Mastering FastAPI Security: A Comprehensive Guide to OAuth2 with Password, JWT Bearer Tokens, and Hashing
FastAPI is one of the most exciting web frameworks to emerge in recent years. It offers modern, fast, web development capabilities and comes packed with tools that help build robust and secure applications. In this guide, we dive deep into securing FastAPI applications - focusing on OAuth2 with Password, JWT Bearer Tokens, and Hashing. We'll explore these components in detail, empowering you with practical knowledge to implement security in your FastAPI projects.
Understanding OAuth2 and Why It Matters
OAuth2 is an industry-standard protocol for authorization. It allows third-party services to exchange your information without exposing your credentials. In FastAPI, integrating OAuth2 ensures secure user authentication and authorization flows within your application.
With OAuth2, you can manage permission levels across your application by issuing access tokens. These tokens eliminate the need to pass sensitive user credentials across the network, thus minimizing security vulnerabilities.
Setting Up OAuth2 with Password in FastAPI
FastAPI provides in-built support for managing OAuth2. One of the most common flows in OAuth2 is using a password grant, which is useful for first-party apps. Here's how you can set it up:
from fastapi import FastAPI, Depends, HTTPException
from fastapi.security import OAuth2PasswordBearer
app = FastAPI()
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
@app.post("/token")
async def login(form_data: OAuth2PasswordRequestForm = Depends()):
# Implement your logic to authenticate user here
return {"access_token": "", "token_type": "bearer"}
@app.get("/users/me")
async def read_users_me(token: str = Depends(oauth2_scheme)):
# Decode and validate the token here
return {"user": ""}
This setup gives you a secure endpoint to issue and validate tokens for authenticated users. Testing on a tool like Postman will help you tweak the authentication process to your application needs.
JWT Bearer Tokens: Ensuring Secure Data Transfer
JWTs, or JSON Web Tokens, are essential in building secure FastAPI applications. They encode data into a payload with a header and signature, which can be verified independently from one service to another. FastAPI makes it simple to manage JWTs.
Let’s look at a basic JWT setup:
import jwt
SECRET_KEY = "mysecretkey"
ALGORITHM = "HS256"
# Encode
data = {"sub": "user_id"}
token = jwt.encode(data, SECRET_KEY, algorithm=ALGORITHM)
# Decode
decoded_data = jwt.decode(token, SECRET_KEY, algorithms=[ALGORITHM])
Integrating JWTs with OAuth2 enhances security by ensuring that tokens can be verified without needing centralized authentication. It's crucial to manage keys securely and rotate them periodically to reduce the risk of exposure.
Hashing: A Necessary Security Layer
Password hashing is vital for ensuring user credentials are stored securely. Even if a security breach occurs, hashed passwords are not easily reversible, safeguarding sensitive information.
FastAPI pairs seamlessly with libraries like passlib
to integrate robust hashing algorithms into your authentication workflows:
from passlib.context import CryptContext
pwd_context = CryptContext(schemes=["bcrypt"], deprecated="auto")
# Hash a password
hashed_password = pwd_context.hash("myplaintextpassword")
# Verify a password
is_verified = pwd_context.verify("myplaintextpassword", hashed_password)
Utilizing such libraries allows you to keep up with best practices in password security without reinventing the wheel.
Conclusion: Strengthen Your FastAPI Application
Securing your FastAPI application is not just about implementing OAuth2, JWTs, and hashing; it's about constantly evaluating and improving your security posture. By leveraging these tools, you can offer users a secure, reliable service.
Armed with this comprehensive understanding, take a proactive approach to your application’s security. As a final step, always stay informed about new security practices and continuously update your security layers. Remember, securing your app is a journey, not a destination.
Start implementing these practices today and see how they fortify your FastAPI applications!
Recent Posts
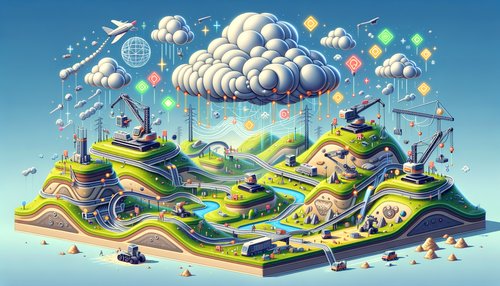
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
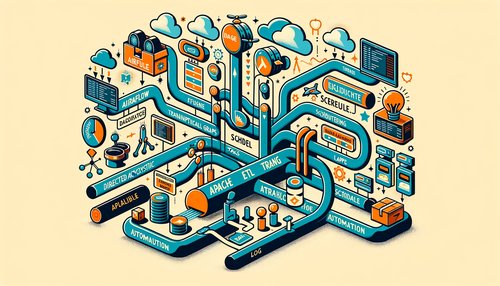
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow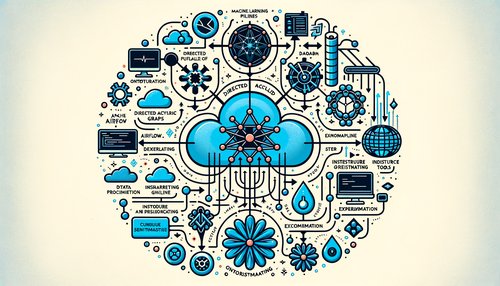
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow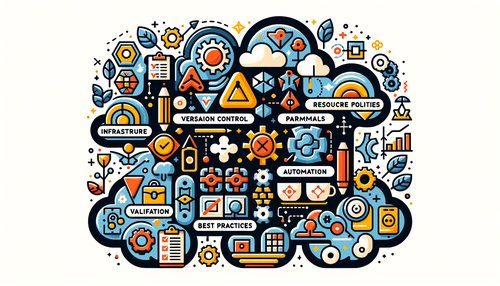
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
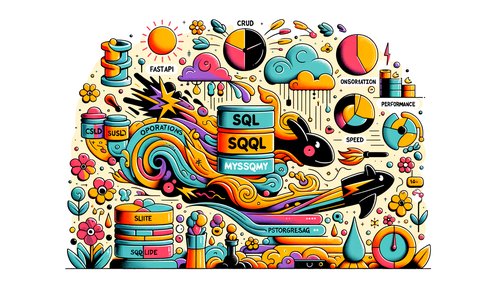