Unlocking FastAPI's Security Features: A Simplified Guide to Implementing OAuth2 with Password and Bearer Authentication
In the rapidly evolving world of web development, securing your application is more crucial than ever. FastAPI, a modern, fast (high-performance) web framework for building APIs with Python 3.7+ based on standard Python type hints, offers robust security features that are both powerful and easy to implement. Among these, OAuth2 with Password (and hashing), plus Bearer with JWT tokens, stands out as a particularly effective method for safeguarding your application. This guide aims to demystify the process of integrating these security mechanisms into your FastAPI application, ensuring that your data remains secure and your APIs are protected against unauthorized access.
Understanding OAuth2 and Bearer Authentication
Before diving into the implementation, it's essential to grasp the concepts of OAuth2 and Bearer Authentication. OAuth2 is an authorization framework that enables applications to obtain limited access to user accounts on an HTTP service. It works by delegating user authentication to the service that hosts the user account and authorizing third-party applications to access the user account. OAuth2 uses "tokens" granted by the authorization server, eliminating the need for the application to store user credentials.
Bearer Authentication, on the other hand, is a method of sending a token in a request's Authorization header. This token acts as a "bearer" credential, allowing access to a protected resource without requiring the application to reveal the identity of the user or device.
Setting Up Your FastAPI Application
First, ensure that you have FastAPI and Uvicorn (an ASGI server) installed. If not, you can install them using pip:
pip install fastapi uvicorn
Create a new Python file for your application and import FastAPI:
from fastapi import FastAPI
app = FastAPI()
Integrating OAuth2 with Password and Bearer Authentication
Implementing OAuth2 in FastAPI requires setting up a security scheme. FastAPI provides several security utilities, including OAuth2PasswordBearer, a class that you can use to define how the OAuth2 security scheme will operate.
from fastapi import Depends, FastAPI, HTTPException, status
from fastapi.security import OAuth2PasswordBearer
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
The tokenUrl
parameter is the URL to receive the token. This URL will be where your users will send their username and password to get the token.
Creating a Token Generation Endpoint
To generate tokens, you need to create an endpoint. This involves importing and using security-related functions from FastAPI:
from fastapi import Depends, FastAPI, HTTPException, status
from fastapi.security import OAuth2PasswordRequestForm
from jose import JWTError, jwt
from datetime import datetime, timedelta
# Your secret key
SECRET_KEY = "a very secretive secret key"
ALGORITHM = "HS256"
ACCESS_TOKEN_EXPIRE_MINUTES = 30
@app.post("/token")
async def login_for_access_token(form_data: OAuth2PasswordRequestForm = Depends()):
user = authenticate_user(fake_users_db, form_data.username, form_data.password)
if not user:
raise HTTPException(
status_code=status.HTTP_401_UNAUTHORIZED,
detail="Incorrect username or password",
headers={"WWW-Authenticate": "Bearer"},
)
access_token_expires = timedelta(minutes=ACCESS_TOKEN_EXPIRE_MINUTES)
access_token = create_access_token(
data={"sub": user.username}, expires_delta=access_token_expires
)
return {"access_token": access_token, "token_type": "bearer"}
This endpoint will authenticate users and return a JWT token. You'll need to implement the authenticate_user
and create_access_token
functions based on your authentication backend.
Protecting Routes with OAuth2
To protect a route, you use the Depends
function with the OAuth2 scheme you've defined:
@app.get("/users/me")
async def read_users_me(token: str = Depends(oauth2_scheme)):
return {"token": token}
This example shows a simple route that returns the token of the authenticated user. In practice, you'd use the token to retrieve and verify the user's information.
Conclusion
FastAPI's security features offer a robust and straightforward way to implement OAuth2 with Password and Bearer Authentication in your applications. By following this guide, you've learned how to set up an OAuth2 security scheme, generate tokens, and protect routes in your FastAPI application. With these capabilities, you can ensure that your APIs are secure and that only authorized users can access sensitive information.
As you continue to develop your FastAPI applications, keep exploring the framework's extensive documentation and community resources to further enhance your application's security and functionality.
Recent Posts
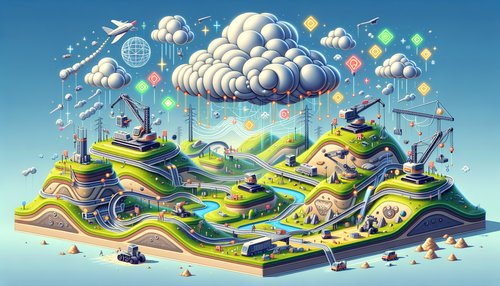
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
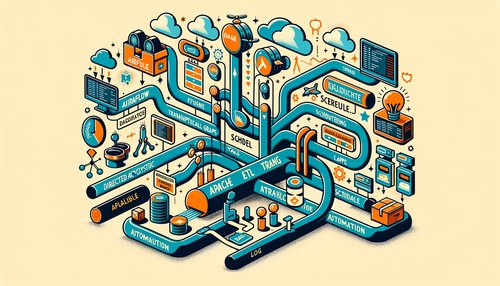
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow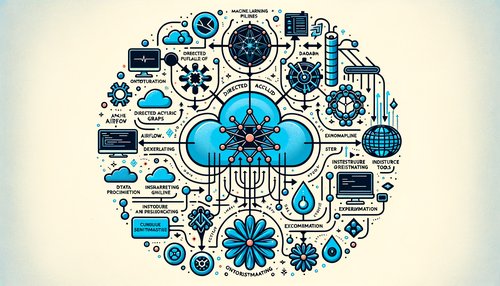
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow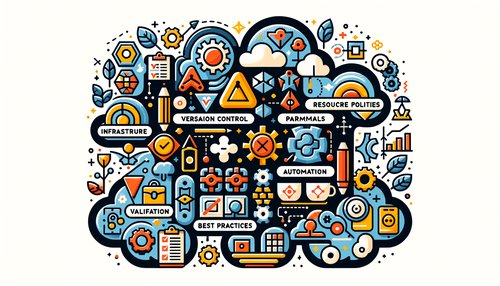
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
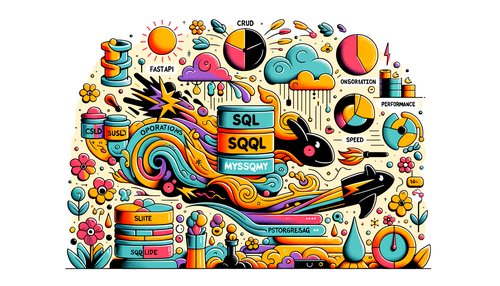