Mastering FastAPI: An In-Depth User Guide to Efficient Testing
If you're a developer looking to build highly scalable and high-performance web APIs, you've likely come across FastAPI. Known for its speed and ease of use, FastAPI is a modern web framework for Python. But building a robust API is just half the battle; the other half is ensuring it remains reliable, smooth, and bug-free through efficient testing. In this comprehensive guide, we'll walk you through everything you need to know about testing FastAPI applications effectively.
Why Testing is Crucial for FastAPI Applications
Before diving into the nitty-gritty details, it’s important to understand why testing is crucial. Testing ensures that your application functions correctly and consistently as expected. It helps in identifying bugs early, facilitates easier code maintenance, and boosts confidence in deployment.
Setting Up Your Testing Environment
The first step to effective testing is setting up your environment. You’ll need to ensure you have FastAPI installed along with a testing framework like Pytest. Here's a quick setup guide:
pip install fastapi
pip install uvicorn
pip install pytest
pip install httpx
Writing Your First Test
To get started, create a new Python file named test_app.py
. This file will contain your test cases. Import the necessary modules:
from fastapi.testclient import TestClient
from myapp import app # replace 'myapp' with the name of your FastAPI app file
Create a TestClient instance:
client = TestClient(app)
Now, write your first test function:
def test_read_main():
response = client.get("/")
assert response.status_code == 200
assert response.json() == {"message": "Hello World"}
This simple test makes a GET request to the root endpoint and checks if the response status is 200 and matches the expected JSON output.
Advanced Testing Techniques
Once you’ve mastered basic testing, it's time to dive into more advanced techniques like testing with database dependencies, handling authentication, and testing error responses.
Testing Endpoints with Database Dependencies
Use the TestingSessionLocal
fixture to handle database sessions in your tests without disturbing your actual database:
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
from myapp.database import Base, get_db
SQLALCHEMY_DATABASE_URL = "sqlite:///./test.db"
engine = create_engine(SQLALCHEMY_DATABASE_URL)
TestingSessionLocal = sessionmaker(autocommit=False, autoflush=False, bind=engine)
Base.metadata.create_all(bind=engine)
def override_get_db():
try:
db = TestingSessionLocal()
yield db
finally:
db.close()
app.dependency_overrides[get_db] = override_get_db
Use the overridden dependency in your test cases:
def test_create_item():
response = client.post("/items/", json={"name": "Test Item"})
assert response.status_code == 200
data = response.json()
assert data["name"] == "Test Item"
Handling Authentication
For endpoints requiring authentication, mock the authentication headers:
def test_auth_endpoint():
response = client.get("/secure-endpoint/", headers={"Authorization": "Bearer testtoken"})
assert response.status_code == 200
Testing Error Responses
Ensure your application handles errors gracefully:
def test_404_error():
response = client.get("/nonexistent")
assert response.status_code == 404
assert response.json() == {"detail": "Not Found"}
Practical Tips for Efficient Testing
Here are some practical tips to make your testing process more efficient:
- Use fixtures to set up and tear down test conditions.
- Take advantage of Pytest's parameterized tests to cover multiple scenarios with less code.
- Keep tests isolated to ensure they do not depend on each other.
Conclusion
Testing is an essential aspect of software development that ensures your FastAPI applications remain reliable and scalable. By setting up a robust testing environment, writing simple and advanced tests, and following best practices, you can significantly improve the quality of your applications. Happy testing!
If you found this guide helpful, don’t forget to share it with your peers and stay tuned for more insights into mastering FastAPI.
Recent Posts
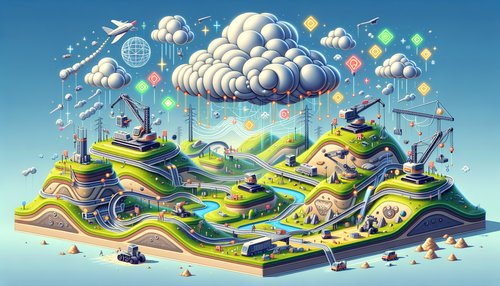
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
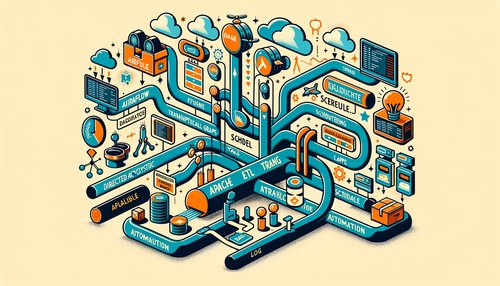
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow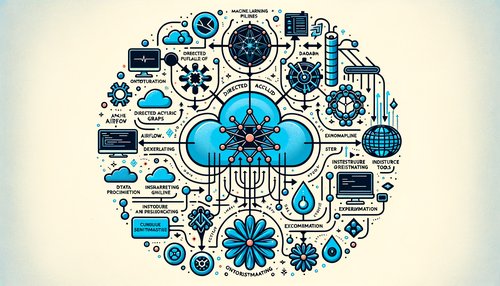
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow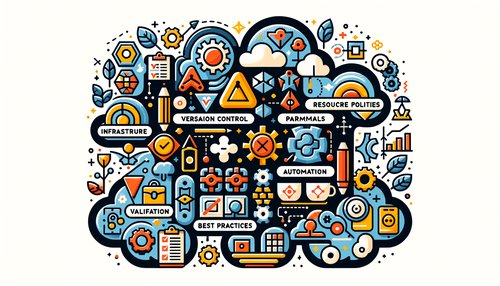
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
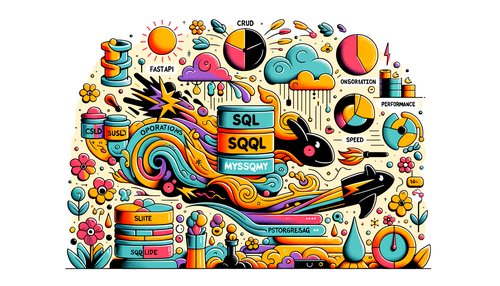