Unlocking the Full Potential of FastAPI: A Comprehensive Guide to Managing Static Files Efficiently
In the rapidly evolving world of web development, FastAPI has emerged as a game-changer for developers seeking performance and simplicity. However, efficiently managing static files—such as HTML, CSS, JavaScript, images, and other resources—can sometimes pose a challenge. This guide aims to provide you with the best practices and insights to streamline static file management in your FastAPI projects.
The Importance of Static File Management
Static files are the backbone of modern web applications, influencing both user experience and site performance. Proper handling of these resources is crucial for reducing server load and ensuring fast response times. By mastering static file management in FastAPI, you can optimize your projects for better performance and scalability.
Serving Static Files in FastAPI
FastAPI does not natively support serving static files. However, you can leverage Starlette’s capabilities—upon which FastAPI is built—to serve static files efficiently. Here’s a quick look at how you can get started:
from fastapi import FastAPI
from fastapi.staticfiles import StaticFiles
app = FastAPI()
app.mount("/static", StaticFiles(directory="static"), name="static")
In this example, static files are served from the static
directory, which is mounted at the /static
endpoint. This allows clients to access files using URLs like /static/filename
.
Optimizing Static Content Delivery
To maximize efficiency, it’s important to minimize the size and load time of your static content. Techniques such as compression, caching, and Content Delivery Networks (CDNs) can significantly enhance performance:
- Compression: Use tools like gzip or brotli to compress your static files. This reduces their size and speeds up download times.
- Caching: Set appropriate cache headers to enable browsers to store static files locally, reducing server requests.
- CDNs: Offload the serving of static files to a Content Delivery Network, which can provide your files from the nearest server location to the client.
Automation and Deployment Considerations
Automating the management of static files can save time and reduce errors. Consider setting up build processes with tools such as Webpack or Gulp to optimize and move files automatically before deployment. Additionally, deploying your FastAPI application to a cloud provider often involves configuring file storage and delivery mechanisms to ensure seamless access to static files across regions.
Practical Tips for Managing Static Files
Here are a few practical tips that can help you manage static files more effectively:
- Organize your static files logically in separate folders (e.g.,
images
,scripts
,styles
) for easier maintenance. - Take advantage of hashing and versioning to manage updates in static files without cache issues.
- Regularly audit your static files to remove unused ones, keeping your project lightweight and efficient.
Conclusion
Managing static files efficiently in FastAPI projects is an essential skill for any developer aiming to build performant web applications. By understanding and implementing the strategies outlined in this guide, you can ensure that your static content is delivered quickly and reliably to users around the globe. Start optimizing your static file management today, and watch your FastAPI applications perform like never before!
Recent Posts
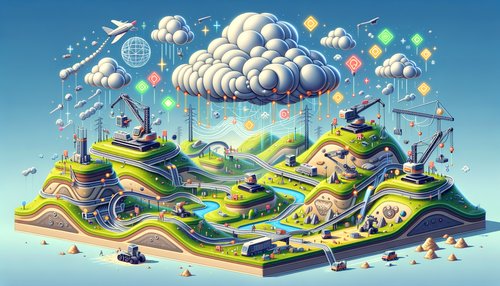
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
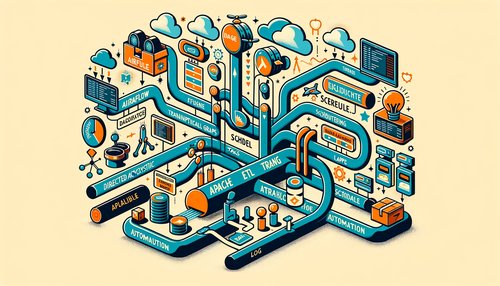
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow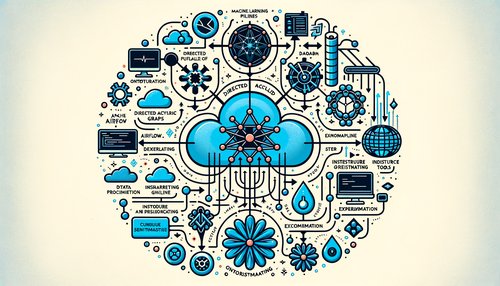
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow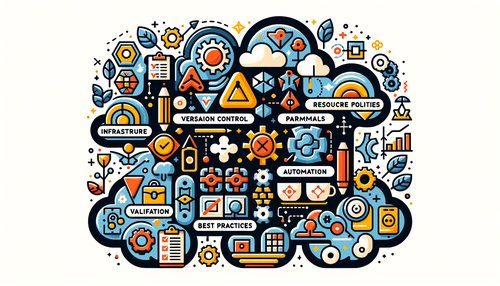
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
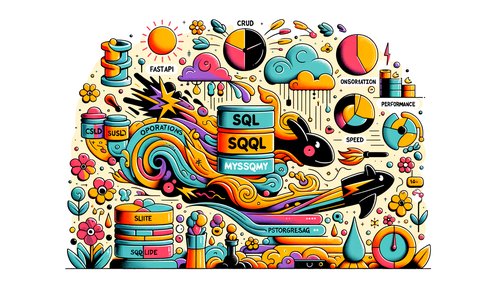