Mastering FastAPI: A Comprehensive User Guide for Scaling Bigger Applications Across Multiple Files
FastAPI has been gaining traction as one of the most efficient and easy-to-learn web frameworks for Python. Its promise of quick development and high performance makes it ideal for small projects. But what about larger applications that require scaling and modularization? In this post, we'll dive deep into how to master FastAPI for scaling bigger applications across multiple files. We will cover structuring your project, organizing routes and dependencies, and practical tips for seamless scalability.
Why FastAPI?
Before jumping into the details of scaling FastAPI applications, it's essential to understand why FastAPI is a good choice:
- Speed: Built on Starlette for the web parts and Pydantic for data parts, FastAPI is designed for fast performance.
- Type Safety: With automatic type checking and robust data validation, you can write cleaner and more error-free code.
- Documentation: FastAPI automatically generates interactive API documentation.
- Asynchronous Support: Native support for asynchronous programming allows you to handle many requests per second.
Structuring Your Project
One of the key aspects of maintaining scalable applications is proper project structure. When your project grows, a monolithic single-file structure can become cumbersome.
Here's a basic template for structuring a larger FastAPI project:
project/ │ ├── app/ │ ├── __init__.py │ ├── main.py │ ├── api/ │ │ ├── __init__.py │ │ ├── routes/ │ │ │ ├── __init__.py │ │ │ ├── users.py │ │ │ ├── items.py │ ├── core/ │ │ ├── __init__.py │ │ ├── config.py │ │ ├── security.py │ ├── models/ │ │ ├── __init__.py │ │ ├── user.py │ │ ├── item.py └── tests/
Organizing Routes
Using multiple files for routes can help keep your code clean and organized. Each route can be defined in its own module, then imported into a central routing module.
For example, in api/routes/users.py
:
from fastapi import APIRouter, Depends router = APIRouter() @router.get("/users/{user_id}") def get_user(user_id: int): return {"user_id": user_id}
Then in main.py
:
from fastapi import FastAPI from app.api.routes import users, items app = FastAPI() app.include_router(users.router) app.include_router(items.router)
Dependencies and Dependency Injection
FastAPI’s dependency injection system allows you to manage dependencies in a clean and efficient manner. Use dependencies to manage database connections, authentication, and other shared resources.
For example, a common database dependency could be defined as:
from sqlalchemy.orm import Session from app.database import SessionLocal async def get_db(): db = SessionLocal() try: yield db finally: db.close()
Usage in a route:
from fastapi import Depends from sqlalchemy.orm import Session @router.get("/items/{item_id}") def get_item(item_id: int, db: Session = Depends(get_db)): # use `db` here return {"item_id": item_id}
Practical Tips
For making your large-scale FastAPI application more manageable, consider the following tips:
- Use Environment Variables: Store configurations and secrets in environment variables to keep your codebase clean and secure.
- Logging: Implement a robust logging mechanism to help monitor and debug the application effectively.
- Testing: Use automated tests to ensure your application’s functionality at scale.
- Documentation: Maintain good documentation, not just auto-generated API docs, but also developer guides and setup instructions.
Conclusion
Scaling a FastAPI application across multiple files may seem daunting initially, but with proper organization and use of FastAPI’s robust features like dependency injection, the process becomes much smoother. Start with a solid project structure, modularize routes, and efficiently manage dependencies to make your application robust and maintainable. By taking these steps, you can ensure that your FastAPI project is ready to scale and handle increased complexity and traffic.
Now that you are well-equipped with the essentials, it's time to roll up your sleeves and start building your scalable FastAPI application. Happy coding!
Recent Posts
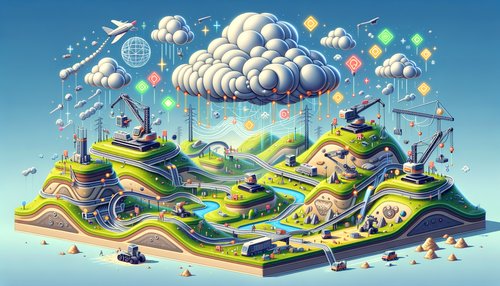
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
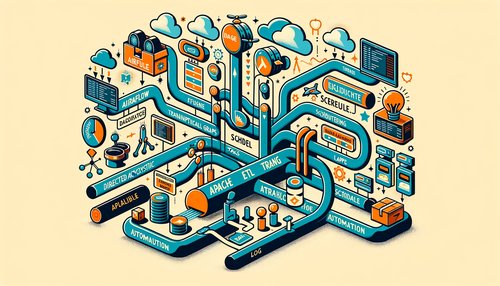
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow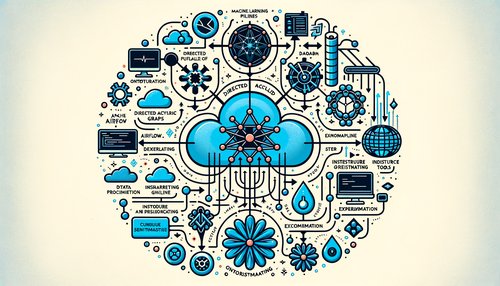
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow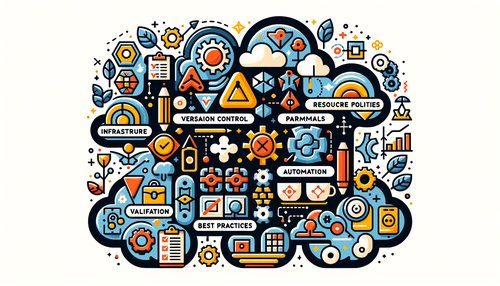
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
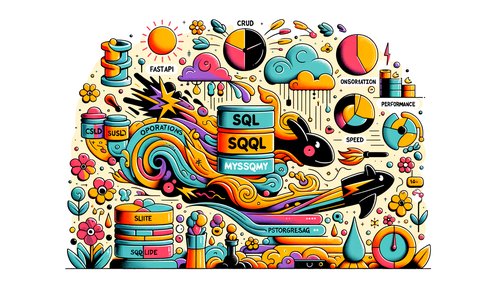