Mastering the Art of Graceful Failures: Your Ultimate Guide to Error Handling in FastAPI
Welcome to the world of FastAPI, where speed meets reliability, and robust applications are the norm. However, even in this high-performance environment, errors are an inevitable part of development. The key to maintaining a seamless user experience lies not in avoiding errors altogether but in handling them gracefully. This blog post will guide you through the art of managing failures elegantly in FastAPI, ensuring your application remains robust, user-friendly, and reliable, no matter what surprises come its way.
Understanding Error Handling in FastAPI
Before diving into the specifics, it's crucial to understand what error handling in FastAPI entails. FastAPI, built on Starlette, provides a straightforward way to deal with errors through exception handlers and status codes. This approach ensures that your API can communicate effectively with clients, even when things go south. We'll explore how to leverage these features to create a resilient application.
Designing Your Error Response Model
The first step in mastering error handling is designing a consistent error response model. This model should convey necessary information to the client, such as the error type, message, and any relevant details. With FastAPI, you can use Pydantic models to define these error structures, ensuring consistency across your API.
from pydantic import BaseModel
class ErrorResponse(BaseModel):
error: str
detail: str
Implementing Global Exception Handlers
FastAPI allows you to define global exception handlers that can catch errors thrown anywhere in your application. This is particularly useful for handling common errors in a DRY (Don't Repeat Yourself) manner. For instance, you can create a global handler for HTTPException
to customize the error response format.
from fastapi import FastAPI, HTTPException
from fastapi.responses import JSONResponse
app = FastAPI()
@app.exception_handler(HTTPException)
async def http_exception_handler(request, exc):
return JSONResponse(
status_code=exc.status_code,
content={"error": "HTTPException", "detail": str(exc.detail)},
)
Customizing Error Handling for Specific Use Cases
While global exception handlers are great for common errors, you might encounter situations requiring more tailored handling. FastAPI's dependency injection system provides a powerful way to deal with such cases elegantly. For example, you can create dependencies that perform error checks and raise exceptions, which are then caught by your handlers.
Logging Errors for Monitoring and Debugging
Proper error logging is crucial for diagnosing and fixing issues quickly. FastAPI does not automatically log exceptions, so you should integrate a logging system into your application. Python's built-in logging
module can be configured to log errors with sufficient detail, helping you monitor your API's health and respond to issues promptly.
Testing Your Error Handling
No error handling strategy is complete without thorough testing. Use FastAPI's TestClient
to simulate requests and verify that your application responds as expected in error scenarios. This ensures your error handling logic works correctly and improves your API's reliability.
Summary
Mastering error handling in FastAPI is about more than just catching exceptions; it's about creating a seamless, reliable experience for your users even when errors occur. By designing a consistent error response model, implementing global and specific exception handlers, logging errors effectively, and rigorously testing your error handling strategies, you can build robust FastAPI applications that stand the test of time. Remember, the goal is not to eliminate errors entirely but to manage them so gracefully that your users barely notice them.
In the journey of application development, embracing errors as opportunities for improvement is the hallmark of a mature developer. So, go forth and make your FastAPI applications not just fast and efficient, but also resilient and user-friendly. Happy coding!
Recent Posts
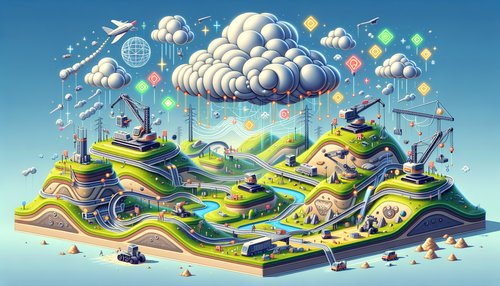
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
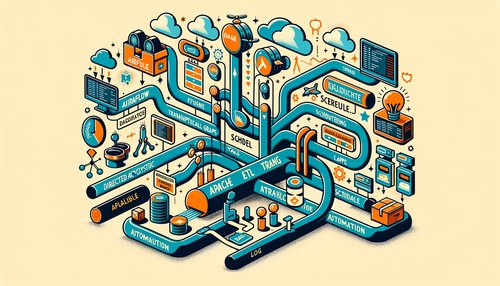
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow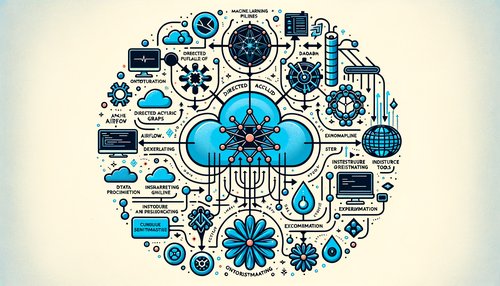
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow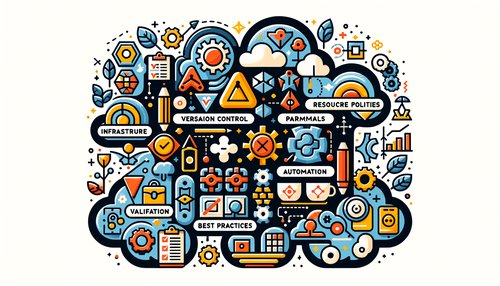
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
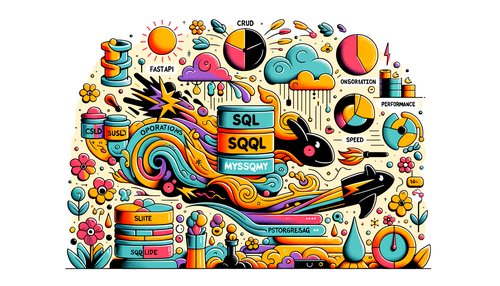