Mastering Data Analysis: How to Unlock the Power of Pandas with Group By's Split-Apply-Combine Strategy
Data analysis is a critical skill in today's data-driven world, and Python's Pandas library is one of the most powerful tools at a data analyst's disposal. One of the key features that make Pandas so invaluable is its group by functionality, which allows for sophisticated aggregation, transformation, and filtration of data using the split-apply-combine strategy. This blog post will guide you through understanding this strategy, how it can be implemented in Pandas, and practical tips to unlock its full potential.
Understanding the Split-Apply-Combine Strategy
The split-apply-combine strategy is a process designed to analyze data by dividing it (split), applying a function (apply), and merging the results into a new dataset (combine). This approach is incredibly versatile, enabling complex data manipulations and analyses in an intuitive and efficient manner.
- Split: The data is divided into subsets based on certain criteria, usually involving one or more key variables.
- Apply: A function is applied to each subset independently. This can be an aggregation, transformation, or filtration operation.
- Combine: The results of the function application are merged into a new dataset, providing a comprehensive view of the analyzed data.
Getting Started with Pandas GroupBy
To leverage the power of the split-apply-combine strategy in Pandas, you'll need to familiarize yourself with the groupby
method. This method allows you to group your data in a DataFrame based on a specified key or keys and then apply aggregation, transformation, or filtration functions to the groups.
Practical Example: Aggregation
Let's say you have a dataset of sales transactions and you want to calculate the total sales per category. Here's how you can do it:
import pandas as pd
# Sample dataset
data = {'Category': ['A', 'B', 'A', 'C', 'B', 'A', 'C', 'A'],
'Sales': [100, 200, 150, 300, 250, 50, 400, 100]}
df = pd.DataFrame(data)
# Group by 'Category' and sum 'Sales'
grouped = df.groupby('Category')['Sales'].sum()
print(grouped)
This simple example demonstrates the aggregation aspect of the apply step, where the sum function is applied to each group of categories.
Advanced Techniques
Transformation
Transformation operations modify the grouped data in a specific way, such as standardizing data within groups. This is particularly useful when you want to normalize or scale your data.
Filtration
Filtration allows you to discard data based on the group properties. For instance, you might want to filter out groups that do not meet a certain threshold, such as groups with a total sales value less than a specified amount.
Best Practices and Tips
- Understand your data: Before applying the group by operations, it's crucial to have a good understanding of your dataset. This includes knowing the data types, the meaning of each column, and what you're trying to achieve with your analysis.
- Use meaningful aggregations: Choose aggregation functions that make sense for your data and your analysis goals. For example, summing up sales figures might be useful, but averaging them could be misleading if the data is skewed.
- Optimize performance: Large datasets can slow down group by operations significantly. Consider filtering your data or selecting a subset of columns before applying group by operations to improve performance.
Conclusion
The group by functionality in Pandas, powered by the split-apply-combine strategy, is a potent tool for data analysis. By mastering this approach, you can unlock insights into your data that would be difficult or impossible to obtain otherwise. Remember to start with a clear understanding of your dataset and analysis goals, and don't be afraid to experiment with different aggregation, transformation, and filtration techniques. With practice, you'll find that the group by functionality becomes an invaluable part of your data analysis toolkit.
As you continue to explore data analysis with Pandas, consider diving deeper into other features of the library and how they can complement your use of the group by functionality. Happy analyzing!
Recent Posts
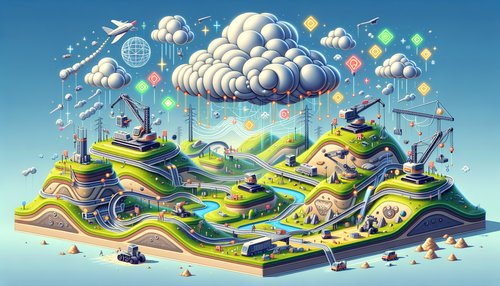
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
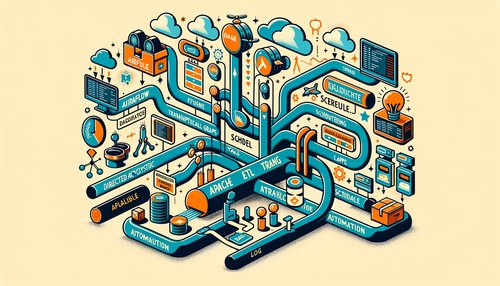
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow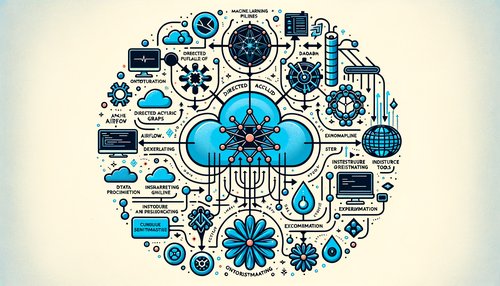
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow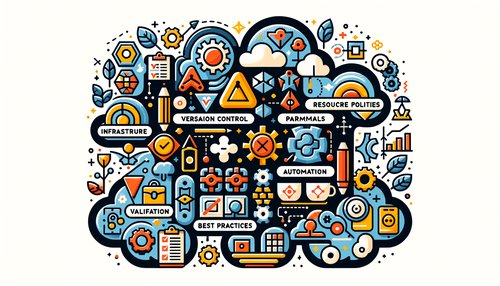
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
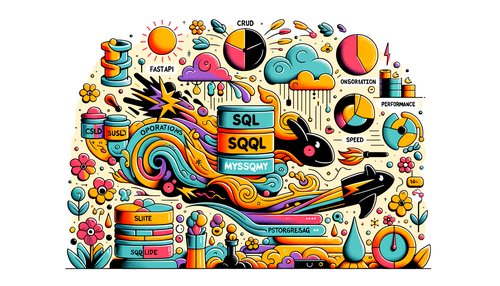