Unlocking the Power of Django Function-Based Views: Unlocking New Possibilities in Application Development
For many developers, the power of Django is clear. It’s a powerful web framework that makes it easy to quickly develop complex applications. But how can developers unlock even more of the potential of Django? One way is through the use of function-based views.
Function-based views are a powerful way to create views for your Django applications. Unlike class-based views, function-based views allow you to write your own custom view logic without relying on the Django framework. This means you can create views tailored to your own unique requirements. You can also create views that are more efficient and simpler to maintain.
One of the advantages of function-based views is that they allow you to keep your code DRY (Don't Repeat Yourself). By creating a single view function and reusing it for multiple views, you can avoid having to rewrite the same code over and over again. This makes your code easier to read and maintain.
Function-based views also give you more control over how your application responds to requests. Instead of relying on the Django framework to handle requests, you can write your own custom logic to handle them. This means you can create views that are tailored to your application’s specific needs.
Finally, function-based views are great for creating APIs. You can write a single view function that handles requests from multiple sources, such as web browsers or mobile applications. This allows you to easily create an API that can be used by multiple clients.
To get started with function-based views, you’ll need to create a view function. This is done by using the @view_function
decorator. This decorator will tell Django that the function is a view function and should be used when handling requests.
from django.http import HttpResponse from django.views.decorators.http import view_function @view_function def my_view(request): return HttpResponse('Hello, World!')
Once you’ve created your view function, you can then use it in your URL configurations. This will tell Django to use your view function when handling requests for that URL. For example, if you wanted to use your view function for all requests to /hello
, you could add the following to your URL configuration:
urlpatterns = [ path('hello/', my_view, name='hello'), ]
Using function-based views is a great way to unlock the power of Django and take your application development to the next level. With function-based views, you can create views tailored to your application’s needs, keep your code DRY, and create powerful APIs. Get started today and see what you can build!
Recent Posts
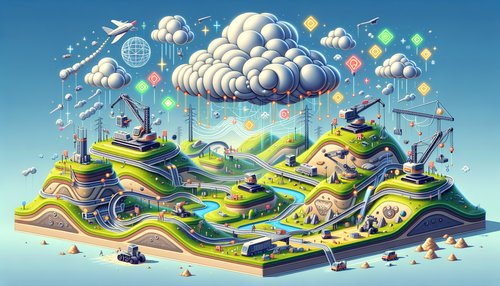
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
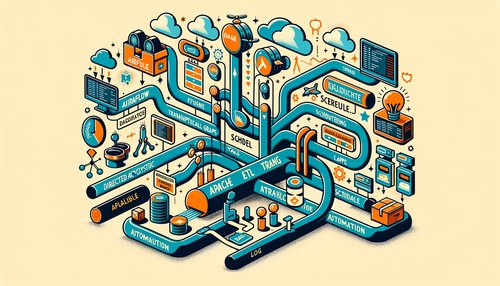
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow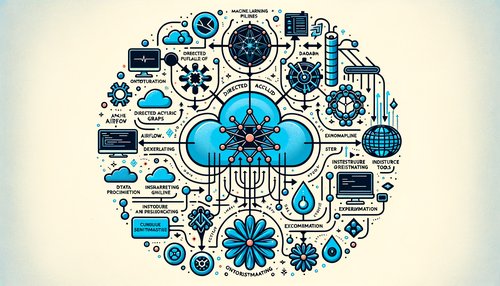
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow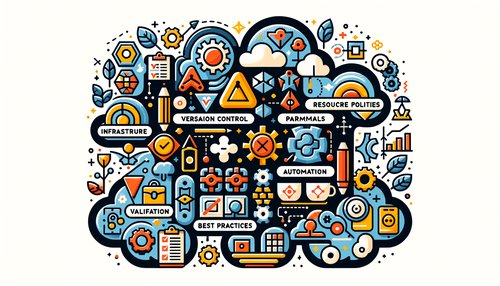
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
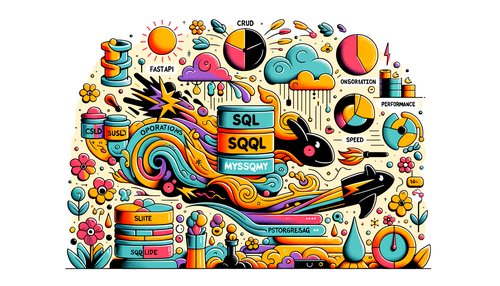