An Introduction to Django Framework Models
Django is an open source web framework that is built on the Python programming language. It is a powerful tool for developing complex web applications quickly and with minimal effort. One of the most important aspects of Django is its model layer. Models are the foundation of any Django application, and they provide a powerful way to interact with data and create complex relationships between different pieces of data. In this blog post, we'll take a look at what models are and how they work in Django.
What is a Model?
A model in Django is a class that is used to define the structure of data in a database. Models are used to define the tables and fields in a database, as well as the relationships between the different pieces of data. Models are also used to query and manipulate data in the database.
Defining a Model
The first step in defining a model is to create a class that inherits from the django.db.models.Model
class. The class will then have a number of fields that define the structure of the data in the database. For example, if we wanted to define a model for a blog post, we would create a class like this:
from django.db import models
class Post(models.Model):
title = models.CharField(max_length=100)
content = models.TextField()
date_published = models.DateTimeField()
This example defines a model with three fields: a title
field that is a character field with a maximum length of 100 characters, a content
field that is a text field, and a date_published
field that is a date/time field.
Working with Models
Once a model is defined, it can be used to query and manipulate data in the database. For example, we can create a new post in the database like this:
post = Post(title='My First Post', content='This is my first post', date_published=datetime.now())
post.save()
We can also query the database for existing posts like this:
posts = Post.objects.all()
This will return all of the posts in the database.
Conclusion
Django models are a powerful tool for working with data in a database. They provide a way to define the structure of the data, as well as a way to query and manipulate it. With models, it is easy to create complex relationships between different pieces of data. If you're working on a web application in Django, models are a must.
Recent Posts
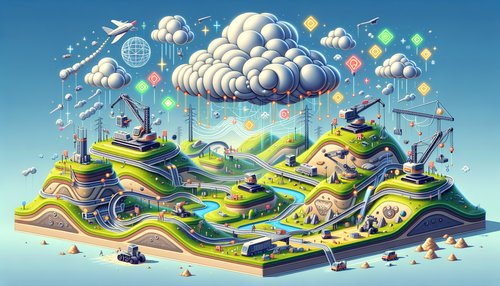
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
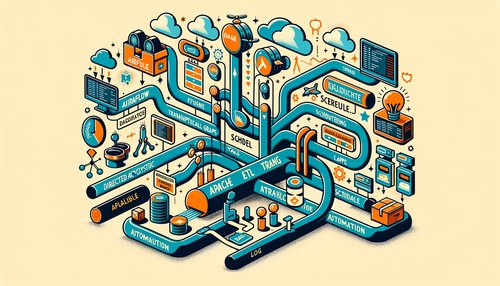
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow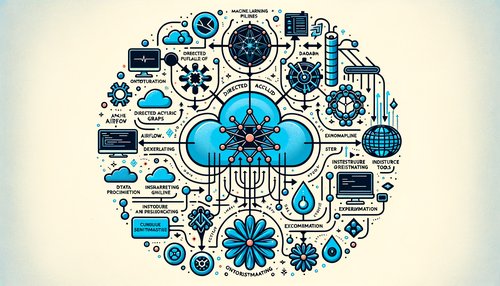
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow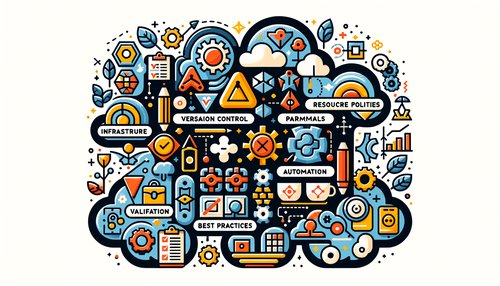
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
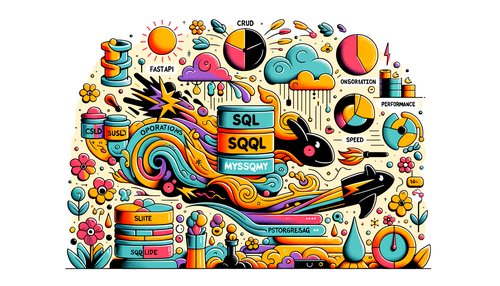