Unlock the Power of React with These Useful Hooks
React is a powerful JavaScript library for building user interfaces that can increase the speed and efficiency of your development process. Its component-based structure makes it easy to create complex, interactive applications. React also offers a range of hooks, which are functions that allow you to access React’s features and state in a more direct way. In this blog post, we’ll take a look at some of the most useful hooks and how they can help you unlock the power of React.
useState
The useState hook is one of the most commonly used hooks in React. It allows you to use state in a functional component, which is a component that does not have a class. The useState hook takes a single argument, which is the initial state, and returns an array with two elements. The first element is the current state, and the second element is a function that allows you to update the state.
For example, if you wanted to create a counter component, you could use the useState hook like this:
const [count, setCount] = useState(0);
This code creates a state variable called count and assigns it an initial value of 0. The setCount function can then be used to update the state. For example, if you wanted to increment the count, you could do this:
setCount(count + 1);
useEffect
The useEffect hook allows you to perform side effects in a functional component. It is similar to the componentDidMount and componentDidUpdate lifecycle methods in class components. The useEffect hook takes a callback function as its first argument and an optional array of values as its second argument. The callback function is called after every render, and the values in the array are used to determine when the callback should be called.
For example, if you wanted to log a message to the console after every render, you could do this:
useEffect(() => {
console.log('Hello!');
});
useContext
The useContext hook allows you to access the context in a functional component. Context is used to pass data through the component tree without having to pass props down manually. The useContext hook takes a context object as its argument and returns the current context value.
For example, if you wanted to access the current user in a component, you could do this:
const user = useContext(UserContext);
Conclusion
React hooks are a powerful tool for unlocking the power of React. They allow you to access React’s features and state in a more direct way, which can make your development process more efficient. In this blog post, we’ve looked at some of the most useful hooks and how they can help you unlock the power of React.
Recent Posts
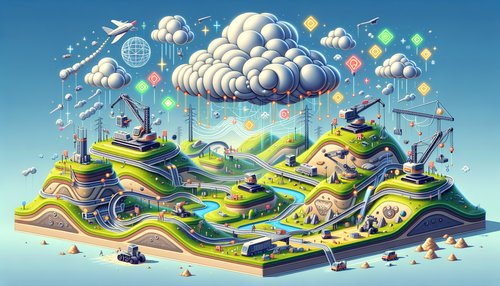
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
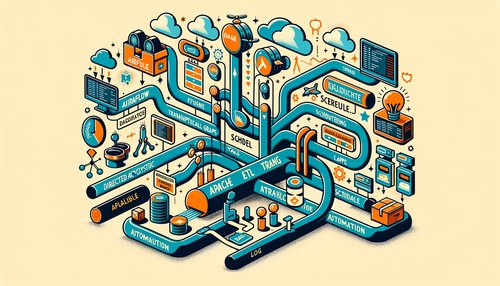
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow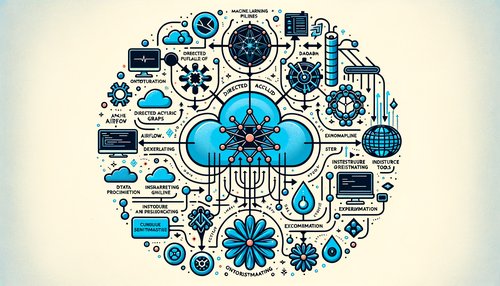
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow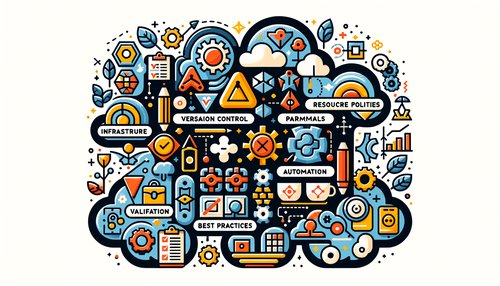
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
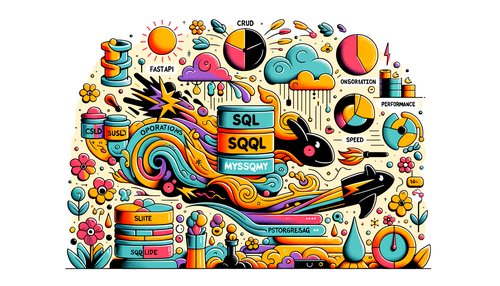