Mastering Infrastructure as Code: The Ultimate Guide to Terraform Modules and Standard Module Structure
In today's rapidly evolving technological landscape, managing infrastructure in a consistent, repeatable, and scalable manner is essential. Terraform, a tool invented by HashiCorp, allows developers to define and provision infrastructure using code. But where Terraform truly shines is in its ability to use modules. In this ultimate guide, we will uncover the significance of Terraform modules, the benefits of using them, and best practices for structuring them efficiently.
Understanding Terraform Modules
Terraform modules are containers for multiple resources that are used together. A module consists of a collection of .tf or .tf.json files kept together in a directory. Modules can be thought of as the basic building blocks that allow you to organize and encapsulate your infrastructure as code.
Why Use Modules?
Using modules offers several advantages:
- Reusable Components: Modules make it easier to reuse code for similar infrastructure components, reducing duplication and errors.
- Simplification: They simplify complex configurations by allowing you to break large configurations into smaller, more manageable pieces.
- Encapsulation: Modules encapsulate implementation details, exposing only the necessary inputs and outputs.
- Maintainability: Modules help in maintaining a clean and organized codebase.
Creating Your First Module
Creating a module is straightforward. Let's walk through an example of creating a basic AWS S3 bucket module:
Step-by-Step Example:
// directory structure
my-module/
├── main.tf
├── variables.tf
└── outputs.tf
// main.tf
resource "aws_s3_bucket" "example" {
bucket = var.bucket_name
}
// variables.tf
variable "bucket_name" {
description = "The name of the S3 bucket"
type = string
}
// outputs.tf
output "bucket_arn" {
description = "The ARN of the bucket"
value = aws_s3_bucket.example.arn
}
In this example, we've created a simple module named my-module
that provisions an AWS S3 bucket. This module takes one input variable bucket_name
and outputs the ARN of the bucket once it's created.
Standard Module Structure
Adopting a standardized module structure is crucial for maintainability and scalability. Here is a recommended structure excerpted from HashiCorp's guidelines:
Typical Module File Layout:
my-module/
├── main.tf # Primary resource configurations
├── variables.tf # Input variables definitions
├── outputs.tf # Output definitions
├── README.md # Documentation
├── versions.tf # Provider and module version constraints
├── locals.tf # Local values
├── modules/ # Internal child modules
├── examples/ # Examples of module usage
└── test/ # Automated tests for the module
Following this structure can significantly improve collaboration and code readability. Each file has a specific purpose, and laying out your module this way helps ensure it remains manageable as it grows.
Best Practices for Working with Modules
To make the most out of your Terraform modules, consider the following best practices:
- Write Clear Documentation: Always document your modules using a README.md file. Include examples and thorough explanations of inputs, outputs, and usage.
- Use Semantic Versioning: Version your modules using SemVer to keep track of changes and ensure backward compatibility.
- Keep Modules Self-Contained: Avoid dependencies on external resources not managed within the module itself.
- Use Meaningful Names: Name your variables, outputs, and purpose in a way that clearly conveys their usage and intent.
- Optimize For Reusability: Design modules in a way that they can be reused across different projects and teams.
Conclusion
Mastering Terraform modules is a significant step forward in the journey towards effective Infrastructure as Code. By organizing your code into reusable modules, you can create more maintainable, scalable, and efficient infrastructure configurations. Remember to adhere to standard structures, follow best practices, and document your modules thoroughly. By doing so, you’ll be well on your way to becoming an infrastructure as code expert. Happy coding!
Ready to get started? Dive into your first module creation and experience the power of Terraform modules today!
Recent Posts
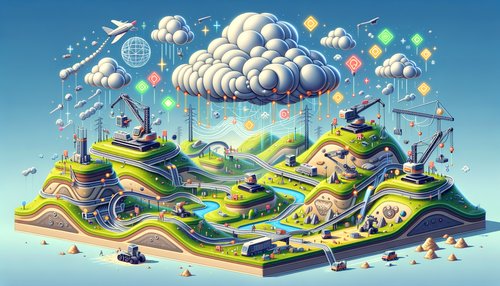
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
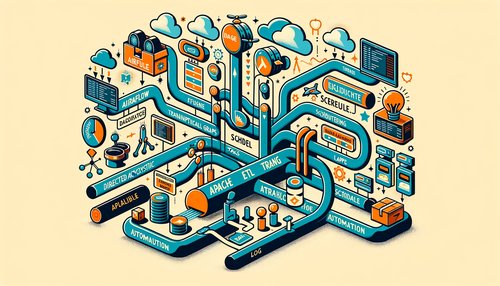
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow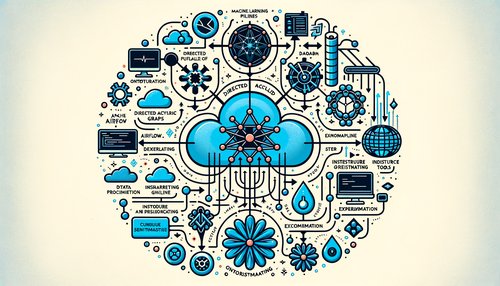
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow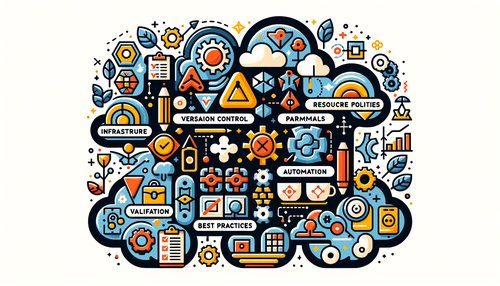
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
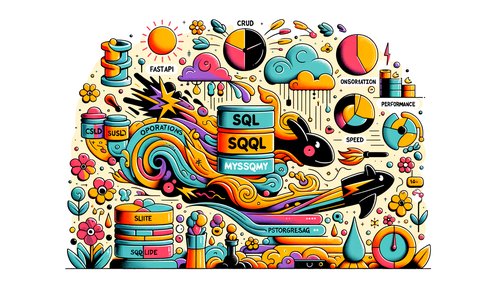