Unlock the Power of Angular Modules: A Guide to Simplifying Your App Development
Angular Modules are a powerful tool for simplifying and organizing your front-end development workflow. By breaking down your application into smaller, manageable pieces, Angular Modules allow you to easily reuse components and keep your codebase consistent and organized.
In this guide, we’ll cover the basics of Angular Modules and how to use them to simplify your app development.
What are Angular Modules?
Angular Modules are a way to divide your code into smaller, more manageable pieces. They are the building blocks of an Angular application and are used to group related components, services, and directives.
Each module can be imported into other modules, which allows you to easily reuse components and keep your codebase consistent and organized.
How to Use Angular Modules
To use Angular Modules, you first need to create a new module. You can do this by using the ng generate
command in your terminal:
ng generate module <module-name>
Once you have created your module, you can import it into other modules using the import
keyword:
import { <module-name> } from './<module-name>';
Once imported, you can use the components, services, and directives of your module in other modules.
Benefits of Using Angular Modules
Using Angular Modules has a number of benefits, including:
-
Organization: Modules help you keep your codebase organized by breaking it down into manageable pieces.
-
Reusability: By importing modules into other modules, you can easily reuse components and keep your codebase consistent.
-
Ease of Maintenance: Modules help you keep your codebase organized, which makes it easier to debug and maintain.
Conclusion
Angular Modules are a powerful tool for simplifying and organizing your front-end development workflow. By breaking down your application into smaller, manageable pieces, Angular Modules allow you to easily reuse components and keep your codebase consistent and organized.
If you’re looking to simplify your app development, Angular Modules are a great way to get started.
Recent Posts
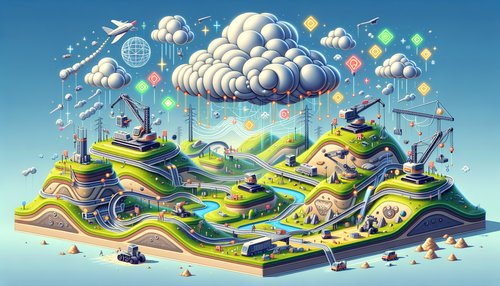
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
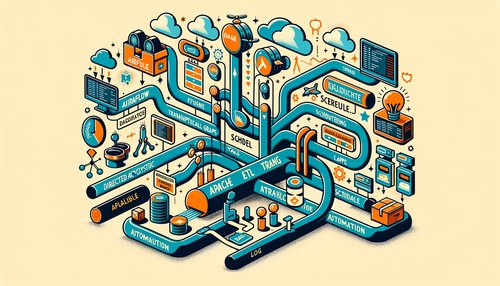
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow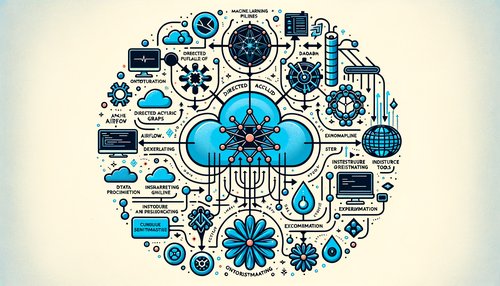
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow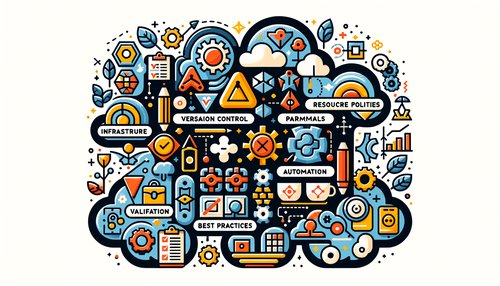
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
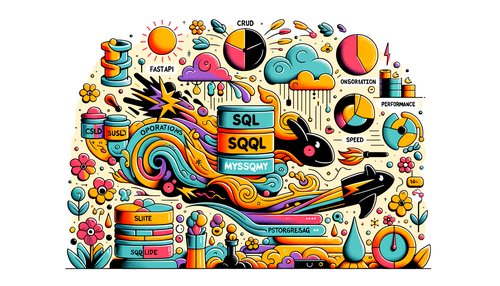