How to Use React Hooks with React 17
React 16 introduced the new Hooks API, which allows developers to use state and other React features without writing a class. This is a great way to write React components in a more concise and organized way. In this blog post, we'll look at how to use React Hooks with React 17.
Using the useState Hook
The first Hook we'll look at is the useState
Hook, which allows you to add state to a functional component. To use the useState
Hook, you need to import it from the react
package:
import { useState } from 'react';
Once imported, you can use the useState
Hook in your component like this:
const [count, setCount] = useState(0);
The useState
Hook takes an initial value as an argument, and returns an array with two elements: the current state and a function to update the state. In this example, we're initializing the state to 0, and the function we get back is called setCount
. We can use this function to update the state:
setCount(count + 1);
This will increment the count
state by 1.
Using the useEffect Hook
The useEffect
Hook allows you to perform side effects in a functional component. This is great for setting up subscriptions, making API calls, and updating the DOM in response to changes in state. To use the useEffect
Hook, you need to import it from the react
package:
import { useEffect } from 'react';
Once imported, you can use the useEffect
Hook in your component like this:
useEffect(() => {
// Code to run when the component is rendered
});
The useEffect
Hook takes a function as an argument, and this function will be called whenever the component is rendered. This is useful for setting up subscriptions, making API calls, and updating the DOM in response to changes in state.
Conclusion
React Hooks are a great way to write React components in a more concise and organized way. In this blog post, we looked at how to use the useState
and useEffect
Hooks with React 17. With these two Hooks, you can easily add state and perform side effects in your functional components.
Recent Posts
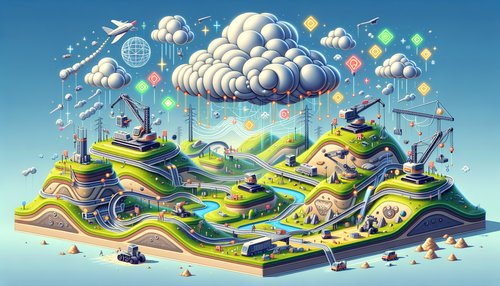
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
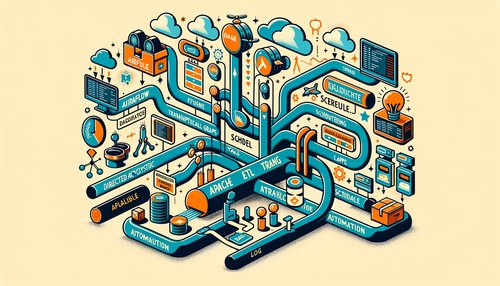
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow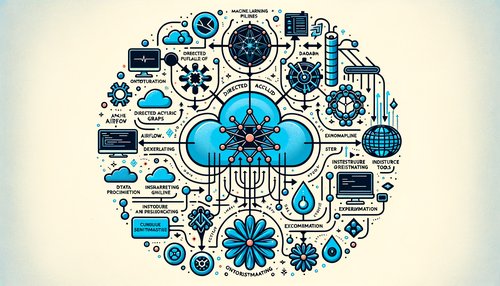
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow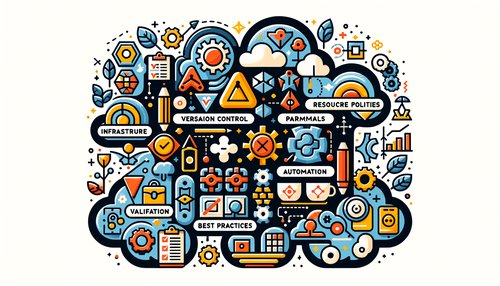
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
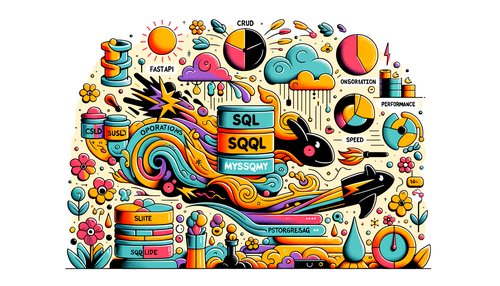