Mastering FastAPI: How to Scale Your Project with a Multi-File Structure - The Ultimate User Guide!
Welcome to the definitive guide on scaling your FastAPI projects! As your application grows, managing your project's complexity becomes paramount. A monolithic file structure, though simple at the start, quickly becomes a bottleneck. This guide is designed to take you through the journey of transforming your FastAPI project into a scalable, maintainable, and efficient system using a multi-file structure. Whether you're a beginner or an experienced developer, the insights and practical tips shared here will elevate your FastAPI projects to the next level.
Understanding FastAPI's Flexibility
FastAPI is a modern, fast (high-performance) web framework for building APIs with Python 3.7+ based on standard Python type hints. The key feature of FastAPI is its speed and ease of use. However, what makes FastAPI truly shine is its flexibility in structuring projects. As your project grows, so does the need for a more organized and scalable structure. This guide will explore how to leverage FastAPI's flexibility to your advantage.
Why Opt for a Multi-File Structure?
Before diving into the how, let's understand the why. A multi-file structure helps in several ways:
- Maintainability: Smaller, logical units of code are easier to manage, understand, and debug.
- Scalability: As features are added, a structured approach allows for easier scaling of the project.
- Collaboration: Teams can work on different parts of the project simultaneously without significant merge conflicts.
Embracing a multi-file structure early in your project can save countless hours of refactoring down the line.
Starting with a Multi-File Structure
The transition to a multi-file structure begins with organizing your FastAPI application into smaller, logical components. Here's a basic structure to start with:
project_directory/ │ ├── app/ │ ├── __init__.py │ ├── main.py # your FastAPI application instance │ ├── dependencies.py # dependency injection │ ├── routers/ │ │ ├── __init__.py │ │ ├── items.py │ │ ├── users.py │ └── models/ │ ├── __init__.py │ ├── item.py │ ├── user.py ├── tests/ │ ├── __init__.py │ ├── test_main.py │ ├── test_items.py │ └── test_users.py ├── .env # environment variables ├── requirements.txt # project dependencies
This structure separates your application into distinct components, making it easier to navigate and scale.
Implementing Routers and Dependencies
In FastAPI, routers help you organize your endpoints into logical groups. Each router can be contained in its own file under the routers
directory, making your API endpoints modular and easy to manage. Here's an example of defining a router in routers/items.py
:
from fastapi import APIRouter router = APIRouter() @router.get("/items/") async def read_items(): return [{"name": "Item 1"}, {"name": "Item 2"}]
Dependencies, on the other hand, can be defined in dependencies.py
and imported wherever needed. This centralizes your dependency logic, making it easier to maintain and update.
Models and Schemas
For data models, the models
directory can house your Pydantic models or ORM models, segregating them from your business logic and endpoint definitions. This separation ensures that your data layer is decoupled from the rest of your application, promoting a clean architecture.
Testing Your Scalable Application
With a multi-file structure, testing becomes more straightforward. You can mirror your application structure in your tests
directory, allowing for targeted and comprehensive testing. This structure makes it easier to identify which parts of your application are covered by tests and which need more attention.
Conclusion
Adopting a multi-file structure in your FastAPI projects is a game-changer for scalability, maintainability, and collaboration. By breaking down your project into logical components, you not only make it easier to understand and manage but also set the stage for seamless scaling as your project grows. Start small, think big, and gradually refactor your project towards a multi-file structure. The benefits, as outlined in this guide, are well worth the effort. Happy coding!
Remember, the journey to mastering FastAPI and scaling your projects efficiently is ongoing. Keep exploring, experimenting, and refining your approach. The ultimate goal is to build robust, scalable, and maintainable applications that stand the test of time.
Recent Posts
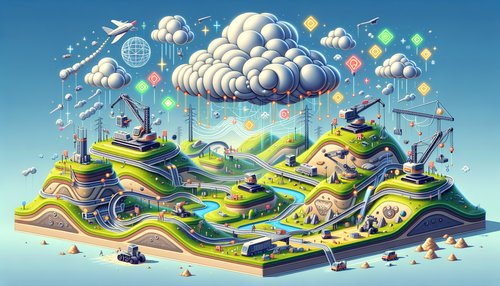
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
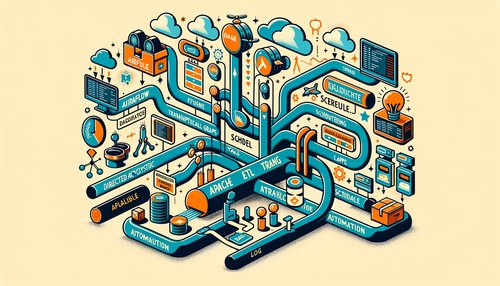
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow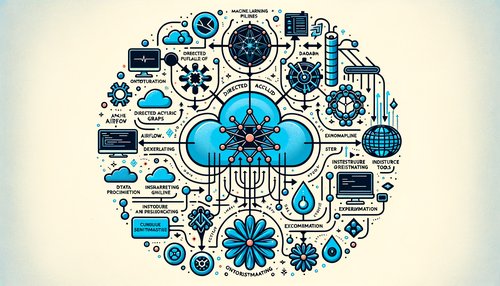
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow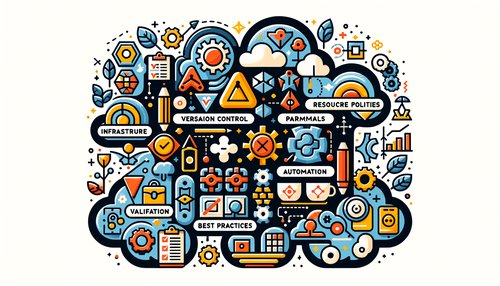
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
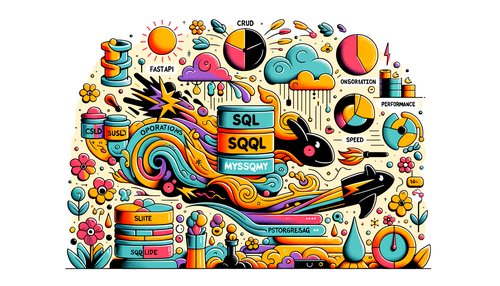