Supercharge Your Angular App with Powerful Pipes!
Angular pipes are a powerful way to transform data within your application. They are a great way to keep your code organized, clean, and easy to read. Pipes are also an easy way to make your app more dynamic and interactive. In this blog post, we’ll explore some powerful pipes you can use to supercharge your Angular app.
Filtering Pipes
One of the most useful pipes are those that filter data. Angular offers a few built-in pipes that make it easy to filter data based on certain criteria. For example, if you want to filter a list of items by a certain value, you can use the filter
pipe. This pipe takes two parameters: the item to filter by and the value to filter on.
items = [
{name: 'Apple', color: 'red'},
{name: 'Banana', color: 'yellow'},
{name: 'Orange', color: 'orange'},
];
filteredItems = this.items | filter: 'color': 'yellow';
// filteredItems = [{name: 'Banana', color: 'yellow'}]
Formatting Pipes
Another type of pipe you can use to supercharge your Angular app is a formatting pipe. These pipes allow you to take a value and format it in a specific way. For example, if you want to format a date or a number, you can use the date
or number
pipe.
date = new Date();
formattedDate = this.date | date: 'short';
// formattedDate = '9/25/2020'
number = 12345.67;
formattedNumber = this.number | number: '2.2-2';
// formattedNumber = '12,345.67'
Custom Pipes
In addition to the built-in pipes, Angular also allows you to create your own custom pipes. This is a great way to extend the functionality of your app and make it even more powerful. To create a custom pipe, you need to create a new class that implements the PipeTransform
interface. Then you can use the @Pipe
decorator to register your pipe with Angular.
@Pipe({
name: 'myPipe'
})
export class MyPipe implements PipeTransform {
transform(value: any, args?: any): any {
// Your code here
}
}
By using Angular pipes, you can quickly and easily transform data within your application. Whether you’re using built-in or custom pipes, they can help you keep your code organized and make your application more dynamic. So don’t wait: start supercharging your Angular app with powerful pipes today!
Recent Posts
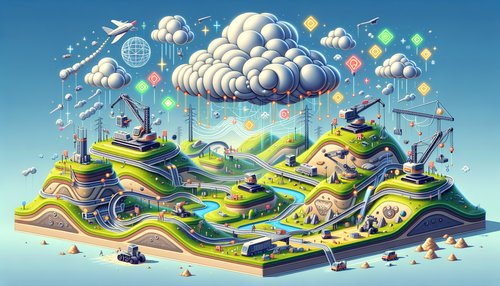
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
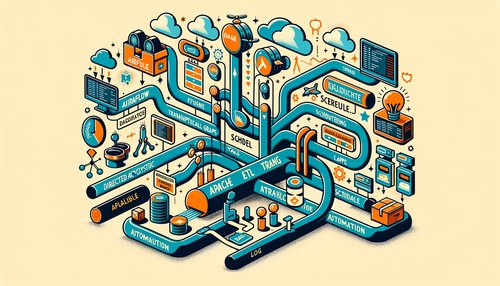
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow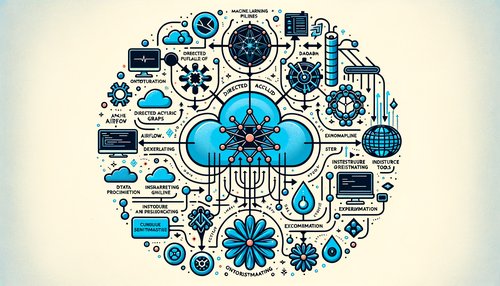
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow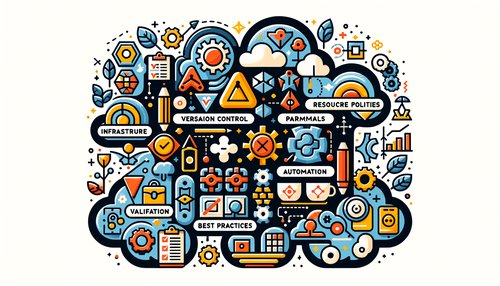
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
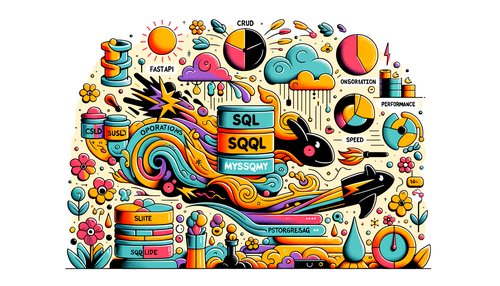