A Guide to Understanding and Implementing Angular Pipes for Maximum Efficiency
Angular pipes are a powerful tool for transforming data in an Angular application. In this guide, we'll be taking a look at what pipes are, how they work, and how to implement them for maximum efficiency.
What are Angular Pipes?
Angular pipes are a feature of the Angular framework that allow developers to transform data within the application. Pipes are a type of function that take an input and return a modified version of that input. For example, a pipe could take a string of text and convert it to all uppercase letters.
Pipes are an easy way to format output data in a consistent manner, and are often used for displaying data in the view layer of an application.
How Do Angular Pipes Work?
Angular pipes are simple functions that take an input and return a modified version of that input. The syntax for a pipe is | <pipeName>
followed by any parameters the pipe might need.
When Angular encounters a pipe in the view layer, it will take the input data and pass it through the pipe, returning the modified data. The modified data is then rendered in the view layer.
Implementing Angular Pipes
Implementing Angular pipes is a simple process. First, create a new pipe in the app.module.ts
file. This is where all of the application's pipes are registered. The syntax for a pipe is:
@Pipe({
name: '<pipeName>'
})
export class <PipeName>Pipe implements PipeTransform {
transform(value: any, args?: any): any {
// modify and return value here
}
}
Next, add the pipe to the view layer where it will be used. The syntax for using a pipe is {{ data | pipeName:parameter }}
. The data will be passed through the pipe, modified, and then rendered in the view.
Conclusion
Angular pipes are a powerful tool for transforming data in an application. By understanding how Angular pipes work and how to implement them, you can use them to maximize the efficiency of your application.
Recent Posts
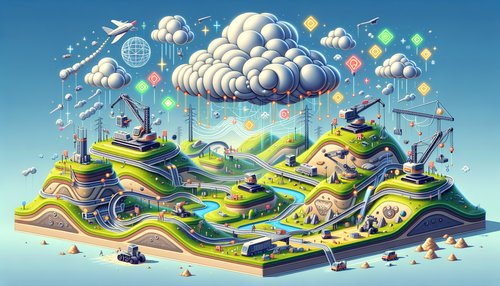
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
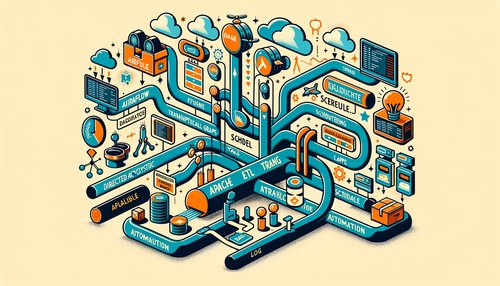
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow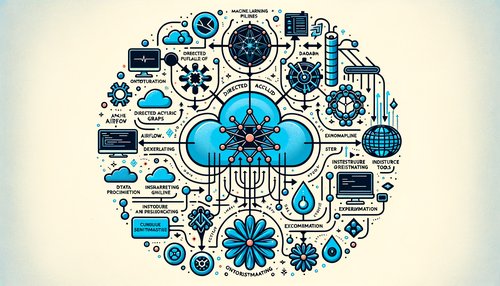
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow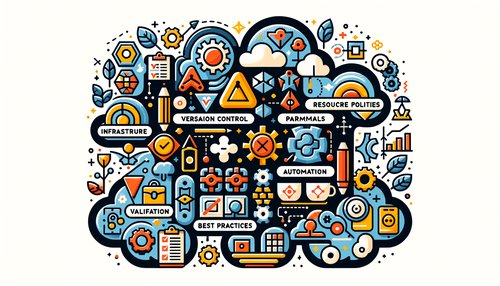
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
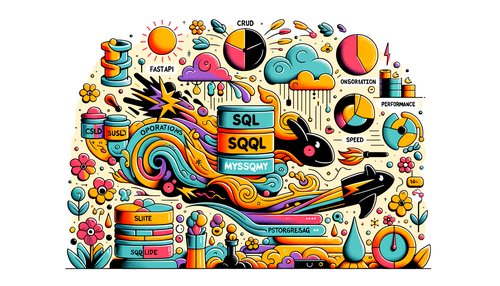