Organizing React Components for Maximum Reusability and Flexibility
React is a powerful JavaScript library for building user interfaces. It allows developers to create components that can be reused and customized for different projects. However, organizing components in a way that maximizes reusability and flexibility can be a challenge. In this blog post, we'll explore some best practices for organizing React components for maximum reusability and flexibility.
1. Create a Component Library
The first step in organizing React components for maximum reusability and flexibility is to create a component library. This is a collection of components that can be used in different projects. Component libraries should be organized in a way that makes it easy to find and use components. For example, you may want to group components by type (e.g. buttons, forms, etc.) or by purpose (e.g. navigation, user profile, etc.).
2. Use Props for Customization
Props are a powerful way to customize components. You can use props to pass data from parent components to child components, or to customize the look and feel of a component. For example, the following code snippet shows how to use props to customize a button component:
<Button
text="Click Me!"
color="blue"
onClick={handleClick}
/>
In this example, the button component is being customized with the text, color, and onClick props.
3. Use State for Dynamic Components
State is another powerful tool for creating dynamic components. State is used to store information about a component that can change over time. For example, the following code snippet shows how to use state to create a dynamic list component:
<List
items={items}
onItemClick={handleItemClick}
/>
In this example, the list component is being populated with an array of items from the state. The onItemClick prop is used to handle user interactions with the list.
4. Separate Presentational and Container Components
Finally, it's important to separate presentational components from container components. Presentational components are responsible for displaying data, while container components handle the logic and state. Separating presentational and container components helps to keep components organized and makes them easier to reuse and modify.
Conclusion
Organizing React components for maximum reusability and flexibility is an important part of building user interfaces. By creating a component library, using props and state for customization, and separating presentational and container components, you can ensure that your components are organized in a way that maximizes reusability and flexibility.
Recent Posts
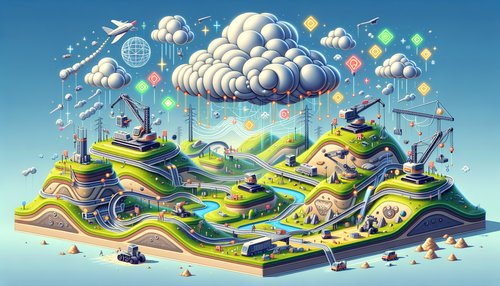
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
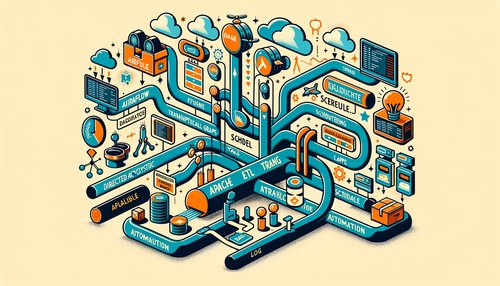
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow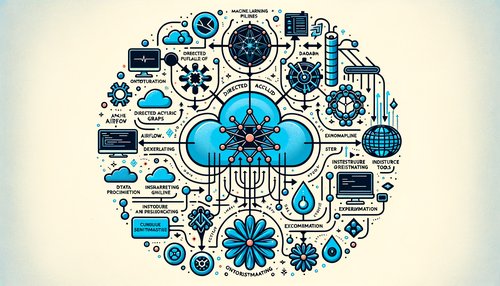
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow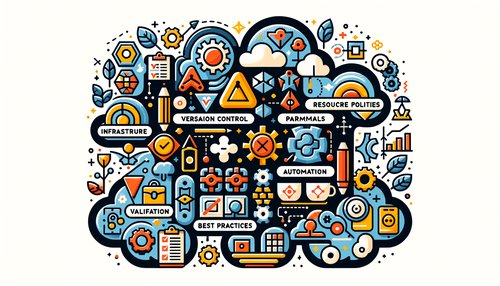
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
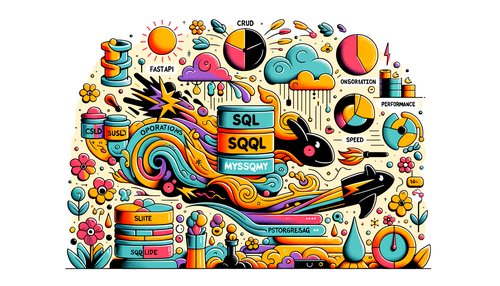