Mastering FastAPI Security: A Comprehensive Guide to OAuth2, Password Hashing, and JWT Bearer Tokens
FastAPI has quickly become a favorite framework for developing APIs due to its high performance and ease of use. However, securing these APIs is critical, especially when handling sensitive information. In this comprehensive guide, we will explore various aspects of FastAPI security, including OAuth2, password hashing, and JWT Bearer Tokens.
Understanding OAuth2 in FastAPI
OAuth2 is an authorization framework that allows applications to obtain limited access to user accounts on an HTTP service. It works by delegating user authentication to the service that hosts the user account and authorizing third-party applications to access the user account.
Setting Up OAuth2
To get started with OAuth2 in FastAPI, you need to install some additional libraries:
pip install fastapi[all]
Next, you can set up the OAuth2 scheme in your FastAPI application:
from fastapi import FastAPI, Depends
from fastapi.security import OAuth2PasswordBearer
app = FastAPI()
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
@app.post("/token")
def login(form_data: OAuth2PasswordRequestForm = Depends()):
return {"access_token": "some_token", "token_type": "bearer"}
Password Hashing
Password hashing is a crucial step in securing user credentials. It ensures that even if your database is compromised, the attackers won't have access to the actual passwords.
Implementing Password Hashing
FastAPI provides utilities to hash and verify passwords using the passlib
library:
pip install passlib[bcrypt]
Here’s how you can use it in your FastAPI application:
from passlib.context import CryptContext
pwd_context = CryptContext(schemes=["bcrypt"], deprecated="auto")
def get_password_hash(password):
return pwd_context.hash(password)
def verify_password(plain_password, hashed_password):
return pwd_context.verify(plain_password, hashed_password)
During user registration, you would hash the password before saving it:
@app.post("/register")
def register_user(user: UserCreate):
hashed_password = get_password_hash(user.password)
# Save hashed_password in the database
Working with JWT Bearer Tokens
JWT (JSON Web Tokens) are a compact, URL-safe means of representing claims to be transferred between two parties. They are often used for authentication and information exchange in a secure manner.
Creating and Verifying JWT Tokens
To handle JWT tokens in FastAPI, you can use the pyjwt
library:
pip install pyjwt
Here's an example of creating and verifying JWT tokens:
import jwt
from datetime import datetime, timedelta
SECRET_KEY = "YOUR_SECRET_KEY"
ALGORITHM = "HS256"
ACCESS_TOKEN_EXPIRE_MINUTES = 30
def create_access_token(data: dict, expires_delta: timedelta = None):
to_encode = data.copy()
if expires_delta:
expire = datetime.utcnow() + expires_delta
else:
expire = datetime.utcnow() + timedelta(minutes=15)
to_encode.update({"exp": expire})
encoded_jwt = jwt.encode(to_encode, SECRET_KEY, algorithm=ALGORITHM)
return encoded_jwt
def verify_token(token: str):
try:
payload = jwt.decode(token, SECRET_KEY, algorithms=[ALGORITHM])
return payload
except jwt.PyJWTError:
return None
Use these methods in your routes where you need to issue or verify tokens:
@app.post("/token")
def login(form_data: OAuth2PasswordRequestForm = Depends()):
user_dict = authenticate_user(form_data.username, form_data.password)
if not user_dict:
raise HTTPException(status_code=400, detail="Invalid credentials")
access_token_expires = timedelta(minutes=ACCESS_TOKEN_EXPIRE_MINUTES)
access_token = create_access_token(data={"sub": user_dict["username"]}, expires_delta=access_token_expires)
return {"access_token": access_token, "token_type": "bearer"}
Conclusion
Securing your FastAPI application is essential to protect user data and ensure trust. By integrating OAuth2, password hashing, and JWT Bearer Tokens, you can enhance the security of your APIs effectively. Remember, security is an ongoing process, so continue to stay informed about the latest practices and updates in the field.
Implement these strategies in your FastAPI projects today and enjoy a more secure application development experience. Happy coding!
Recent Posts
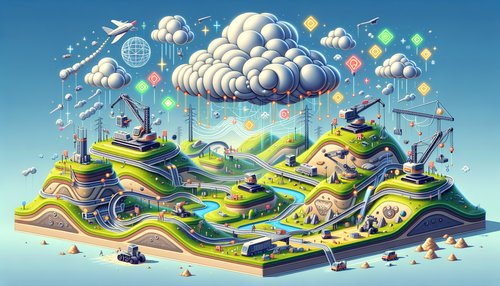
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
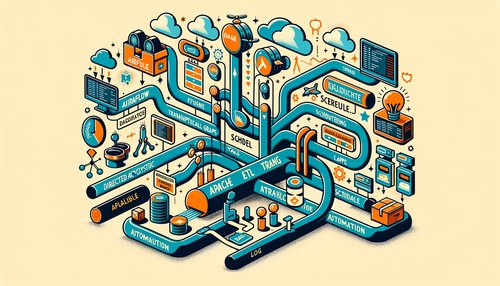
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow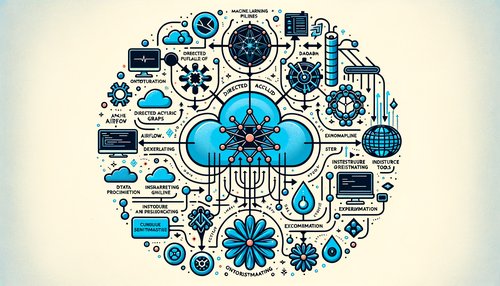
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow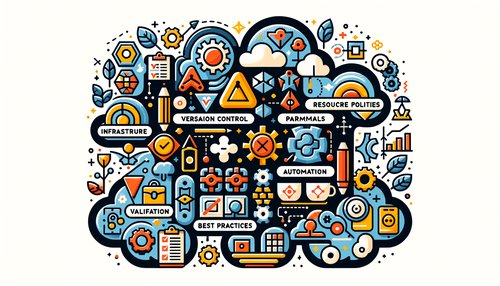
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
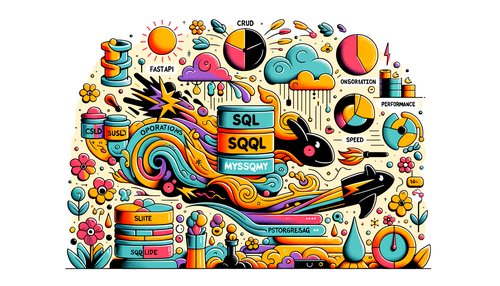