Unlock the Power of Django with REST APIs: A Comprehensive Guide
Django is a powerful web framework for Python that makes it easy to create complex web applications quickly and efficiently. With the help of Django REST framework, it's possible to unlock the full potential of Django and create powerful, secure, and easy-to-use REST APIs.
In this comprehensive guide, we'll explore the basics of Django REST framework and its advanced features. We'll also look at how to create a full-featured REST API using Django REST framework, including code snippets in Markdown format.
What is Django REST framework?
Django REST framework is a high-level Python library that makes it easy to create REST APIs with Django. It provides a full range of features, including authentication, serialization, routing, and more.
Setting up Django REST framework
To get started, you'll need to install Django REST framework. You can do this using the pip package manager:
pip install djangorestframework
Once Django REST framework is installed, you'll need to add it to your INSTALLED_APPS
setting in your settings.py
file:
INSTALLED_APPS = [
...
'rest_framework',
]
Creating a REST API with Django REST framework
Now that Django REST framework is set up, we can start creating our API. We'll start by creating a new Django app. We can do this by running the following command in the terminal:
python manage.py startapp api
Once our Django app is created, we'll need to add it to our INSTALLED_APPS
setting in our settings.py
file:
INSTALLED_APPS = [
...
'api',
]
Next, we'll need to create our API views. We can do this by creating a views.py
file in our api
app, and adding the following code:
from rest_framework.views import APIView
class APIView(APIView):
def get(self, request):
return Response("Hello, World!")
Finally, we'll need to create our API URLs. We can do this by creating a urls.py
file in our api
app, and adding the following code:
from django.urls import path
from .views import APIView
urlpatterns = [
path('', APIView.as_view(), name="api-view")
]
Conclusion
By following the steps outlined in this guide, you should now have a full-featured REST API built with Django REST framework. You can now start building powerful and secure APIs with Django.
If you have any questions or comments, please feel free to leave them in the comments section below.
Recent Posts
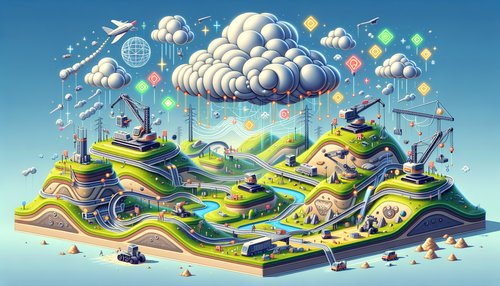
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
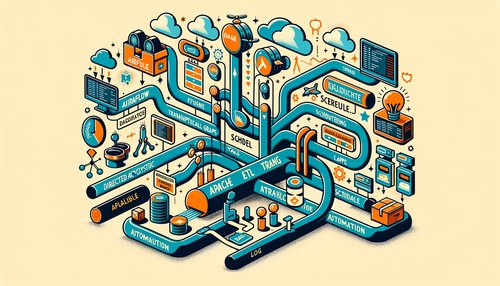
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow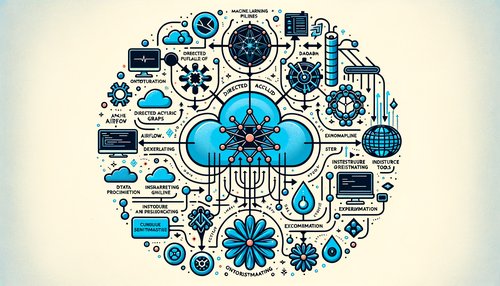
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow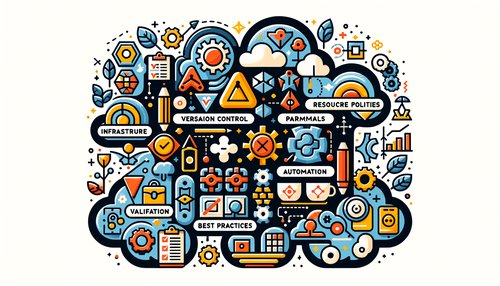
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
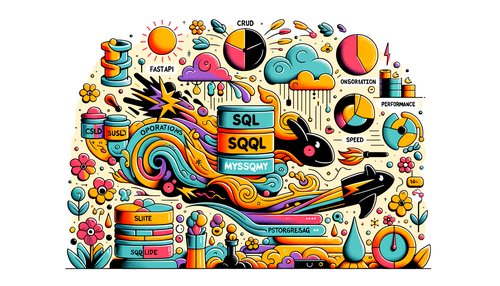