The Differences between Class Based and Function Based Views in the Django Framework
Django is a powerful web framework that allows developers to quickly create web applications. One of the key features of Django is its ability to provide developers with two different types of views: class-based views and function-based views. Both of these views have their own advantages and disadvantages, and it is important to understand the differences between them in order to make an informed decision when developing an application.
Class Based Views
Class-based views are a type of view that is defined as a Python class. They are typically used when a view needs to be more complex than a simple function. Class-based views provide developers with a convenient way to organize code and make it easier to reuse. They also allow developers to override certain methods to customize the behavior of the view.
An example of a class-based view in Django might look like this:
from django.views.generic import View
class MyView(View):
def get(self, request):
# Do something with the request
return Response(...)
This example shows a basic class-based view that responds to GET requests. The code inside the get
method is executed when a GET request is sent to the view.
Function Based Views
Function-based views are a type of view that is defined as a Python function. They are typically used for simpler views that do not require the complexity of a class-based view. Function-based views provide developers with a convenient way to quickly create simple views without having to write a lot of code.
An example of a function-based view in Django might look like this:
def my_view(request):
# Do something with the request
return Response(...)
This example shows a basic function-based view that responds to requests. The code inside the function is executed when a request is sent to the view.
Conclusion
Class-based views and function-based views are two different types of views that can be used in Django. Class-based views are useful when a view needs to be more complex and require more customization, while function-based views are useful for quickly creating simple views. It is important to understand the differences between the two in order to make an informed decision when developing an application.
Recent Posts
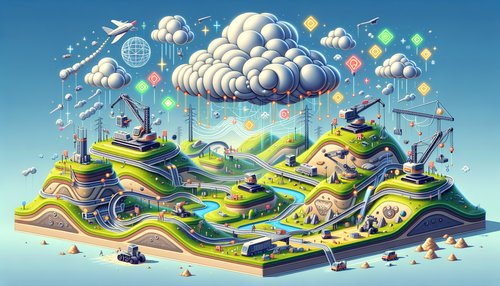
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
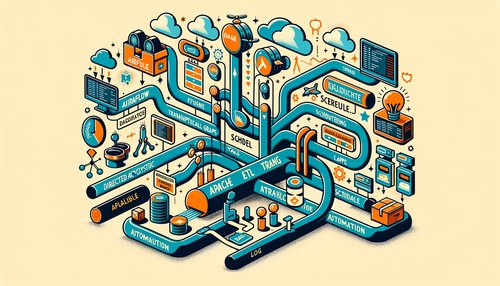
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow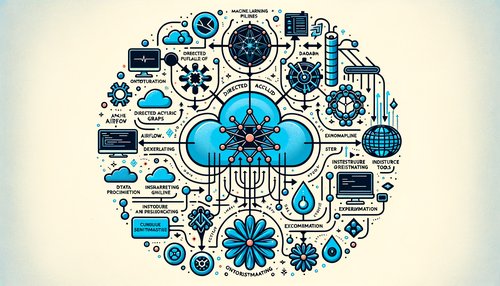
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow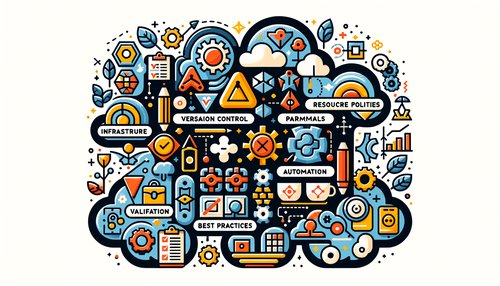
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
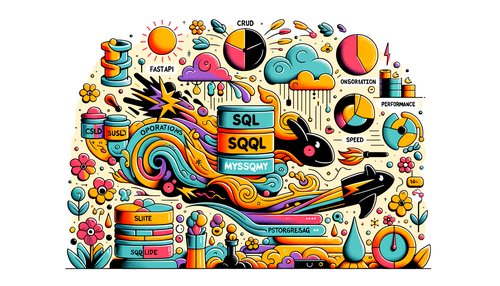