Mastering FastAPI: A Comprehensive Guide to SQL (Relational) Database Integration
In an age where web application performance and scalability are paramount, FastAPI emerges as a standout choice for developers. Known for its speed and ease of use, FastAPI is a modern web framework that has quickly gained popularity for building APIs. However, a powerful API relies heavily on seamless integration with a database. This blog post delves into integrating SQL (relational) databases with FastAPI, ensuring you can leverage the best of both worlds in your projects.
Choosing the Right SQL Database
Before diving into integration, it's crucial to choose the right SQL database that meets your application's needs. Options like PostgreSQL, MySQL, and SQLite each have their pros and cons. PostgreSQL is known for its advanced features and performance, making it ideal for complex applications. MySQL is renowned for its speed and reliability, favored in many legacy applications. SQLite offers simplicity and is suitable for small applications or prototyping.
Consider factors such as transaction support, scalability, and community support when choosing a database. Regardless of your choice, FastAPI's flexibility allows smooth integration with any of these databases.
Setting Up FastAPI with SQLAlchemy
SQLAlchemy is a popular ORM (Object-Relational Mapper) that allows Python developers to interact with databases in a pythonic way. Setting up SQLAlchemy with FastAPI involves a few steps:
- Install Dependencies: Start by installing FastAPI, SQLAlchemy, and a database driver, such as aiopg for PostgreSQL or PyMySQL for MySQL.
pip install fastapi sqlalchemy psycopg2-binary
- Define your Database Models: Create Python classes that represent your database tables. Use SQLAlchemy's `Base` to define your models.
from sqlalchemy import Column, Integer, String, create_engine from sqlalchemy.ext.declarative import declarative_base Base = declarative_base() class User(Base): __tablename__ = 'users' id = Column(Integer, primary_key=True, index=True) name = Column(String, index=True)
- Create a database session: Setup your database engine and session to enable communication with the database.
from sqlalchemy.orm import sessionmaker database_url = "postgresql://user:password@localhost/dbname" engine = create_engine(database_url) SessionLocal = sessionmaker(autocommit=False, autoflush=False, bind=engine)
Implementing CRUD Operations
The core of any database interaction is CRUD: Create, Read, Update, and Delete. Implementing these operations in FastAPI with SQLAlchemy is straightforward.
Consider a simple FastAPI route to create a new user:
from fastapi import FastAPI, Depends
from sqlalchemy.orm import Session
from . import models, schemas, database
app = FastAPI()
def get_db():
db = database.SessionLocal()
try:
yield db
finally:
db.close()
@app.post("/users/", response_model=schemas.User)
async def create_user(user: schemas.UserCreate, db: Session = Depends(get_db)):
db_user = models.User(name=user.name)
db.add(db_user)
db.commit()
db.refresh(db_user)
return db_user
By leveraging SQLAlchemy's session, we manage transactions confidently and can easily extend the application with additional CRUD operations.
Optimizing Database Performance
Integration is nothing without performance. Here are some tips to optimize your SQL database with FastAPI:
- Indexing: Ensure appropriate indexing on frequently queried columns to speed up read operations.
- Connection Pooling: Utilize SQLAlchemy's pooling features to manage database connections efficiently.
- Async Operations: FastAPI supports async operations, which can be combined with async ORMs to handle high workloads.
- Monitoring and Profiling: Continuously monitor performance metrics and profile queries to find and fix bottlenecks.
Conclusion
Integrating SQL databases with FastAPI equips developers with the tools necessary to build robust, scalable APIs. By choosing the right database, setting up SQLAlchemy, implementing essential CRUD operations, and optimizing database performance, you ensure your application is ready to meet modern-day demands.
Now armed with the knowledge and practical examples provided, it's time to implement these skills in your projects. Dive into FastAPI, unleash its potential with SQL databases, and craft reliable backend systems.
Recent Posts
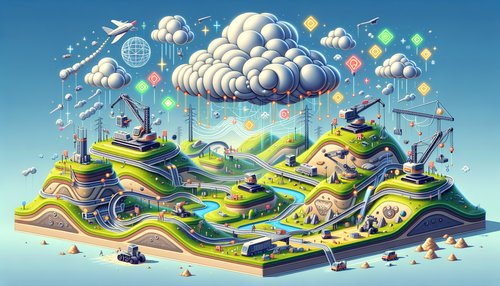
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
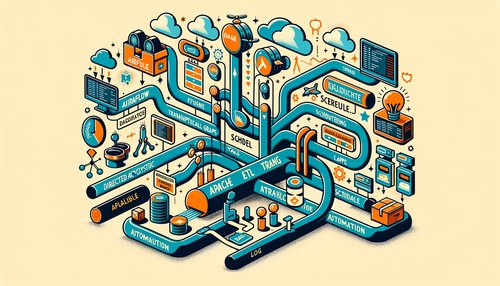
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow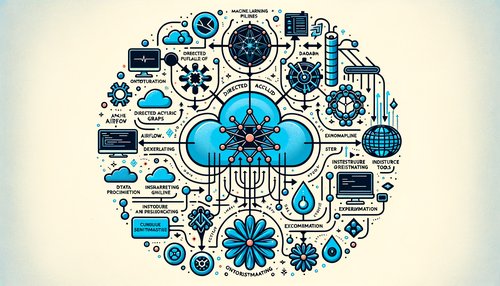
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow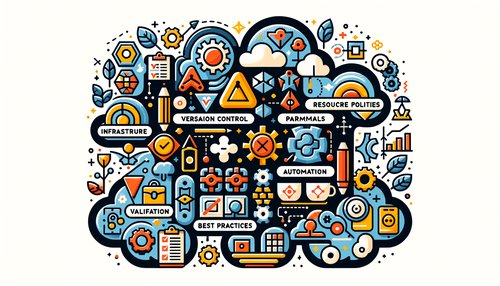
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
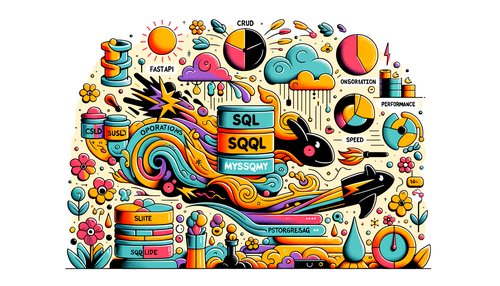