.TextField()
This will create a model called MyModel
with two fields: name
and description
.
Step 4: Create the Serializers
Now that your models are created, you’ll need to create the serializers for your API. To do this, create a new file called serializers.py
in the my_project
directory and add the following code:
from rest_framework import serializers
from .models import MyModel
class MyModelSerializer(serializers.ModelSerializer):
class Meta:
model = MyModel
fields = ('name', 'description')
This will create a serializer for the MyModel
model.
Step 5: Create the Views
Now that your models and serializers are created, you’ll need to create the views for your API. To do this, create a new file called views.py
in the my_project
directory and add the following code:
from rest_framework import viewsets
from .models import MyModel
from .serializers import MyModelSerializer
class MyModelViewSet(viewsets.ModelViewSet):
queryset = MyModel.objects.all()
serializer_class = MyModelSerializer
This will create a viewset for the MyModel
model.
Step 6: Create the URLs
Now that you’ve created your views, you’ll need to create the URLs for your API. To do this, create a new file called urls.py
in the my_project
directory and add the following code:
from django.urls import path
from .views import MyModelViewSet
urlpatterns = [
path('my_model/', MyModelViewSet.as_view({'get': 'list'})),
]
This will create a URL for the MyModel
viewset.
Step 7: Add the URLs to the Project
Now that you’ve created the URLs for your API, you’ll need to add them to the project. To do this, open the my_project/urls.py
file and add the following code:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('api/', include('my_project.urls')),
]
This will add the URLs for your API to the project.
Step 8: Migrate the Database
Now that you’ve added the URLs to the project, you’ll need to migrate the database. To do this, run the following commands:
python manage.py makemigrations
python manage.py migrate
This will create the necessary tables in the database for your API.
Step 9: Run the Server
Now that your database is migrated, you can run the server. To do this, run the following command:
python manage.py runserver
This will start the server and you’ll be able to access your API at http://localhost:8000/api/my_model/
.
Step 10: Test the API
Now that your server is running, you can test the API. To do this, you can use a tool like Postman to send requests to the API and view the responses.
Creating a powerful Django REST API can be a daunting task, but with the right tools and a few simple steps, it can be achieved with ease. In this blog post, we’ve explored how to build a powerful Django REST API in 10 simple steps.
Recent Posts
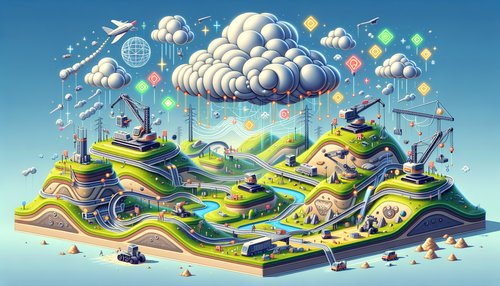
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
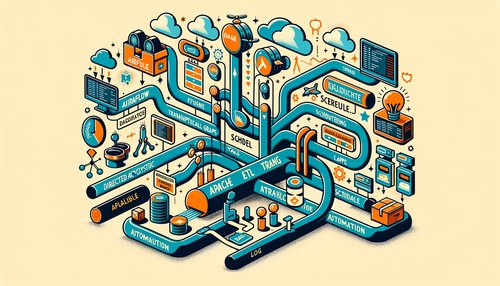
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow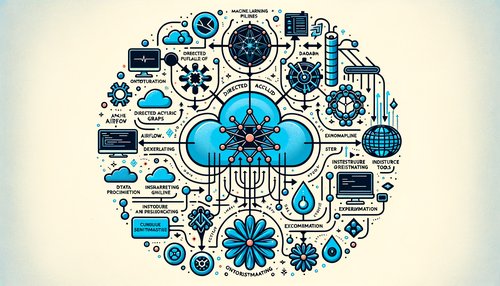
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow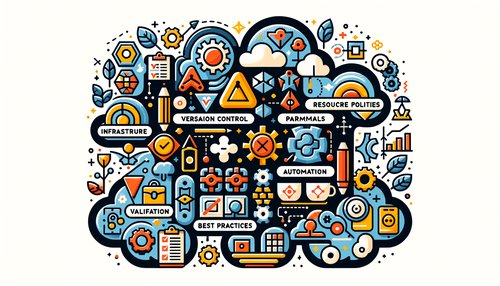
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
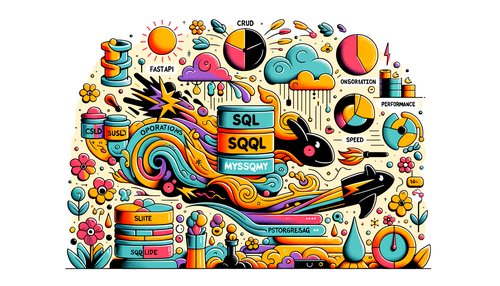