How Django Signals Can Help Streamline Your App Development Process
Developing an app can be a time-consuming and laborious process. From setting up the initial framework to creating the necessary models and views, there are many steps involved. Fortunately, Django, a popular Python web framework, has a powerful feature called signals that can help streamline your app development process.
Django signals are a way of sending notifications when certain actions occur. For example, if you want to be notified when a user registers on your app, you can set up a signal to send an email to the user. Signals can also be used to update records in the database, send emails, and more.
In addition to simplifying development, Django signals also make your code more efficient. By using signals, you can avoid writing code that performs the same task multiple times. Instead, you can define a signal once and have it run whenever the specified action occurs. This saves time and reduces the risk of errors.
Using Django signals is relatively straightforward. To set up a signal, you first need to define a receiver function. This is the code that will run when the signal is triggered. You can then connect the receiver to a signal using the @receiver
decorator.
For example, let's say you want to create a signal that sends an email to a user when they register on your app. First, you would define the receiver function:
@receiver(user_registered)
def send_registration_email(sender, **kwargs):
# code to send an email
Next, you would connect the receiver to the user_registered
signal:
user_registered.connect(send_registration_email)
Once the signal is set up, it will run every time a new user registers on your app. This eliminates the need to write code to send the registration email each time a user registers.
As you can see, Django signals can be a powerful tool for streamlining your app development process. They allow you to define functionality once and have it run automatically whenever a specified action occurs. This saves time and reduces the risk of errors, making it easier to develop high-quality apps.
Recent Posts
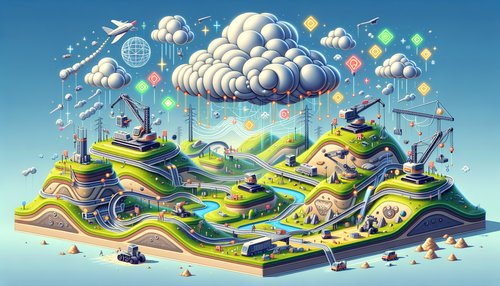
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
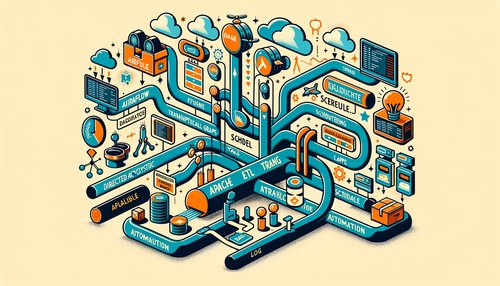
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow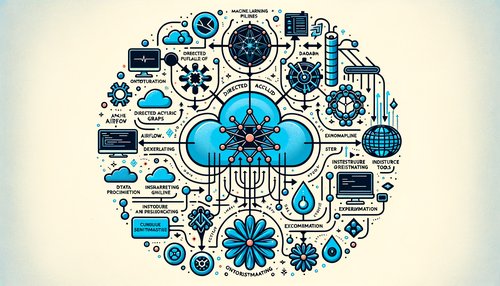
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow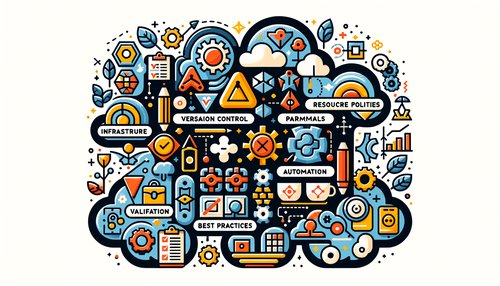
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
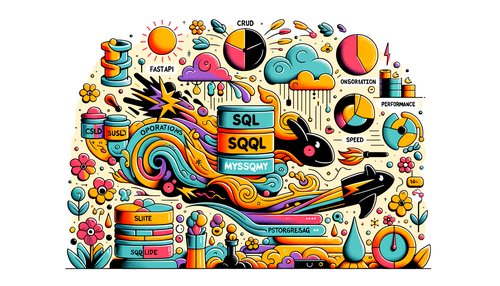