Unlocking the Power of Django Rest Framework Serializers for Maximum Efficiency
Django Rest Framework (DRF) is a powerful open source library for building RESTful APIs. It provides an intuitive interface for creating robust and secure web applications. One of its most useful features is the ability to create custom serializers to handle complex data structures. Serializers are an essential tool for managing data in DRF, allowing developers to easily convert complex data into a format that can be used in an API.
In this blog post, we'll explore how to unlock the power of DRF serializers and use them to maximum efficiency. We'll take a look at how to create custom serializers, how to use nested serializers, and how to manage complex data structures.
Creating Custom Serializers
Creating custom serializers in DRF is easy. All you need to do is create a class that extends the DRF Serializer class and define the fields you want to serialize. For example, if we wanted to serialize a list of products, we could create a ProductSerializer class like this:
class ProductSerializer(serializers.Serializer):
name = serializers.CharField()
price = serializers.FloatField()
description = serializers.CharField()
This serializer will serialize a list of products with the name, price, and description fields. We can also add additional fields to the serializer if needed.
Using Nested Serializers
Nested serializers allow us to serialize complex data structures such as nested dictionaries and lists. For example, if we wanted to serialize a list of products with each product containing a list of images, we could create a nested serializer like this:
class ProductSerializer(serializers.Serializer):
name = serializers.CharField()
price = serializers.FloatField()
description = serializers.CharField()
images = ImageSerializer(many=True)
class ImageSerializer(serializers.Serializer):
url = serializers.URLField()
This serializer will serialize a list of products with each product containing a list of images. This is a powerful way to manage complex data structures.
Managing Complex Data Structures
When working with complex data structures, it's important to be able to manage them efficiently. DRF provides a number of useful tools for managing complex data structures, such as the ModelSerializer class, which allows us to easily serialize Model instances. We can also use the SerializerMethodField to define custom serialization methods for complex data structures.
For example, if we wanted to serialize a list of products with each product containing a list of images, we could create a ModelSerializer like this:
class ProductSerializer(serializers.ModelSerializer):
images = serializers.SerializerMethodField()
def get_images(self, product):
return ImageSerializer(product.images, many=True).data
This serializer will serialize a list of products with each product containing a list of images. This is a powerful and efficient way to manage complex data structures.
Conclusion
Django Rest Framework serializers are a powerful and flexible tool for managing complex data structures. They allow us to easily convert complex data into a format that can be used in an API. By understanding how to create custom serializers, use nested serializers, and manage complex data structures, we can unlock the power of DRF serializers and use them to maximum efficiency.
Recent Posts
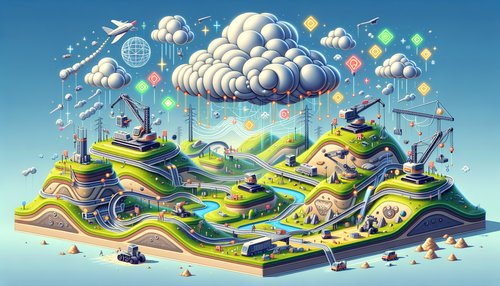
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
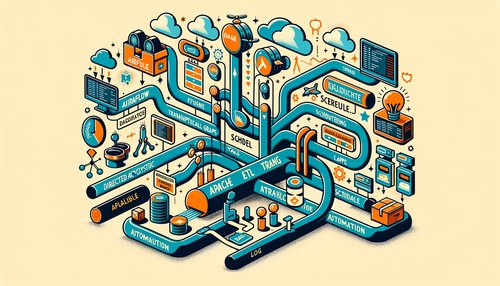
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow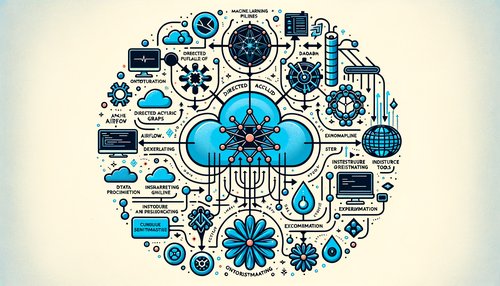
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow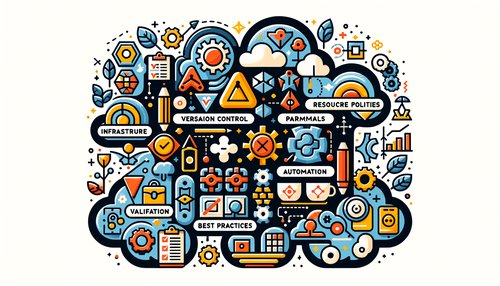
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
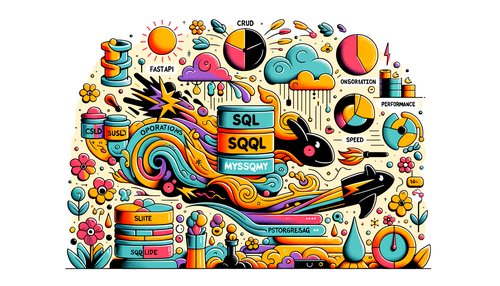