Getting Started With FastAPI
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.6+ based on standard Python type hints. It has been gaining a lot of popularity lately due to its ease of use and scalability.
If you are looking for a modern, easy-to-use and high-performance web framework for building APIs with Python, then FastAPI is the right choice for you. In this blog post, we will discuss how to get started with FastAPI and some of its key features.
Installation
The first step to get started with FastAPI is to install it. You can install FastAPI using pip
:
pip install fastapi
Once it is installed, you can import it into your project:
from fastapi import FastAPI
Creating an App
The next step is to create an app with FastAPI. You can do this by creating an instance of the FastAPI
class:
app = FastAPI()
Adding Routes
Once your app is created, you can start adding routes to it. Routes are used to map URLs to functions or classes that handle requests. To add a route, you can use the @app.get
decorator:
@app.get("/")
def home():
return {"message": "Hello, world!"}
This route will map the URL /
to the home()
function which will return a JSON response with the message "Hello, world!".
Running the App
Once you have added all the routes, you can run the app by calling the run()
method:
app.run()
This will start the development server and your app will be available at http://127.0.0.1:8000
.
Conclusion
In this blog post, we discussed how to get started with FastAPI and some of its key features. FastAPI is a modern, fast and easy-to-use web framework for building APIs with Python. It is gaining a lot of popularity due to its scalability and ease of use.
If you are looking for a modern web framework for building APIs with Python, then FastAPI is definitely worth checking out.
Recent Posts
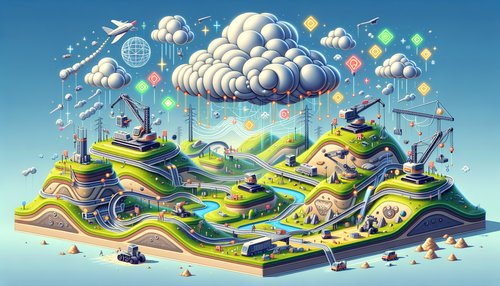
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
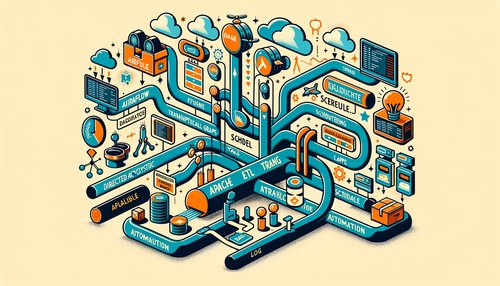
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow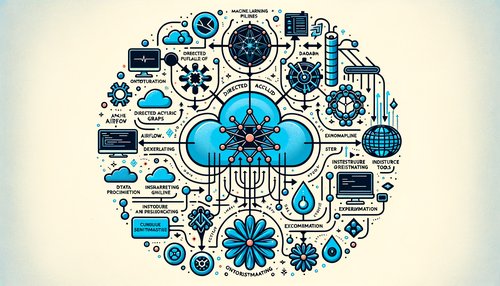
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow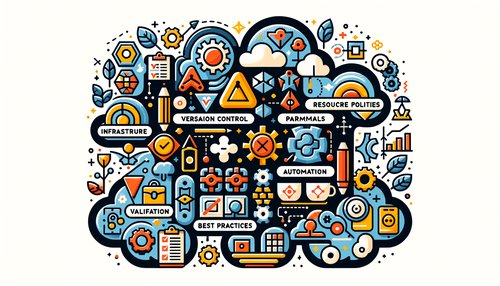
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
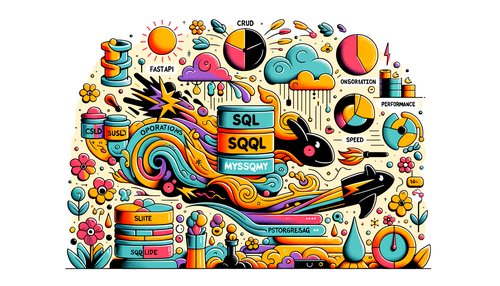